Connect to Meta trader server using c# and console application in order to place orders automatically
When you post code please use the Code button (Alt+S) !
Please EDIT your post and use the CODE button (Alt-S) when inserting code.
Hover your mouse over your post and select "edit" ...
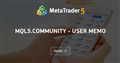
MQL5.community - User Memo
- www.mql5.com
You have just registered and most likely you have questions such as, "How do I insert a picture to my a message?" "How do I format my MQL5 source code?" "Where are my personal messages kept?" You may have many other questions. In this article, we have prepared some hands-on tips that will help you get accustomed in MQL5.community and take full advantage of its available features.
You have shown C# code but what does that have to do with MQL and MetaTrader?
MetaQuotes trading servers use a propriety protocol and it's not possible to connect directly.
You have to go via MetaTrader but MetaTrader 5 does not offer any API for C#, only Python.
If you want to have MetaTrader receive instructions from an external source, then you will have to create a MQL program to do so.
ionel stefanuca #:
Hi Fernando,
Thanks for your message. You have a project example for this to share with us? :)
Regards,
Ionel
Hi Fernando,
Thanks for your message. You have a project example for this to share with us? :)
Regards,
Ionel
There are plenty of example using C# with MQL on this site. Please do a search before asking.
You need to go through MT5 Terminal, you can't connect directly to an MT5 server.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I want to send to meta trader orders automatically from an external API. For example we receive semnals from a API and we want to place orders automatically in metatrader4/5.
Is there a way to do this?
We think to code an personal application using c# ( console application) and connect to meta trader broker server using TCP/IP but we are not able to connect to the broker server.
We are receiving this error message:
Eroare: A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond 188.40.223.58:433
My code is: