I should mention the iMA_On_Array function I got from here:
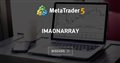
iMAOnArray
- 2015.04.24
- www.mql5.com
Could you suggest function or technique similar to iMAOnArray in MQL5...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Trying to translate ThinkOrSwim's Relative Volatility Index indicator to MQL5, and I'm close (I think), but it's not plotting the same as in ToS. I found this indicator already written in MQL5, however whenever I utilize it in optimization of a trading strategy, it runs extremely slow, so I want to trim it down and make it myself to run as fast as it should.
The applicable code in ThinkScript looks like this:
We start by finding the trailing 10 periods of STDDEV of the high and low. We then find their respective moving average values of either 0 or stDevHi/stDevLo based on the shown price conditions. We then check if their respective sums are == 0, if so the rviHi/rviLo would be 50 for that bar, otherwise you see the formula for the value it should be. Finally, you calculate the current bar's average between rviHi and rviLo. This RVI is the line I'd like to plot.
The code I have and some screenshots of the current output are below. The code is well commented, I'm just not very skilled in MQL5 yet to figure out why this doesn't work just yet. Hoping someone can shed some light on it.
This is what it SHOULD look like from ToS chart:
And this is the current output of the code I've shown: