Yes ... Documentation on MQL5: Language Basics / Operations and Expressions / Bitwise Operations
Right Shift
The binary representation of x is shifted to the right by y digits. If the value to shift is of the unsigned type, the logical right shift is made, i.e. the freed left-side bits will be filled with zeroes.
If the value to shift is of a sign type, the arithmetic right shift is made, i.e. the freed left-side digits will be filled with the value of a sign bit (if the number is positive, the value of the sign bit is 0; if the number is negative, the value of the sign bit is 1).
x = x >> y;Left Shift
The binary representation of x is shifted to the left by y digits, the freed right-side digits are filled with zeros.
x = x << y;
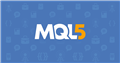
- www.mql5.com
Fernando Carreiro #:
Yes ... Documentation on MQL5: Language Basics / Operations and Expressions / Bitwise Operations
Right Shift
The binary representation of x is shifted to the right by y digits. If the value to shift is of the unsigned type, the logical right shift is made, i.e. the freed left-side bits will be filled with zeroes.
If the value to shift is of a sign type, the arithmetic right shift is made, i.e. the freed left-side digits will be filled with the value of a sign bit (if the number is positive, the value of the sign bit is 0; if the number is negative, the value of the sign bit is 1).
Left Shift
The binary representation of x is shifted to the left by y digits, the freed right-side digits are filled with zeros.
thanks.
I did not explain this properly ,sorry .I am forced to use an int or long as typescript has the type "number" (a long judging by the outcome in typescript/javascript)
also , off topic , shouldn't (-32)>>7 be zero ?
also , off topic , shouldn't (-32)>>7 be zero ?
It is best to shift unsigned integers, or else it will seem a little confusing.
-32 = 0xFFFFFFE0 (11111111 11111111 11111111 11100000)
shifting it 4 bits to right, makes it 268435454 = 0x0FFFFFFE (00001111 11111111 11111111 11111110)
shifting it 7 bits to right, makes it 33554431 = 0x01FFFFFF (00000001 11111111 11111111 11111111)
EDIT: I see what you mean. According to the documentation is should be filling in the left bit with the sign bit and not zero.
Show your code, as there might be a typecast into an unsigned which is causing the issue.
Hi , in javascript theres >>> and its called an "unsigned right shift"
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Unsigned_right_shift
Is >> the equivalent in mql5 ?
Thanks
There is no unsigned right shift in mql5. You need to be creative.
// Not compiled, not tested. union toShift { int vsigned; uint vnotsigned; }; void Start() { toShift i; i.vsigned = -32; Print(i.vnotsigned>>7); }
It is best to shift unsigned integers, or else it will seem a little confusing.
-32 = 0xFFFFFFE0 (11111111 11111111 11111111 11100000)
shifting it 4 bits to right, makes it 268435454 = 0x0FFFFFFE (00001111 11111111 11111111 11111110)
shifting it 7 bits to right, makes it 33554431 = 0x01FFFFFF (00000001 11111111 11111111 11111111)
EDIT: I see what you mean. According to the documentation is should be filling in the left bit with the sign bit and not zero.
Show your code, as there might be a typecast into an unsigned which is causing the issue.
it does it for the unsigned types but not for the signed i think . Thanks
int a=-32; for(int i=0;i<8;i++){ int sh=a>>i; Print(IntegerToString(a)+" >> "+IntegerToString(i)+" = "+IntegerToString(sh)); }
thanks will test it
----
it almost did it
// Not compiled, not tested. union toShift { int vsigned; uint vnotsigned; }; toShift x; x.vsigned=-32; for(int i=0;i<8;i++){ int sh=x.vnotsigned>>i; Print(IntegerToString(x.vsigned)+" >> "+IntegerToString(i)+" = "+IntegerToString(sh)); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi , in javascript theres >>> and its called an "unsigned right shift"
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Unsigned_right_shift
Is >> the equivalent in mql5 ?
Thanks