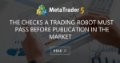
- www.mql5.com
Verify the Minimum and Maximum volumes at the end, not at the beginning ...
Forum on trading, automated trading systems and testing trading strategies
Market Registration of EA Unable to Validate
Fernando Carreiro, 2022.08.30 14:20
For your screenshot with an Error 131 and it also has a link on "How to fix it". So follow up on it and fix your EA accordingly.
In regards to volume, you have to check the contract specification of the symbol and limit your volume to the minimum, maximum and step that is allowed for the symbol.
// Variables for symbol volume conditions double dbLotsMinimum = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_MIN ), dbLotsMaximum = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_MAX ), dbLotsStep = SymbolInfoDouble( _Symbol, SYMBOL_VOLUME_STEP ); // Adjust volume for allowable conditions dbLots = fmin( dbLotsMaximum, // Prevent too greater volume fmax( dbLotsMinimum, // Prevent too smaller volume round( dbLots / dbLotsStep ) * dbLotsStep ) ); // Align to step value
Thanks, your suggestion and code works locally but, when I submit the program again I get the exact same error. I even had to add the limit volume condition just in case, the error was coming from that angle.
double PositionsVolume() { double vol= 0; for (int i=PositionsTotal()-1; i>=0; i--) if (m_position.SelectByIndex(i)) if (m_position.Symbol()==Symbol()) vol += m_position.Volume(); for (int i=OrdersTotal()-1; i>=0; i--) if (m_order.SelectByIndex(i)) if (m_order.Symbol()==Symbol()) vol += m_order.VolumeCurrent(); return vol; }
Then;
if (CheckMoneyForTrade(lotsize, ORDER_TYPE_SELL)) { if (volume_limit ==0 ? true : (lotsize + PositionsVolume()) < volume_limit) m_trade.Sell(lotsize, Symbol(), ticks.bid, NormalizeDouble(intern_sl, Digits()), NormalizeDouble(intern_tp, Digits())); //open a sell trade }
I keep on getting the same error, still.
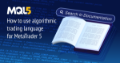
- www.mql5.com
Yes, you also have to also check for total volume limit per symbol (SYMBOL_VOLUME_LIMIT), and for pending orders check the initial volume requested (ORDER_VOLUME_INITIAL).
Also, in your validation process, it is failing on a "netting" account, not a "hedging" account. There may be something else in your code that is contributing to the error due to the difference of account types. So make sure you test on a "netting" account on your own local testing.
Yes, you also have to also check for total volume limit per symbol ( SYMBOL_VOLUME_LIMIT ), and for pending orders check the initial volume requested ( ORDER_VOLUME_INITIAL).
Also, in your validation process, it is failing on a "netting" account, not a "hedging" account. There may be something else in your code that is contributing to the error due to the difference of account types. So make sure you test on a "netting" account on your own local testing.
Yes, I agree. Let me find a netting broker and test in that specific environment.
Probably not related at all, but this is strange condition, mixing "if" and "?" operator :
if (volume_limit ==0 ? true : (lotsize + PositionsVolume()) < volume_limit)
You should avoid that style.
if (volume_limit ==0 || (lotsize + PositionsVolume()) < volume_limit)
Thanks, your suggestion and code works locally but, when I submit the program again I get the exact same error. I even had to add the limit volume condition just in case, the error was coming from that angle.
Then;
I keep on getting the same error, still.
I have been working on an update on one of my programs, The code to validate lot size seems fine mostly as I have used it in several programs in the market. Error:
Code:However, this time when I submit it the program to the market, the validation fails due to invalid volume. Can anyone spot an issue
I have been getting this too even with all the checks. Something is up with the validator

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have been working on an update on one of my programs, The code to validate lot size seems fine mostly as I have used it in several programs in the market. Error:
Code:However, this time when I submit it the program to the market, the validation fails due to invalid volume. Can anyone spot an issue