- Instant notifications and alerts in MetaTrader 5
- How to Use - MQL5 Cloud Network
- Additional Features - Price Charts, Technical and Fundamental Analysis
you can code this within the EA itself, no need to make an indicator
input int number_of_days = 3; // number of days to check int seconds_in_a_day = 1440 * 60; int time_threshold = number_of_days * seconds_in_a_day; datetime open_time = OrderOpenTime(); // The time when the order was opened datetime current_time = TimeCurrent(); int time_of_position = current_time - open_time; if((long)time_of_position > time_threshold) { //Alert }
Thanks Conor, I don't have the script for the EA. It's a market purchase. Not sure how I would incorporate it. Would this exclude weekends?
change TimeCurrent() to TimeTradeServer() and then you can be confident that it excludes weekends if the market is closed at the weekend.
Awesome, thanks Conor. I'll give it a whirl.
- johnsbf #: Hi Conor, I get a bunch of errors but that's probably because I'm clueless. I intend to learn how to code though.
Don't post pictures of code, they are generally too hard to read.
Please edit your (original) post and use the CODE button (or Alt+S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum #25 (2019)
Messages Editor
Forum rules and recommendations - General - MQL5 programming forum (2023) -
Put your inputs outside of functions.
- Conor Mcnamara #: change TimeCurrent() to TimeTradeServer() and then you can be confident that it excludes weekends if the market is closed at the weekend.
There is no TimeTradeServer on MT4.
- Using either will not exclude weekends. There are no bars when market is closed.
// Remember to select the order. datetime open_time = OrderOpenTime(); int open_bars = iBarShift(_Symbol, _Period, open_Time); int time_of_position = open_Bars * PeriodSeconds();
William Roeder #:
// Remember to select the order. datetime open_time = OrderOpenTime(); int open_bars = iBarShift(_Symbol, _Period, open_Time); int time_of_position = open_Bars * PeriodSeconds();
that looks like a good clever solution
you have to look up the manual to know how to make an alert
https://docs.mql4.com/common/alert
but if you're taking this seriously, you will need to learn how to make a loop that performs analysis on the active orders. I don't build EAs in MT4 so I know very little on that, I know a lot more about mql5
something like that:
// Globals input int number_of_days = 3; // Number of days to check int seconds_in_a_day = 1440 * 60; // Seconds in a day int time_threshold = number_of_days * seconds_in_a_day; void OnTick() { if (OrdersTotal() > 0) { for (int i = 0; i < OrdersTotal(); i++) { if (OrderSelect(i, SELECT_BY_POS)) { datetime open_time = OrderOpenTime(); int open_bars = iBarShift(_Symbol, _Period, open_time); int time_of_position = open_bars * PeriodSeconds(); if (time_of_position > time_threshold) { double currentProfit = OrderProfit(); string alert_message = "Position has been open for more than " + IntegerToString(time_of_position) + " seconds. Current profit: " + DoubleToString(currentProfit, 2) + " GBP"; Alert(alert_message); SendNotification(alert_message); } } } } }
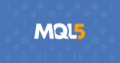
that looks like a good clever solution
you have to look up the manual to know how to make an alert
https://docs.mql4.com/common/alert
but if you're taking this seriously, you will need to learn how to make a loop that performs analysis on the active orders. I don't build EAs in MT4 so I know very little on that, I know a lot more about mql5
something like that:
Awesome, thanks.
It works great except I get alerts every couple of seconds.
Is there a way to only have it only check every 12 or 24 hours or something like that?
try this:
// Globals input int number_of_days = 3; // Number of days to check int seconds_in_a_day = 1440 * 60; // Seconds in a day int time_threshold = number_of_days * seconds_in_a_day; int alerted_positions[]; void OnTick() { if (OrdersTotal() > 0) { for (int i = 0; i < OrdersTotal(); i++) { if (OrderSelect(i, SELECT_BY_POS)) { int ticket = OrderTicket(); // Get the unique ticket ID datetime open_time = OrderOpenTime(); int open_bars = iBarShift(_Symbol, _Period, open_time); int time_of_position = open_bars * PeriodSeconds(); if (time_of_position >= time_threshold && !IsPositionAlerted(ticket)) { double currentProfit = OrderProfit(); string alert_message = "Position has been open for more than " + IntegerToString(time_of_position) + " seconds. Current profit: " + DoubleToString(currentProfit, 2) + " GBP"; Alert(alert_message); SendNotification(alert_message); ArrayResize(alerted_positions, ArraySize(alerted_positions) + 1); alerted_positions[ArraySize(alerted_positions) - 1] = ticket; } } } } } bool IsPositionAlerted(int ticket) { if (ArraySize(alerted_positions) == 0) { return false; // no positions alerted yet } else{ for (int i = 0; i < ArraySize(alerted_positions); i++) { if (alerted_positions[i] == ticket) { return true; } } } return false; }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use