2. CCandle 클래스는 CObject의 상속자이기 때문에 우리가 그리고 싶은 모든 양초를 CArrayObj 클래스의 객체에 순차적으로 쓸 수 있습니다. 이 배열은 cbdata 매개변수로 사용자 정의 렌더 메소드에 전달됩니다. 결과적으로 양초를 그리는 방법은 다음과 같습니다.
//+------------------------------------------------------------------+//| Custom method for plot candles |//+------------------------------------------------------------------+void PlotCandles( double &x[], double &y[], int size,CGraphic *graphic,CCanvas *canvas, void *cbdata)
{
//--- check obj
CArrayObj *candles=dynamic_cast<CArrayObj*>(cbdata);
if (candles== NULL || candles.Total()!=size)
return ;
//--- plot candles for ( int i= 0 ; i<size; i++)
{
CCandle *candle=dynamic_cast<CCandle*>(candles.At(i));
if (candle== NULL )
return ;
//--- primary calculateint xc=graphic.ScaleX(x[i]);
int width_2=candle.CandleWidth()/ 2 ;
int open=graphic.ScaleY(candle.OpenValue());
int close=graphic.ScaleY(candle.CloseValue());
int high=graphic.ScaleY(candle.HigthValue());
int low=graphic.ScaleY(candle.LowValue());
uint clr=(open<=close) ? candle.CandleColorIncrement() : candle.CandleColorDecrement();
//--- plot candle
canvas.LineVertical(xc,high,low, 0x000000 );
//--- plot candle real body
canvas.FillRectangle(xc+width_2,open,xc-width_2,close,clr);
canvas.Rectangle(xc+width_2,open,xc-width_2,close, 0x000000 );
}
}
삼. 단순화를 위해 모든 양초는 무작위로 생성됩니다. 그래서 우리는 순차적으로 10개의 양초를 생성하고 CArrayObj 클래스의 객체로 채웁니다. CGraphics 개체를 만들고 여기에 하나의 곡선을 추가한 후 PlotCandles 함수를 기반으로 그려질 것임을 나타냅니다. 또한 촛불이 완전히 보이도록 y축의 최대값과 최소값을 변경해야 합니다.
CGraphic 클래스에서 요청한 대로 모든 위치에서 색상 유형을 uint로 대체했습니다.
또한 CCanvas 클래스에 주어진 두께로 기본 요소를 그릴 수 있는 새로운 메서드를 추가했습니다.
혁신에 따라 CCurve는 CCurve의 속성을 확장했습니다.
이제 선으로 곡선을 그릴 때 선의 굵기와 끝 부분의 스타일을 설정할 수 있습니다.
예, 정말 대단합니다.
스플라인 작업(베지어 곡선에 의한 보간)이 수정되었습니다. 구현이 CGraphics 클래스에서 CCanvas로 직접 이동되어 그래픽 라이브러리 외부에서 스플라인을 빌드할 수 있습니다.
또한 닫힌 스플라인을 그리는 알고리즘이 추가되었습니다.
결과적으로 두 개의 새로운 공용 메서드가 CCCanvas 클래스 에 나타났습니다.
이 방법을 사용하면 주어진 스타일과 두께로 스플라인을 그릴 수 있습니다.
베지어 곡선이 원과 타원을 정확하게 묘사한다는 사실 때문에 주어진 두께로 이러한 프리미티브를 그리는 새로운 방법으로 CCanvas 클래스를 보완할 필요가 없습니다.
PolygoneSmooth 방법에 기반한 베지어 곡선에 의한 타원 근사의 예:
결과:
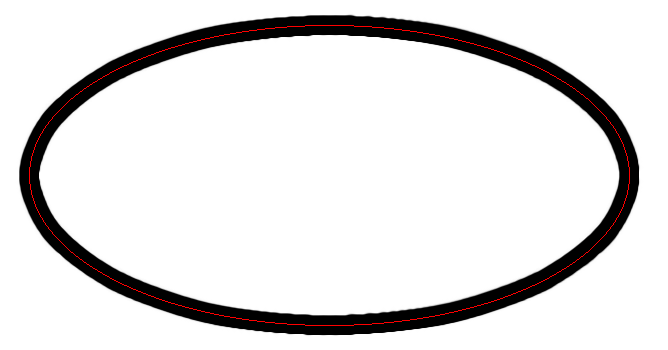
그래픽 라이브러리의 보편성을 향한 또 다른 가능한 단계: 곡선 CURVE_CUSTOM 그리기를 위한 사용자 정의 모드.
이 모드는 표준 라이브러리 도구가 허용하는 것과 다르게 곡선을 그리기 위해 CGraphic 클래스의 상속자를 만들고 ...Plot 메서드를 오버로드할 필요가 없습니다.
이 CURVE_CUSTOM 모드를 구현하기 위해 CCurve 클래스에 새 속성이 추가됩니다.
새로운 함수 포인터 PlotFucntion을 기반으로 합니다.
이 접근 방식은 차트를 그릴 수 있는 새로운 가능성을 열어줍니다.
예를 들어, CGraphics 라이브러리에서 촛대를 구축해 보겠습니다.
1. 하나의 양초에 대한 모든 데이터가 저장될 컨테이너 클래스를 생성합시다.
2. CCandle 클래스는 CObject의 상속자이기 때문에 우리가 그리고 싶은 모든 양초를 CArrayObj 클래스의 객체에 순차적으로 쓸 수 있습니다. 이 배열은 cbdata 매개변수로 사용자 정의 렌더 메소드에 전달됩니다. 결과적으로 양초를 그리는 방법은 다음과 같습니다.
삼. 단순화를 위해 모든 양초는 무작위로 생성됩니다. 그래서 우리는 순차적으로 10개의 양초를 생성하고 CArrayObj 클래스의 객체로 채웁니다. CGraphics 개체를 만들고 여기에 하나의 곡선을 추가한 후 PlotCandles 함수를 기반으로 그려질 것임을 나타냅니다. 또한 촛불이 완전히 보이도록 y축의 최대값과 최소값을 변경해야 합니다.
결과적으로 다음 그래프를 얻습니다.
@로만 코노펠코
CGraphic::SetDefaultParameters 함수에 작은 오류가 있습니다.
색상은 불투명도를 염두에 두고 초기화해야 합니다.
@로만 코노펠코
CGraphic::SetDefaultParameters 함수에 작은 오류가 있습니다.
색상은 불투명도를 염두에 두고 초기화해야 합니다.
다음은 컴퓨터를 정지시킨 예입니다. 내가 한 일 : 편집기 에서 차트에 표시기를 붙인 후 다양한 조합으로 재생하여 87 및 88 행 주석 처리 / 주석 해제 (한 번에 하나씩, 함께 할 때)
업적을 두 번 반복했습니다. 단계를 기록하지 않은 것이 아쉽습니다. 세 번째는 확인하기가 두렵습니다.
추가됨: 빌드 1607 x64
다음은 컴퓨터를 정지시킨 예입니다. 내가 한 일 : 편집기 에서 차트에 표시기를 붙인 후 다양한 조합으로 재생하여 87 및 88 행 주석 처리 / 주석 해제 (한 번에 하나씩, 함께 할 때)
업적을 두 번 반복했습니다. 단계를 기록하지 않은 것이 아쉽습니다. 세 번째는 확인하기가 두렵습니다.
추가됨: 빌드 1607 x64
오늘 나는 기록을 반복했습니다. 컴퓨터가 완전히 정지되어 RAM 소비가 2GB에서 5.5GB로 증가했습니다. 차트를 닫는 데 성공한 것 같지만 컴퓨터가 5분 동안 중단되었습니다.
이번에는 300개 이하의 요소로 배열 크기에 대한 제한을 도입했습니다. 분명히 도움이되지 않았습니다.
디버그는 무엇을 말합니까?
디버그는 무엇을 말합니까?
Zhebug는 도달하지 못했습니다. 나는 이것을했습니다 : 표시기를 매달고 주석을 달지 않고 한두 줄을 주석 처리하고 컴파일했습니다. 결과적으로 타블렛에서 글을 쓰다보니 노트북이 나가버렸네요..
Zhebug는 도달하지 못했습니다. 나는 이것을했습니다 : 표시기를 매달고 주석을 달지 않고 한두 줄을 주석 처리하고 컴파일했습니다. 결과적으로 타블렛에서 글을 쓰다보니 노트북이 나가버렸네요..
따라서 하드 리셋 후 랩톱이 활성화되었지만 파괴적인 실험을 계속하려는 열망은 사라졌습니다. 랩톱에서 여러 Expert Advisors가 회전하고 있으므로 한 시간 동안 정지하려는 욕구가 없습니다.