
You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
you could start by this article: https://www.mql5.com/en/articles/12751
you could start by this article: https://www.mql5.com/en/articles/12751
Great...Thanks a ton!
I already gave you an example 4 years ago :))
Forum on trading, automated trading systems and testing trading strategies
how to convert all objects into 1 object
Nikolai Semko, 2019.10.05 22:39
Indeed, Canvas is the solution.
And Canvas is much easier than it seems at first glance.
Here is a primitive example of an indicator (MQL5 & MQL4) with one object OBJ_BITMAP_LABEL in which there are many windows.
n this new article, another option for implementing Information panels.
https://www.mql5.com/ru/articles/13179
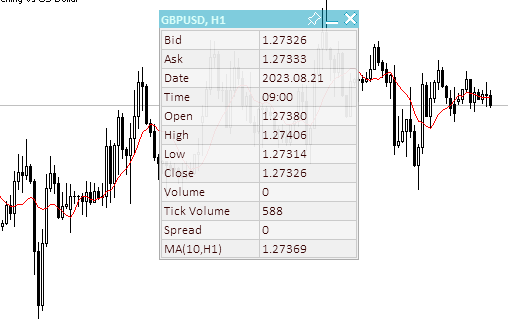
Here, for example, I have sketched a script that demonstrates it clearly. On the right is this fast algorithm, and on the left is mine (about 4-10 times slower).
.
I can reduce the Bmp picture, but the canvas remains the same size. For the same reason I can't enlarge the picture, it is cropped by the original size, i.e. the size of the canvas.
I don't fully understand arrays, so can you explain it to me, or better show me an example with code:
1. Image enlargement
2. A canvas equal to the size of the picture if we have reduced or enlarged it.
Thank you.
Nikolai Semko, hi. In this example https://www.mql5.com/ru/forum/227736/page66#comment_20456641 you have demonstrated Bmp resizing (reducing).
I can reduce the Bmp picture, but the canvas remains the same size. For the same reason I can't enlarge the image, it is cropped by the original size, i.e. the size of the canvas.
I don't fully understand arrays, so can you explain it to me, or better show me an example with code:
1. Image enlargement
2. A canvas equal to the size of the picture if we have reduced or enlarged it.
Thank you.
Cool and useful. Just for interest, can this be used in MT4?
Good day to all. I am also interested in this code. But when using Resize(), it does not work. Maybe it should be done in a different sequence.
Good day to all. I am also interested in this code. But when using Resize(), it does not work. Maybe it should be done in a different sequence.
Okay, I'll give you an example a little later.
I would be very grateful for a code example. Thanks in advance...
Okay, I'll give you an example a little later.
Here is an example based on the png.mql library, when moving the mouse changes the position of the canvas and changes its size. If the mouse pointer is in the centre horizontally, it corresponds to the original size of the image, if to the left - zoom out, to the right - zoom in.
Only I had to rethrow variables C and _C in png.mql from private to public. So you need to overwrite this library if it is already installed.
This example has two modes of operation (input variable bool resize_canvas):
That's why I prefer to use one canvas for the whole screen!