- Templates
- Types of Execution - Trading Principles - Trade - MetaTrader 5 for Android
- Instant Execution - Opening and Closing Positions - Trade - MetaTrader 5 for iPhone
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
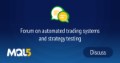
- www.mql5.com
You are mixing MQL4 with MQL5. The indicator functions like iMA(), do not return buffer values. It returns a handle which you use with CopyBuffer() function.
Please reference the documentation on the correct way to use the iMA() function in MQL5.
And, in case this is ChatGPT code, then please stop using.
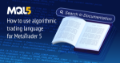
- www.mql5.com
Hi
As was mentioned above – this is code in mq5 and the MA and RSI return handles not buffer values. You need to add those functions in the OnInit and here use CopyBuffer functions to get the values like I showed you below – example is for getting values from last closed bar. Also you don’t need those ObjectFind functions.
int bar =1; double rsivalues[1]; int copied1 = CopyBuffer(rsi,0,bar,1,rsivalues); double ema20values[1]; int copied2 = CopyBuffer(ema_20,0,bar,1,ema20values); double ema50values[1]; int copied3 = CopyBuffer(ema_50,0,bar,1,ema50values); ... if(ema20values
Have a nice day👍📊
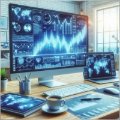
Fernando Carreiro #:
You "get it", by learning it, by reading the documentation or the book and practising to code. This website is for helping those willing to learn, not for fixing the output from ChatGPT. If that is what you need, then consider using the Freelance section instead.
Can you provide me with a link where I can read the MT5 language?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use