am i doing something wrong somewhere? This Ea Refuse to place Order @ the open And Close of candlesticks
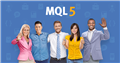
Discover new MetaTrader 5 opportunities with MQL5 community and services
- www.mql5.com
Ask questions on technical analysis, discuss trading systems and improve your MQL5 programming skills to develop your own trading strategies. Communicate and share your experience with traders from anywhere in the world, answer questions and help beginners — MQL5.community is developing along with you. Taking Neural Networks to the next level...
D p Canidae: am i doing something wrong somewhere?
- Please edit your (original) post and use the CODE
button (Alt-S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor -
double ticketsize = MarketInfo(Symbol(), MODE_TICKSIZE);
Don't try to use any price or server related functions in OnInit (or on load,) as there may be no connection/chart yet:- Terminal starts.
- Indicators/EAs are loaded. Static and globally declared variables are initialized. (Do not depend on a specific order.)
- OnInit is called.
- For indicators OnCalculate is called with any existing history.
- Human may have to enter password, connection to server begins.
- New history is received, OnCalculate called again.
- New tick is received, OnCalculate/OnTick is called. Now TickValue, TimeCurrent, account information and prices are valid.
- For a new bar test, Bars is unreliable (a refresh/reconnect can change number of bars on chart,) volume is unreliable
(miss ticks,) Price is unreliable (duplicate prices and
The == operand. - MQL4 programming forum.) Always use time.
I disagree with making a new bar function, because it can only be called once per tick. A variable can be tested multiple times. You are calling your function multiple times per tick.
New candle - MQL4 programming forum -
if(OrdersTotal() == 0)
Using OrdersTotal (or OrdersHistoryTotal) directly and/or no Magic number filtering on your OrderSelect loop means your code is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 programming forum
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles -
BuyTicket = OrderSend(Symbol(),OP_BUY,LotSize,Ask,0,Ask-(StopLoss*Pips), Ask+(TakeProfit*Pips),NULL,Magicnumber,0,Green);
You buy at the Ask and sell at the Bid. So for buy orders you pay the spread on open. For sell orders you pay the spread on close.- Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid reaches it. Not the Ask. Your SL is shorter by the spread and your TP is longer. Don't you want the same/specified amount for either direction?
- Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask reaches it. To
trigger at a specific
Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 - The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (Control-O) → charts → Show ask line.)
-
if(BuyTicket == -1) { ErrorCode = GetLastError();
Check your return codes for errors, and report them including GLE/LE. Don't look at GLE/LE. unless you have an error. You are looking at your variable, even if you do not try to open an orwder.
What are Function return values ? How do I use them ? - MQL4 programming forum
Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articles - Don't use NULL.
- You can use NULL in place of _Symbol only in those calls that the documentation specially says you can. iHigh does, iCustom does, MarketInfo does not. OrderSend does not.
- Don't use NULL (except for pointers where you explicitly check for it.) Use _Symbol and _Period, that is minimalist as possible and more efficient.
- Zero is the same as PERIOD_CURRENT which means _Period. Don't hard code numbers.
- MT4: No need for a function call with iHigh(NULL,0,s) just use the predefined arrays, i.e. High[].
- Use the debugger or print out your variables, including _LastError and find out why.
-
double COneOpen = iOpen(NULL,0,OpenCO); double COneClose =iClose(NULL,0,CloseCO); if(COneOpen < COneClos
On the first tick of a new candle, open[0] and close[0] will usually be the same.
Thanks a lot your always helpful..
i added ZigZag indicator to my Ea after fixing the problems your address and the trailing stop started moving both ways
CheckCandleTrade() function checks for trade entry
void CheckCandleTrade() { double COneOpen = iOpen(_Symbol,_Period,OpenCO); double COneClose =iClose(_Symbol,_Period,CloseCO); double CTwoOpen = iOpen(_Symbol,_Period,OpenCT); double CTwoClose =iClose(_Symbol,_Period,CloseCT); //----------------|Custom ZigZag |----------------------------- double ZigZagHigh = iCustom(_Symbol,_Period,"ZigZag",InpDepth,InpDeviation,InpBackstep,1,1); double ZigZagLow = iCustom(_Symbol,_Period,"ZigZag",InpDepth,InpDeviation,InpBackstep,2,1); //Print("ZigZagHigh: ",ZigZagHigh ," ZigZagLow: ",ZigZagLow," Time: "+TimeToStr(Time[1])); if(ZigZagHigh > 0 && COneOpen < COneClose && CTwoOpen < CTwoClose) OrderEntry(0); if(ZigZagLow > 1 && COneOpen > COneClose && CTwoOpen > CTwoClose) OrderEntry(1); }
and i'm using candle trailing Stop.
but the Stop opens the locked profits, how can i fix this?
void AdjustTrail() { //Buy Order section for(int b = OrdersTotal()-1; b>=0; b--) { if(OrderSelect(b,SELECT_BY_POS,MODE_TRADES)) if(OrderMagicNumber()== Magicnumber) if(OrderSymbol()== Symbol()) if(OrderType()==OP_BUY) if(UseCandleTrail) { if(IsNewCandle()) if(OrderStopLoss()< Low[StopCandle] - PadAmount*Pips) int ms=OrderModify(OrderTicket(),OrderOpenPrice(),Low[1]-PadAmount*Pips,OrderTakeProfit(),0,CLR_NONE); } } //Sell Order section for(int s=OrdersTotal()-1; s>=0; s--) { if(OrderSelect(s,SELECT_BY_POS,MODE_TRADES)) if(OrderMagicNumber()== Magicnumber) if(OrderSymbol() == Symbol()) if(OrderType() == OP_SELL) if(UseCandleTrail) { if(IsNewCandle()) if(OrderStopLoss()>High[StopCandle] + PadAmount*Pips) int ms=OrderModify(OrderTicket(),OrderOpenPrice(),High[1]+PadAmount*Pips,OrderTakeProfit(),0,CLR_NONE); } } }

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register