while (RefreshRates() == false) { }
That loop only exits when a new tick arrives. Delete it.
My EA works only when I open MT$ afresh and the runs once.
It does not close the trade it opens.
When I try to run it again, it does nothing.
When I try to remove it from the chart, it freezes for a while before leaving the chart.
Here is a the full code (82 lines of code).
I will appreciate any help that resolves this challenge.
#property copyright "Copyright 2023, MetaQuotes Ltd." #property link "https://www.mql5.com" #property version "1.00" #property strict //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ // Define the input parameters extern int ADX_Period = 14; extern int ADX_Level = 20; // Define the global variables double adx_plus; double adx_minus; double adx_signal; int chartPeriod = Period(); string chartName = Symbol(); // Define the start function void OnTick() { // Loop forever while (true) { // Calculate ADX values adx_plus = iADX(chartName, chartPeriod, ADX_Period, PRICE_CLOSE, MODE_PLUSDI, 0); adx_minus = iADX(chartName, chartPeriod, ADX_Period, PRICE_CLOSE, MODE_MINUSDI, 0); adx_signal = iADX(chartName, chartPeriod, ADX_Period, PRICE_CLOSE, MODE_MAIN, 0); // Check for buy signal if (adx_plus > ADX_Level && adx_signal > ADX_Level && adx_minus < ADX_Level) { // Check if there is no open buy order if (OrderType() != OP_BUY) { // Place a buy order int ticket = OrderSend(Symbol(), OP_BUY, 0.1, Ask, 3, 0, 0, "ADX Buy", 0, 0, Green); } } // Check for sell signal if (adx_minus > ADX_Level && adx_signal > ADX_Level && adx_plus < ADX_Level) { // Check if there is no open sell order if (OrderType() != OP_SELL) { // Place a sell order int ticket = OrderSend(Symbol(), OP_SELL, 0.1, Bid, 3, 0, 0, "ADX Sell", 0, 0, Red); } } // Check for open buy order if (OrderType() == OP_BUY) { // Close buy order if adx_plus goes below 20 or adx_minus goes above 20 if (adx_plus < ADX_Level || adx_minus > ADX_Level) { OrderClose(OrderTicket(), OrderLots(), Bid, 3, Green); } } // Check for open sell order if (OrderType() == OP_SELL) { // Close sell order if adx_minus goes below 20 or adx_plus goes above 20 if (adx_minus < ADX_Level || adx_plus > ADX_Level) { OrderClose(OrderTicket(), OrderLots(), Ask, 3, Red); } } // Sleep for 1 second Sleep(1000); } }
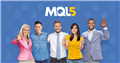
- 2023.04.07
- www.mql5.com
You have been warned about this before on other posts.
Please EDIT your post and use the CODE button when you post code.
I will repeat ... edit your post.
Forum on trading, automated trading systems and testing trading strategies
EA freezes at close of Parameter window
Fernando Carreiro, 2023.04.07 14:58
You have been warned about this before on other posts.
Please EDIT your post and use the CODE button when you post code.
To make things more clear for you, as William has pointed out, remove these parts of your code ...
// Loop forever while (true) {
// Sleep for 1 second Sleep(1000); }
OnTick, is an event handler. It should not be blocked. It needs to carry out its task and return as quickly as possible so that it can handle the next tick event.
The function is called in EAs when the NewTick event occurs to handle a new quote

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Also, the EA works through 30 open charts and I have the following in two blocks of code within the EA:
It says that the EA should be making efforts to refresh rates untill it is successful.
Note that I have also tried preventing new incoming ticks at the OnTick() function prior to the close of the parameter window but the issue continued.