How do i get coding to trade on specific time 08:00-20:00 on day of week - Tuesday to Thursday and from April to December on MQL5
- Trade Balance - USA - Fundamental Analysis - Price Charts, Technical and Fundamental Analysis
- MetaEditor - Professional editor of trading applications
- Getting Started
You have only four choices:
-
Search for it (CodeBase or Market). Do you expect us to do your research for you?
- Try asking at:
- Coding help - MQL4 programming forum
- Requests & Ideas - MQL4 programming forum
- Make It No Repaint Please! - MQL4 programming forum
- MT4 to MT5 code converter - MQL5 programming forum
- Please fix this indicator or EA - General - MQL5 programming forum
- Requests & Ideas (MQL5 only!) - Expert Advisors and Automated Trading - MQL5 programming forum
- Indicator to EA Free Service - General - MQL5 programming forum
- I will write an advisor free of charge - Expert Advisors and Automated Trading - MQL5 programming forum
- I will write you an advisor for free - Trading Systems - MQL5 programming forum
- I will write the indicator for free - MQL4 programming forum
-
MT4: Learn to code it.
MT5: Begin learning to code it.If you don't learn MQL4/5, there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into your code.
I need HEEEELP, please, it's URGENT...really ! - General - MQL5 programming forum (2017) -
Or pay (Freelance) someone to code it. Top of every page is the link Freelance.
Hiring to write script - General - MQL5 programming forum (2019)
We're not going to code it for you (although it could happen if you are lucky or the problem is interesting.) We are willing to help you when you post your attempt (using CODE button) and state the nature of your problem.
No free help (2017)
Quite complicate for me to coding this kind of function. Someone has knowledge please help.
Specifying Trading Time
To specify the time you want to trade during the day, here is a simple solution:
string start = "08:00"; string end = "20:00"; void OnTick() { datetime start_time = StringToTime(start); datetime end_time = StringToTime(end); datetime current_time = TimeCurrent(); if(current_time >= start_time && current_time <= end_time) { //your conditions } }
Explanation:
string start = "08:00"; string end = "20:00";
- 'start' and ' end' are string variables that define the start and end times in the format "HH:MM". In this case, the trading period starts at 08:00 (8 AM) and ends at 20:00 (8 PM).
datetime start_time = StringToTime(start); datetime end_time = StringToTime(end); datetime current_time = TimeCurrent();
- 'StringToTime(start)' converts the start string ("08:00") into a datetime value representing 08:00 on the current day.
- ' StringToTime(end)' converts the end string ("20:00") into a datetime value representing 20:00 on the current day.
- TimeCurrent() gets the current server time as a datetime value.
if(current_time >= start_time && current_time <= end_time) { // your conditions }
- This condition checks whether the current time ( current_time ) falls within the specified start ( start_time ) and end ( end_time ) times.
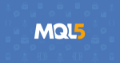
- www.mql5.com
Quite complicate for me to coding this kind of function. Someone has knowledge please help.
Specifying Trading Weekdays
To specify the days of the week you want to trade, here is a simple solution:
void OnTick() { // Declare an MqlDateTime structure to hold the current time and date MqlDateTime day; // Get the current time and fill the MqlDateTime structure TimeCurrent(day); // Extract the day of the week (0 = Sunday, 1 = Monday, ..., 6 = Saturday) int week_day = day.day_of_week; // Check if the current day is between Tuesday (2) and Thursday (4) if(week_day >= 2 && week_day <= 4) { // Your conditions } }
Explanation:
- MqlDateTime day;: Declares a variable day of type MqlDateTime to hold date and time information.
- TimeCurrent(day);: Gets the current time and fills the day structure with the current date and time details.
- int week_day = day.day_of_week;: Extracts the day of the week from the day structure. The day_of_week member ranges from 0 (Sunday) to 6 (Saturday).
- if(week_day >= 2 && week_day <= 4): Checks if the current day of the week is between Tuesday (2) and Thursday (4).
- { // Your conditions }: Executes the code within the braces if the condition is met.
Quite complicate for me to coding this kind of function. Someone has knowledge please help.
Specifying Trading Months
In MQL5, the MqlDateTime structure represents dates and times, where months ar counted starting from 1 for January to 12 for December. Here's an explanation of your OnTick() function:
void OnTick() { // Declare a structure to hold current month information MqlDateTime month; // Get the current date and time TimeCurrent(month); // Extract the month component (1 for January, 2 for February, ..., 12 for December) int year_month = month.mon; // Print the current month number Print(year_month); // Check if the current month is between April (4) and December (12) if(year_month >= 4 && year_month <= 12) { //your conditions } }
Explanation:
- MqlDateTime: This structure is used to store date and time information. When you call TimeCurrent(month) , it populates the month variable with the current date and time details.
- month.mon: This retrieves the month component from the MqlDateTime structure. As mentioned, months in MQL5 are numbered from 1 to 12.
- Print(year_month): This line prints the current month number to the Experts tab in MetaTrader's Terminal window, allowing you to monitor the output during EA operation.if (year_month >= 4 && year_month <= 12): This condition checks if the current month ( year_month ) falls within the range from April (4) to December (12). If true, the block of code within the if statement will execute.
I hope you find this helpful. I will attach a file that includes all the codes, so you'll get a better understanding and use it for your EA if needed.
Thank you Israel Pelumi Abioye. This is my coding to share. I hope to get comments to improve my skill. Originally it did'nt work as I want. I will learn from Israel Pelumi Abioye gave me your coding as well.
input int TimeStartHour = 8 ;
input int TimeStartMin = 00 ;
input int TimeEndHour = 20;
input int TimeEndMin = 00 ;
input int StartDayOfWeek = 2 ;
input int EndDayOfWeek = 4 ;
input int StartMonth = 4 ;
input int EndMonth = 12;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//---
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//---
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
{
MqlDateTime structTime;
TimeCurrent (structTime);
structTime.sec = 0;
structTime.hour = TimeStartHour;
structTime.min = TimeStartMin;
datetime timeStart = StructToTime(structTime);
structTime.hour = TimeEndHour;
structTime.min = TimeEndMin;
datetime timeEnd = StructToTime(structTime);
structTime.day_of_week = StartDayOfWeek;
datetime weekdaystart = StructToTime(structTime);
structTime.day_of_week = EndDayOfWeek;
datetime weekdayend = StructToTime(structTime);
structTime.mon = StartMonth ;
datetime startmonth = StructToTime(structTime);
structTime.mon = EndMonth;
datetime endmonth = StructToTime(structTime);
bool isTime = TimeCurrent()>=timeStart&&TimeCurrent()<timeEnd&&TimeCurrent()>=weekdaystart&&TimeCurrent()<=weekdayend&&TimeCurrent()>=startmonth&&TimeCurrent()<=endmonth;
Print ("Currenttime :",TimeCurrent(),
"\nendmonth:",endmonth);
if(isTime == true)
{
Comment("Trading Time");
}
else {
Comment("No trad");
}
}
Thank you Israel Pelumi Abioye. This is my coding to share. I hope to get comments to improve my skill. Originally it did'nt work as I want. I will learn from Israel Pelumi Abioye gave me your coding as well.
input int TimeStartHour = 8 ;
input int TimeStartMin = 00 ;
input int TimeEndHour = 20;
input int TimeEndMin = 00 ;
input int StartDayOfWeek = 2 ;
input int EndDayOfWeek = 4 ;
input int StartMonth = 4 ;
input int EndMonth = 12;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//---
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//---
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
{
MqlDateTime structTime;
TimeCurrent (structTime);
structTime.sec = 0;
structTime.hour = TimeStartHour;
structTime.min = TimeStartMin;
datetime timeStart = StructToTime(structTime);
structTime.hour = TimeEndHour;
structTime.min = TimeEndMin;
datetime timeEnd = StructToTime(structTime);
structTime.day_of_week = StartDayOfWeek;
datetime weekdaystart = StructToTime(structTime);
structTime.day_of_week = EndDayOfWeek;
datetime weekdayend = StructToTime(structTime);
structTime.mon = StartMonth ;
datetime startmonth = StructToTime(structTime);
structTime.mon = EndMonth;
datetime endmonth = StructToTime(structTime);
bool isTime = TimeCurrent()>=timeStart&&TimeCurrent()<timeEnd&&TimeCurrent()>=weekdaystart&&TimeCurrent()<=weekdayend&&TimeCurrent()>=startmonth&&TimeCurrent()<=endmonth;
Print ("Currenttime :",TimeCurrent(),
"\nendmonth:",endmonth);
if(isTime == true)
{
Comment("Trading Time");
}
else {
Comment("No trad");
}
}
It might not be very clear in the API but for MqlDateTime day_of_week here are the codes:
- 0: Sunday
- 1: Monday
- 2: Tuesday
- 3: Wednesday
- 4: Thursday
- 5: Friday
- 6: Saturday
as you saw in his condition above, day_of_week understands a number
Thank you Israel Pelumi Abioye. This is my coding to share. I hope to get comments to improve my skill. Originally it did'nt work as I want. I will learn from Israel Pelumi Abioye gave me your coding as well.
...Improperly formatted post. Please use the CODE button (Alt-S) when inserting code — https://www.mql5.com/en/articles/24#insert-code
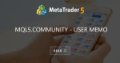
- www.mql5.com
Improperly formatted post. Please use the CODE button (Alt-S) when inserting code — https://www.mql5.com/en/articles/24#insert-code

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use