When you post code please use the Code button (Alt+S) !
...
Improperly formatted code removed by moderator. Please EDIT your post and use the CODE button (Alt-S) when inserting code.
Hover your mouse over your post and select "edit" ...
...
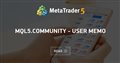
MQL5.community - User Memo
- www.mql5.com
You have just registered and most likely you have questions such as, "How do I insert a picture to my a message?" "How do I format my MQL5 source code?" "Where are my personal messages kept?" You may have many other questions. In this article, we have prepared some hands-on tips that will help you get accustomed in MQL5.community and take full advantage of its available features.
Junior Vidal:
This code is using mql4 syntax and you are trying to compile it with MT5 (mql5).
I am not able to fix these errors:
'ask' - undeclared identifier line:48
'ask' - undeclared identifier line:50
'OP_BUY' undeclared identifier line:53
'Ordersed'- wrong parameters count line:53
built-in: bool OrderSend(const MqlTradeRequest&,Mqltraderresult&) line:53

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I am not able to fix these errors: