- Patrik Simon: Whats wrong with this code?
Please edit your (original) post and use the CODE button (or Alt+S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum #25 (2019)
Messages Editor
Forum rules and recommendations - General - MQL5 programming forum (2023) -
double rsiCurrent = iRSI(Symbol(), PERIOD_M1, RSI_Length, PRICE_CLOSE, 0); double rsiPrevious = iRSI(Symbol(), PERIOD_M1, RSI_Length, PRICE_CLOSE, 1);
Those are MT4 calls.
Perhaps you should read the manual, especially the examples.
How To Ask Questions The Smart Way. (2004)
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up.They all (including iCustom) return a handle (an int). You get that in OnInit. In OnTick/OnCalculate (after the indicator has updated its buffers), you use the handle, shift and count to get the data.
Technical Indicators - Reference on algorithmic/automated trading language for MetaTrader 5
Timeseries and Indicators Access / CopyBuffer - Reference on algorithmic/automated trading language for MetaTrader 5
How to start with MQL5 - General - MQL5 programming forum - Page 3 #22 (2020)
How to start with MQL5 - MetaTrader 5 - General - MQL5 programming forum - Page 7 #61 (2020)
MQL5 for Newbies: Guide to Using Technical Indicators in Expert Advisors - MQL5 Articles (2010)
How to call indicators in MQL5 - MQL5 Articles (2010) -
takeProfit = SymbolInfoDouble(Symbol(), SYMBOL_ASK) + 2 * (SymbolInfoDouble(Symbol(), SYMBOL_ASK) - stopLoss); // 2RR take profit
You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit by the Ask.
-
Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid / OrderClosePrice reaches it. Using Ask±n, makes your SL shorter and your TP longer, by the spread. Don't you want the specified amount used in either direction?
-
Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask / OrderClosePrice reaches it. To trigger close at a specific Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 -
The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (control+O) → charts → Show ask line.)
Most brokers with variable spreads widen considerably at end of day (5 PM ET) ± 30 minutes.
My GBPJPY shows average spread = 26 points, average maximum spread = 134.
My EURCHF shows average spread = 18 points, average maximum spread = 106.
(your broker will be similar).
Is it reasonable to have such a huge spreads (20 PIP spreads) in EURCHF? - General - MQL5 programming forum (2022)
-
Use the documentation reference. Or press F1 key after highlighting the function name
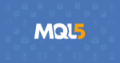
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Whats wrong with this code?