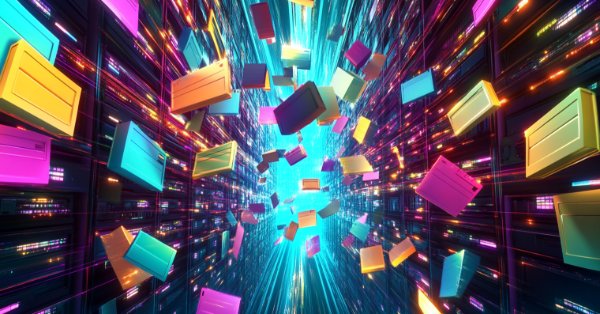
Mastering File Operations in MQL5: From Basic I/O to Building a Custom CSV Reader
Introduction
In today’s automated trading world, data is everything. Maybe you need to load custom parameters for your strategy, read a watchlist of symbols, or integrate historical data from outside sources. If you’re working in MetaTrader 5, you’ll be glad to know MQL5 makes it pretty straightforward to handle files right from your code.
But let’s be honest: digging through the documentation to figure out file operations can feel a bit overwhelming at first. That’s why in this article, we’ll break down the fundamentals in a friendly, step-by-step manner. Once we cover the basics – like how MQL5’s “sandbox” protects your files, how to open files in text or binary mode, and how to safely read and split lines – we’ll put it all into practice by building a simple CSV reader class.
Why CSV files? Because they’re everywhere – simple, human-readable, and supported by countless tools. With a CSV reader, you can import external parameters, symbol lists, or other custom data right into your Expert Advisor or script, adjusting your strategy’s behavior without changing the code every time.
We won’t drown you in every tiny detail of MQL5 file functions, but we will cover what you need to know. By the end, you’ll have a clear example of how to open a CSV file in text mode, how to read its lines until the end of the file, how to split each line into fields by a chosen delimiter, how to store and retrieve these fields by column name or index and clear understanding for each of them.
Here's the plan for this article:
- Fundamentals of MQL5 File Operations
- Designing the CSV Reader Class
- Finalizing the CSV Reader Class Implementation
- Testing & Usage Scenarios
- Conclusion
Fundamentals of MQL5 File Operations
Before we implement our CSV reader, let’s take a closer look at some core file-handling concepts in MQL5 and illustrate them with code. We’ll focus on understanding the sandbox restrictions, file opening modes, line-by-line reading, and basic error handling. Seeing these fundamentals in action will make it easier to build and debug our CSV reader later on.
First, let's understand The Sandbox, Restricted File Access. MQL5 enforces a security model that restricts file operations to certain directories known as the “sandbox.” Typically, you can only read and write files located in <TerminalDataFolder>/MQL5/Files. If you attempt to access files outside this directory, the FileOpen() function will fail.
For example, if you place a file named data.csv in the MQL5/Files folder of your MT5 terminal, you can open it like this:
int fileHandle = FileOpen("data.csv", FILE_READ|FILE_TXT); if(fileHandle == INVALID_HANDLE) { Print("Error: Could not open data.csv. LastError=", _LastError); // _LastError can help diagnose if it's a path or permission issue return; } // Successfully opened the file, now we can read from it.
You might wonder what those error codes mean. For instance, _LastError = 5004 typically means something like “File not found” or “Can’t open the file,” which often comes down to a typo in the filename or the file not being inside MQL5/Files. If you see another code, a quick check in the MQL5 documentation or community forums can help you decode the message. Sometimes it’s just a path issue, sometimes the file is locked by another program. If external data is crucial to your EA, consider adding a quick retry or a detailed error printout so you can fix problems fast.
We have many options when opening a file. When you call FileOpen(), you specify flags to control how the file is accessed. Common flags include:
- FILE_READ : Open the file for reading.
- FILE_WRITE : Open for writing.
- FILE_BIN : Binary mode (no text processing).
- FILE_TXT : Text mode (handles line endings and text conversions).
- FILE_CSV : Special text mode that treats the file as a CSV.
For reading a standard CSV, FILE_READ|FILE_TXT is a great starting point. Text mode ensures that FileReadString() will stop at newlines, making it simpler to process files line-by-line:
int handle = FileOpen("params.txt", FILE_READ|FILE_TXT); if(handle != INVALID_HANDLE) { Print("File opened in text mode."); // ... read lines here ... FileClose(handle); } else { Print("Failed to open params.txt"); }
Once the file is open in text mode, reading lines is straightforward. Use FileReadString() to read until the next newline. When the file ends, FileIsEnding() returns true. Consider this loop:
int handle = FileOpen("list.txt", FILE_READ|FILE_TXT); if(handle == INVALID_HANDLE) { Print("Error opening list.txt"); return; } while(!FileIsEnding(handle)) { string line = FileReadString(handle); if(line == "" && _LastError != 0) { // If empty line and there's an error, break Print("Read error or unexpected end of file. _LastError=", _LastError); break; } // Process the line Print("Line read: ", line); } FileClose(handle);
In this snippet, we continuously read lines until we reach the end of the file. If an error occurs, we stop. Empty lines are allowed, so if you want to skip them, just check if(line=="") continue; . This approach will be handy when handling CSV rows.
Keep in mind that text files aren’t always uniform. Most use \n or \r\n for line endings, and MQL5 usually handles these cleanly. Still, if you get a file from an unusual source, it’s worth checking if lines read correctly. If FileReadString() returns odd results (like merged lines), open the file in a text editor and confirm its encoding and newline style. Also, consider extremely long lines – rare for small CSVs, but possible. A length check or trimming can help ensure your EA doesn’t stumble over unexpected formats.
To process CSV data, you’ll split each line into fields based on a delimiter, often a comma or semicolon. MQL5’s StringSplit() function helps:
string line = "EURUSD;1.2345;Some Comment"; string fields[]; int count = StringSplit(line, ';', fields); if(count > 0) { Print("Found ", count, " fields"); for(int i=0; i<count; i++) Print("Field[", i, "] = ", fields[i]); } else { Print("No fields found in line: ", line); }
This code prints each parsed field. For CSV reading, after splitting, you’ll store these fields in memory so you can access them later by column index or name.
While StringSplit() works great for simple delimiters, remember that CSV formats can get tricky. Some have quoted fields or escaped delimiters, which we’re not handling here. If your file is straightforward – no quotes or fancy tricks – StringSplit() is enough. If fields have trailing spaces or odd punctuation, consider using StringTrim() after splitting. Such small checks keep your EA robust, even if your data source adds minor formatting quirks.
Many CSV files have a header row that defines column names. If _hasHeader is true in our upcoming CSV reader, the first line read will be split and stored in a hash map mapping column names to indices.
For example:
// Assume header line: "Symbol;MaxLot;MinSpread" string header = "Symbol;MaxLot;MinSpread"; string cols[]; int colCount = StringSplit(header, ';', cols); // Suppose we have a CHashMap<string,uint> Columns; for(int i=0; i<colCount; i++) Columns.Add(cols[i], i); // Now we can quickly find the index for "MinSpread" or any other column name. uint idx; bool found = Columns.TryGetValue("MinSpread", idx); if(found) Print("MinSpread column index: ", idx); else Print("Column 'MinSpread' not found");If no header is present, we’ll just rely on numerical indexes. The first line read will be a data row, and columns will be referenced by their positions.
The hash map ( CHashMap ) for column names is a small detail that makes a big difference. Without it, every time you needed a column index, you’d have to loop through the header fields. With a hash map, TryGetValue() gives you the index right away. If a column isn’t found, you can return an error value—simple and elegant. If you worry about columns that appear twice, you can add a quick check when reading the header and print a warning if duplicates occur. Little enhancements like that keep your code robust as your CSVs get more complex over time.
For data storage, we’ll keep it simple: each parsed line (after splitting) becomes a row. We’ll use CArrayString to hold fields of a single row and CArrayObj to store multiple rows:
#include <Arrays\ArrayObj.mqh> #include <Arrays\ArrayString.mqh> CArrayObj Rows; // after splitting line into fields: CArrayString *row = new CArrayString; for(int i=0; i<count; i++) row.Add(fields[i]); Rows.Add(row);
Later, to retrieve a value:
// Access row 0, column 1 CArrayString *aRow = Rows.At(0); string val = aRow.At(1); Print("Row0 Col1: ", val);
// Access row 0, column 1 CArrayString *aRow = Rows.At(0); string val = aRow.At(1); Print("Row0 Col1: ", val);
We must ensure the indexes are valid before accessing them.
Always handle the possibility of missing files or columns. For example, if FileOpen() returns INVALID_HANDLE, log an error and return. If a requested column name doesn’t exist, return a default value. Our final CSV reader class will encapsulate these checks so that your main EA code remains tidy.
To bring it all together with these basics – sandbox rules, file opening, reading lines, splitting fields, and storing results – we have all the building blocks we need. In the next sections, we’ll design and implement our CSV reader class step-by-step, using these concepts. By focusing on clarity and error handling now, we’ll make the subsequent implementation smoother and more reliable.
Designing the CSV Reader Class
Now that we’ve refreshed the fundamentals, let’s outline the structure of our CSV reader class and start implementing key parts. We’ll create a class named something like CSimpleCSVReader that allows you to:
- Open a specified CSV file in read-text mode.
- If requested, treat the first line as a header, store column names, and build a map from column names to indices.
- Read all subsequent lines into memory, each line split into an array of strings (one per column).
- Provide methods to query data by column index or name.
- Return default or error values if something’s missing.
We’ll do this step-by-step. First, let’s consider the data structures we’ll use internally:
- A CHashMap<string,uint> to store the column name -> index mapping when headers are present.
- A dynamic array of CArrayString* for rows, where each CArrayString is one row of fields.
- Some stored properties like _hasHeader , _filename , _separator , and maybe a _rowCount and _colCount.
Using CArrayObj and CArrayString isn’t just convenient – it helps you avoid low-level array resizing headaches. Native arrays are powerful but can get messy with complex datasets. With CArrayString , adding fields is easy, and CArrayObj lets you store a growing list of rows without hassle. Meanwhile, a hash map for column names avoids scanning the header line over and over. It’s a design that’s both simple and scalable, making life easier as your CSV grows or your data needs evolve.
Before coding the entire class, let’s write some building-block code snippets to illustrate how to open a file and read lines. Later, we’ll integrate these pieces into the final class code. Let's open a file:
int fileHandle = FileOpen("data.csv", FILE_READ|FILE_TXT);
if(fileHandle == INVALID_HANDLE)
{
Print("Error: Could not open file data.csv. Error code=", _LastError);
return;
}
// If we reach here, the file is open successfully.
This snippet tries to open data.csv from the MQL5/Files directory. If it fails, it prints an error and returns. The _LastError variable can provide insight into why the file failed to open. For example, 5004 means CANNOT_OPEN_FILE . Now let's read the file until the file ends:
string line; while(!FileIsEnding(fileHandle)) { line = FileReadString(fileHandle); if(line == "" && _LastError != 0) // If empty line and error occurred { Print("Error reading line. Possibly end of file or another issue. Error=", _LastError); break; } // Process the line here, e.g., split it into fields }
Here we loop until FileIsEnding() returns true. Each iteration reads a line into line. If we get an empty line and there’s an error, we stop. If it’s genuinely the end of the file, we’ll naturally exit the loop. Keep in mind, a completely empty line in the file would still result in an empty string, so you might want to handle that scenario depending on your CSV format.
Now, Suppose our CSV uses a semicolon ( ; ) as a delimiter. We can do:
string line = "Symbol;Price;Volume"; string fields[]; int fieldCount = StringSplit(line, ';', fields); if(fieldCount < 1) { Print("No fields found in line: ", line); } else { // fields now contains each piece of data for(int i=0; i<fieldCount; i++) Print("Field[", i, "] = ", fields[i]); }
StringSplit() returns the number of parts found. After this call, fields contains each token separated by ; . If the line was EURUSD;1.2345;10000 , fields[0] would be EURUSD , fields[1] would be 1.2345 , and fields[2] would be 10000 .
If _hasHeader is true, the first line we read is special. We’ll split it and store the column names in a CHashMap . For example:
#include <Generic\HashMap.mqh> CHashMap<string,uint> Columns; // columnName -> columnIndex // Assume line is the header line string columns[]; int columnCount = StringSplit(line, ';', columns); for(int i=0; i<columnCount; i++) Columns.Add(columns[i], i);
The hash map for column names is a small detail that pays big dividends. Without it, you’d loop through column headers each time you wanted an index. With a hash map, a quick TryGetValue() call gives you the index, and if a column isn’t found, you can just return a default value. If duplicates or weird column names appear, you can detect them upfront. This setup keeps lookups fast and code clean, so even if your CSV doubles in size, retrieving column indexes stays simple.
Now Columns maps each column name to its index. If we later need the index for a given column name, we can do:
uint idx; bool found = Columns.TryGetValue("Volume", idx); if(found) Print("Volume column index = ", idx); else Print("Column 'Volume' not found");
Each data row should be stored in a CArrayString object, and we’ll keep a dynamic array of pointers to these rows. Something like:
#include <Arrays\ArrayString.mqh> #include <Arrays\ArrayObj.mqh> CArrayObj Rows; // holds pointers to CArrayString objects // After reading and splitting a line into fields: // (Assume fields[] array is populated) CArrayString *row = new CArrayString; for(int i=0; i<ArraySize(fields); i++) row.Add(fields[i]); Rows.Add(row);
Later, to retrieve a value, we’d do something like:
CArrayString *aRow = Rows.At(0); // get the first row string value = aRow.At(1); // get second column Print("Value at row=0, col=1: ", value);
Of course, we must always check boundaries to avoid out-of-range errors.
Let's access columns by name or index, If our CSV has a header, we can use the Columns map to find column indexes by name:
string GetValueByName(uint rowNumber, string colName, string errorValue="") { uint idx; if(!Columns.TryGetValue(colName, idx)) return errorValue; // column not found return GetValueByIndex(rowNumber, idx, errorValue); } string GetValueByIndex(uint rowNumber, uint colIndex, string errorValue="") { if(rowNumber >= Rows.Total()) return errorValue; // invalid row CArrayString *aRow = Rows.At(rowNumber); if(colIndex >= (uint)aRow.Total()) return errorValue; // invalid column index return aRow.At(colIndex); }
This pseudo-code shows how we might implement two accessor functions. GetValueByName uses the hash map to convert the column name into an index, then calls GetValueByIndex . GetValueByIndex checks boundaries and returns values or error defaults as needed.
Constructor and Destructor: We can wrap everything into a class. The constructor might just initialize internal variables, and the destructor should free memory. For example:
class CSimpleCSVReader { private: bool _hasHeader; string _separator; CHashMap<string,uint> Columns; CArrayObj Rows; public: CSimpleCSVReader() { _hasHeader = true; _separator=";"; } ~CSimpleCSVReader() { Clear(); } void SetHasHeader(bool hasHeader) { _hasHeader = hasHeader; } void SetSeparator(string sep) { _separator = sep; } uint Load(string filename); string GetValueByName(uint rowNum, string colName, string errorVal=""); string GetValueByIndex(uint rowNum, uint colIndex, string errorVal=""); private: void Clear() { for(int i=0; i<Rows.Total(); i++) { CArrayString *row = Rows.At(i); if(row != NULL) delete row; } Rows.Clear(); Columns.Clear(); } };
This sketch of a class shows a possible structure. We haven’t implemented Load() yet, but we will soon. Notice how we keep a Clear() method to release memory. After calling delete row; , we must also Rows.Clear() to reset the array of pointers.
Let's implement the load method now. Load() will open the file, read the first line (possibly header), read all remaining lines, and parse them:
uint CSimpleCSVReader::Load(string filename) { // Clear any previous data Clear(); int fileHandle = FileOpen(filename, FILE_READ|FILE_TXT); if(fileHandle == INVALID_HANDLE) { Print("Error opening file: ", filename, " err=", _LastError); return 0; } if(_hasHeader) { // read first line as header if(!FileIsEnding(fileHandle)) { string headerLine = FileReadString(fileHandle); string headerFields[]; int colCount = StringSplit(headerLine, StringGetCharacter(_separator,0), headerFields); for(int i=0; i<colCount; i++) Columns.Add(headerFields[i], i); } } uint rowCount=0; while(!FileIsEnding(fileHandle)) { string line = FileReadString(fileHandle); if(line == "") continue; // skip empty lines string fields[]; int fieldCount = StringSplit(line, StringGetCharacter(_separator,0), fields); if(fieldCount<1) continue; // no data? CArrayString *row = new CArrayString; for(int i=0; i<fieldCount; i++) row.Add(fields[i]); Rows.Add(row); rowCount++; } FileClose(fileHandle); return rowCount; }
This Load() function:
- Clears old data.
- Opens the file.
- If _hasHeader is true, reads the first line as header and fills Columns .
- Then reads lines until the file ends, splitting them into fields.
- For each line, creates a CArrayString , populates it, and adds it to Rows .
- Returns the number of data rows read.
To bring it all together, We now have a good portion of the logic outlined. In the next sections, we’ll refine and finalize the code, add the missing accessor methods, and show the final full code listing. We’ll also demonstrate usage examples, like how to check how many rows you got, what columns exist, and how to fetch values safely.
By walking through these code snippets, you can see how the logic pieces fit together. The final CSV reader class will be self-contained and easy to integrate: just create an instance, call Load("myfile.csv"), then use GetValueByName() or GetValueByIndex() to retrieve the information you need.
In the next section, we’ll complete the entire class implementation and show a final code snippet ready for you to copy and adapt. After that, we’ll wrap up with some usage examples and concluding remarks.
Finalizing the CSV Reader Class Implementation
In the previous sections, we outlined the structure of our CSV reader and worked through various parts of the code. Now it’s time to put it all together into a single, cohesive implementation. Afterward, we’ll briefly show how to use it. In the final article structure, we’ll present the entire code at once here, so you have a clear reference.
We’ll integrate the helper functions we discussed – loading files, parsing headers, storing rows, and accessor methods – into a single MQL5 class. Then we’ll show a short snippet demonstrating how you might use the class in your EA or script. Recall that this class:
- Reads a CSV from the MQL5/Files directory.
- If _hasHeader is true, it extracts column names from the first line.
- Subsequent lines form rows of data stored in CArrayString.
- You can retrieve values by column name (if a header exists) or by column index.
We’ll also include some error checking and defaults. Let’s present the full code now. Please note that this code is an illustrative example and might require minor tweaks depending on your environment. We assume that files HashMap.mqh, ArrayString.mqh, and ArrayObj.mqh are available from the standard MQL5 include directories.
Here's the Full Code Listing of the CSV Reader:
//+------------------------------------------------------------------+ //| CSimpleCSVReader.mqh | //| A simple CSV reader class in MQL5. | //| Assumes CSV file is located in MQL5/Files. | //| By default, uses ';' as the separator and treats first line as | //| header. If no header, columns are accessed by index only. | //+------------------------------------------------------------------+ #include <Generic\HashMap.mqh> #include <Arrays\ArrayObj.mqh> #include <Arrays\ArrayString.mqh> class CSimpleCSVReader { private: bool _hasHeader; string _separator; CHashMap<string,uint> Columns; CArrayObj Rows; // Array of CArrayString*, each representing a data row public: CSimpleCSVReader() { _hasHeader = true; _separator = ";"; } ~CSimpleCSVReader() { Clear(); } void SetHasHeader(bool hasHeader) {_hasHeader = hasHeader;} void SetSeparator(string sep) {_separator = sep;} // Load: Reads the file, returns number of data rows. uint Load(string filename); // GetValue by name or index: returns specified cell value or errorVal if not found string GetValueByName(uint rowNum, string colName, string errorVal=""); string GetValueByIndex(uint rowNum, uint colIndex, string errorVal=""); // Returns the number of data rows (excluding header) uint RowCount() {return Rows.Total();} // Returns the number of columns. If no header, returns column count of first data row uint ColumnCount() { if(Columns.Count() > 0) return Columns.Count(); // If no header, guess column count from first row if available if(Rows.Total()>0) { CArrayString *r = Rows.At(0); return (uint)r.Total(); } return 0; } // Get column name by index if header exists, otherwise return empty or errorVal string GetColumnName(uint colIndex, string errorVal="") { if(Columns.Count()==0) return errorVal; // Extract keys and values from Columns string keys[]; int vals[]; Columns.CopyTo(keys, vals); if(colIndex < (uint)ArraySize(keys)) return keys[colIndex]; return errorVal; } private: void Clear() { for(int i=0; i<Rows.Total(); i++) { CArrayString *row = Rows.At(i); if(row != NULL) delete row; } Rows.Clear(); Columns.Clear(); } }; //+------------------------------------------------------------------+ //| Implementation of Load() method | //+------------------------------------------------------------------+ uint CSimpleCSVReader::Load(string filename) { Clear(); // Start fresh int fileHandle = FileOpen(filename, FILE_READ|FILE_TXT); if(fileHandle == INVALID_HANDLE) { Print("CSVReader: Error opening file: ", filename, " err=", _LastError); return 0; } uint rowCount=0; // If hasHeader, read first line as header if(_hasHeader && !FileIsEnding(fileHandle)) { string headerLine = FileReadString(fileHandle); if(headerLine != "") { string headerFields[]; int colCount = StringSplit(headerLine, StringGetCharacter(_separator,0), headerFields); for(int i=0; i<colCount; i++) Columns.Add(headerFields[i], i); } } while(!FileIsEnding(fileHandle)) { string line = FileReadString(fileHandle); if(line == "") continue; // skip empty lines string fields[]; int fieldCount = StringSplit(line, StringGetCharacter(_separator,0), fields); if(fieldCount<1) continue; // no data? CArrayString *row = new CArrayString; for(int i=0; i<fieldCount; i++) row.Add(fields[i]); Rows.Add(row); rowCount++; } FileClose(fileHandle); return rowCount; } //+------------------------------------------------------------------+ //| GetValueByIndex Method | //+------------------------------------------------------------------+ string CSimpleCSVReader::GetValueByIndex(uint rowNum, uint colIndex, string errorVal="") { if(rowNum >= Rows.Total()) return errorVal; CArrayString *aRow = Rows.At(rowNum); if(aRow == NULL) return errorVal; if(colIndex >= (uint)aRow.Total()) return errorVal; string val = aRow.At(colIndex); return val; } //+------------------------------------------------------------------+ //| GetValueByName Method | //+------------------------------------------------------------------+ string CSimpleCSVReader::GetValueByName(uint rowNum, string colName, string errorVal="") { if(Columns.Count() == 0) { // No header, can't lookup by name return errorVal; } uint idx; bool found = Columns.TryGetValue(colName, idx); if(!found) return errorVal; return GetValueByIndex(rowNum, idx, errorVal); } //+------------------------------------------------------------------+
Let’s take a closer look at Load() . It clears old data, tries opening the file, and if _hasHeader is true, reads one line as the header. It then splits and stores column names. After that, it loops through the file, line by line, ignoring empty lines and splitting valid ones into fields. Each set of fields becomes a CArrayString row in Rows. By the end, you know exactly how many rows you got, and Columns is ready for name-based lookups. This straightforward flow means your EA can adapt easily if tomorrow’s CSV has more rows or slightly different formatting.
Regarding GetValueByName() and GetValueByIndex() methods, These accessor methods are your main interface to the data. They’re safe because they always check boundaries. If you request a row or column that doesn’t exist, you get a harmless default rather than a crash. If there’s no header, GetValueByName() gracefully returns an error value. This way, even if your CSV is missing something or _hasHeader is set incorrectly, your EA won’t break. You might add a quick Print() statement if you want to log these mismatches, but that’s optional. The point is: these methods keep your workflow smooth and error-free.
If params.csv looks like this:
Symbol;MaxLot;MinSpread
EURUSD;0.20;1
GBPUSD;0.10;2
Output:
Loaded 2 data rows. First Row: Symbol=EURUSD MaxLot=0.20 MinSpread=1
And if you want to access by index instead of name:
// Access second row, second column (MaxLot) by index: string val = csv.GetValueByIndex(1, 1, "N/A"); Print("Second row, second column:", val);
This should print 0.10 corresponding to GBPUSD’s MaxLot.
What if No Header is Present? If _hasHeader is set to false, we skip creating the Columns map. Then you must rely on GetValueByIndex() to access data. For example, if your CSV doesn’t have headers and each row has three fields, you know:
- Column 0: Symbol
- Column 1: Price
- Column 2: Comment
You can directly call csv.GetValueByIndex(rowNum, 0) to get the symbol.
What about error handling? Our code returns default values if something’s missing, such as a non-existent column or row. It also prints errors if the file can’t be opened. In practice, you might want more robust logging. For example, if you rely heavily on external data, consider checking rows = csv.Load("file.csv") and if rows == 0 , handle it gracefully. Maybe you abort your EA initialization or revert to internal defaults.
We haven’t implemented extreme error handling for malformed CSVs or unusual encodings. For more complex scenarios, add checks. If ColumnCount() is zero, maybe log a warning. If a needed column doesn’t exist, print a message in the Experts tab.
Let take a look at the performance, for small to moderate CSV files, this approach is perfectly fine. If you need to handle extremely large files, consider more efficient data structures or a streaming approach. However, for typical EA usage – such as reading a few hundred or thousand lines – this will perform adequately.
We now have a complete CSV reader. In the next (and final) section, we’ll briefly discuss testing, provide some usage scenarios, and wrap up with concluding remarks. You’ll walk away with a ready-to-use CSV reader class that integrates smoothly with your MQL5 EAs or scripts.
Testing & Usage Scenarios
With the CSV reader implementation complete, it’s wise to confirm everything works as intended. Testing is straightforward: create a small CSV file, put it in MQL5/Files , and write an EA that loads it and prints some results. You can then check the Experts tab to see if the values are correct. Here are a few test suggestions:
-
Basic Test with Header: Create a test.csv like:
Symbol;Spread;Comment EURUSD;1;Major Pair USDJPY;2;Another Major
Load it with:
CSimpleCSVReader csv; csv.SetHasHeader(true); csv.SetSeparator(";"); uint rows = csv.Load("test.csv"); Print("Rows loaded: ", rows); Print("EURUSD Spread: ", csv.GetValueByName(0, "Spread", "N/A")); Print("USDJPY Comment: ", csv.GetValueByName(1, "Comment", "N/A"));
Check the output. If it shows “Rows loaded: 2”, “EURUSD Spread: 1” and “USDJPY Comment: Another Major”, then it’s working.
What if the CSV isn’t perfectly uniform? Suppose one row has fewer columns than expected. Our approach doesn’t force consistency. If a row is missing a field, asking for that column returns a default. This is good if you can handle partial data, but if you need strict formatting, consider verifying column counts after Load() . For huge files, this method still works fine, though if you’re pushing tens of thousands of lines, you might start thinking about performance optimizations or partial loading. For everyday needs – small to medium CSVs – this setup is more than enough.
-
No Header Test: If you set csv.SetHasHeader(false); and use a file without a header:
EURUSD;1;Major Pair USDJPY;2;Another Major
Now you must access columns by index:
string val = csv.GetValueByIndex(0, 0, "N/A"); // should be EURUSD Print("Row0 Col0: ", val);
Confirm that the output matches your expectations. - Missing Columns or Rows: Try requesting a column name that doesn’t exist, or a row beyond the loaded data. You should get the default error values you provided. For example:
string nonExistent = csv.GetValueByName(0, "NonExistentColumn", "MISSING"); Print("NonExistent: ", nonExistent);
This should print MISSING rather than crash. - Larger Files: If you have a file with more rows, load it and confirm the row count. Check that memory usage and performance remain reasonable. This step helps ensure that the approach is robust enough for your scenario.
Also consider character encodings and unusual symbols. Most CSVs are plain ASCII or UTF-8, which MQL5 handles nicely. If you ever get strange characters, converting the file to a friendlier encoding first might help. Similarly, if your CSV has trailing whitespace or odd punctuation, trimming fields after splitting ensures cleaner data. Testing these “less pretty” scenarios now ensures that when your EA runs for real, it won’t choke on a slightly different file format or unexpected glyph.
Usage Scenarios:
-
External Parameters:
Suppose you have a CSV with strategy parameters. Each row might define a symbol and some thresholds. Instead of hardcoding these values in your EA, you can load them at startup, iterate over the rows, and apply them dynamically. Changing parameters becomes as easy as editing the CSV, no recompilation required. -
Watchlist Management:
You might store a list of symbols to trade in a CSV file. Your EA can read this list at runtime, enabling you to quickly add or remove instruments without touching the code. For example, a CSV might have:Symbol EURUSD GBPUSD XAUUSD
Reading this file and iterating over rows in your EA allows you to adapt the traded symbols on the fly. - Integration with Other Tools: If you have a Python script or another tool generating CSV analytics – like custom signals or forecasts – you can export the data to CSV and have your EA import it in MQL5. This bridges the gap between different programming ecosystems.
Conclusion
We’ve now explored the fundamentals of MQL5 file operations, learned how to safely read text files line by line, parse CSV lines into fields, and store them for easy retrieval by column names or indexes. By presenting the full code for a simple CSV reader, we’ve provided a building block that can enhance your automated trading strategies.
This CSV reader class is not just a code snippet; it’s a practical utility you can adapt to suit your needs. Need a different delimiter? Change _separator. No header in your file? Set _hasHeader to false and rely on indexes. The approach is flexible and transparent, allowing you to integrate external data cleanly. As you continue to develop more complex trading ideas, you might extend this CSV reader further – adding more robust error handling, supporting different encodings, or even writing back to CSV files. For now, this foundation should cover most basic scenarios.
Remember, reliable data is key to building solid trading logic. With the ability to import external data from CSV files, you can harness a broader range of market insights, configurations, and parameter sets, all dynamically controlled by simple text files rather than hardcoded values and if your needs grow more complex – like handling multiple delimiters, ignoring certain lines, or supporting quoted fields – just tweak the code. That’s the beauty of having your own CSV reader: it’s a solid base you can refine as your strategy and data sources evolve. Over time, you might even build a mini data toolkit around it, always ready to feed your EA new insights without rewriting the core logic from scratch.
Happy coding, and happy trading!





- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use