hello, i've been facing issues on how to read file and convert it into usable data for calculation. i've read and convert the file into integer but when i'm trying to place it in a array and try to do alert function it does not show anything. the code below is working properly as intended but i don't know if this code is the most efficient way to do this logic and when i try to change the highlighted code into array it does not work? any thoughts?
I tried your code - it was crashing as the numberofparameters was larger than the arraysize.
Easily fixed (although this does assume both arrays are the same size always):
for(int x = 0; x<ArraySize(aroonperiod); x++) { aroonperiodvalue = StringToInteger(aroonperiod[x]); aroonstoplossvalue =StringToDouble(aroonstoploss[x]); PrintFormat("%d aroonperiodvalue=%.2f aroonstoplossvalue=%.2f ", x, aroonperiodvalue, aroonstoplossvalue); }
(I also changed the Alerts to Print for my convenience)
In terms of efficiency, do you mean less code, faster runtime? What are you looking for exactly?
One thought is that if you are going to accessing this data thousands of times, one (minor) optimisation could be to convert them into integers and doubles so you don't need to keep calling StringToInteger and StringToDouble every time.
The runtime is already around 1000 microsecs so its not unreasonably slow - output below:
2022.06.09 15:54:15.774 === Start === 2022.06.09 15:54:15.774 ArraySize=56 numberofparameters=137 2022.06.09 15:54:15.774 [000] aroonperiodvalue=299.00 aroonstoplossvalue=15.00 2022.06.09 15:54:15.774 [001] aroonperiodvalue=159.00 aroonstoplossvalue=14.90 2022.06.09 15:54:15.774 [002] aroonperiodvalue=154.00 aroonstoplossvalue=14.80 2022.06.09 15:54:15.774 [003] aroonperiodvalue=156.00 aroonstoplossvalue=14.70 2022.06.09 15:54:15.774 [004] aroonperiodvalue=163.00 aroonstoplossvalue=14.60 2022.06.09 15:54:15.774 [005] aroonperiodvalue=283.00 aroonstoplossvalue=14.50 2022.06.09 15:54:15.774 [006] aroonperiodvalue=60.00 aroonstoplossvalue=14.40 2022.06.09 15:54:15.774 [007] aroonperiodvalue=114.00 aroonstoplossvalue=14.30 2022.06.09 15:54:15.774 [008] aroonperiodvalue=110.00 aroonstoplossvalue=14.20 2022.06.09 15:54:15.774 [009] aroonperiodvalue=208.00 aroonstoplossvalue=14.10 2022.06.09 15:54:15.774 [010] aroonperiodvalue=119.00 aroonstoplossvalue=14.00 2022.06.09 15:54:15.774 [011] aroonperiodvalue=152.00 aroonstoplossvalue=13.90 2022.06.09 15:54:15.774 [012] aroonperiodvalue=155.00 aroonstoplossvalue=13.80 2022.06.09 15:54:15.774 [013] aroonperiodvalue=171.00 aroonstoplossvalue=13.70 2022.06.09 15:54:15.774 [014] aroonperiodvalue=284.00 aroonstoplossvalue=13.60 2022.06.09 15:54:15.774 [015] aroonperiodvalue=207.00 aroonstoplossvalue=13.50 2022.06.09 15:54:15.774 [016] aroonperiodvalue=153.00 aroonstoplossvalue=13.40 2022.06.09 15:54:15.774 [017] aroonperiodvalue=61.00 aroonstoplossvalue=13.30 2022.06.09 15:54:15.774 [018] aroonperiodvalue=166.00 aroonstoplossvalue=13.20 2022.06.09 15:54:15.774 [019] aroonperiodvalue=298.00 aroonstoplossvalue=13.10 2022.06.09 15:54:15.774 [020] aroonperiodvalue=96.00 aroonstoplossvalue=13.00 2022.06.09 15:54:15.774 [021] aroonperiodvalue=175.00 aroonstoplossvalue=12.90 2022.06.09 15:54:15.774 [022] aroonperiodvalue=173.00 aroonstoplossvalue=12.80 2022.06.09 15:54:15.774 [023] aroonperiodvalue=206.00 aroonstoplossvalue=12.70 2022.06.09 15:54:15.774 [024] aroonperiodvalue=178.00 aroonstoplossvalue=12.60 2022.06.09 15:54:15.774 [025] aroonperiodvalue=201.00 aroonstoplossvalue=12.50 2022.06.09 15:54:15.774 [026] aroonperiodvalue=133.00 aroonstoplossvalue=12.40 2022.06.09 15:54:15.774 [027] aroonperiodvalue=106.00 aroonstoplossvalue=12.30 2022.06.09 15:54:15.774 [028] aroonperiodvalue=124.00 aroonstoplossvalue=12.20 2022.06.09 15:54:15.774 [029] aroonperiodvalue=132.00 aroonstoplossvalue=12.10 2022.06.09 15:54:15.774 [030] aroonperiodvalue=217.00 aroonstoplossvalue=12.00 2022.06.09 15:54:15.774 [031] aroonperiodvalue=98.00 aroonstoplossvalue=11.90 2022.06.09 15:54:15.775 [032] aroonperiodvalue=138.00 aroonstoplossvalue=11.80 2022.06.09 15:54:15.775 [033] aroonperiodvalue=174.00 aroonstoplossvalue=11.70 2022.06.09 15:54:15.775 [034] aroonperiodvalue=221.00 aroonstoplossvalue=11.60 2022.06.09 15:54:15.775 [035] aroonperiodvalue=75.00 aroonstoplossvalue=11.50 2022.06.09 15:54:15.775 [036] aroonperiodvalue=172.00 aroonstoplossvalue=11.40 2022.06.09 15:54:15.775 [037] aroonperiodvalue=246.00 aroonstoplossvalue=11.30 2022.06.09 15:54:15.775 [038] aroonperiodvalue=167.00 aroonstoplossvalue=11.20 2022.06.09 15:54:15.775 [039] aroonperiodvalue=147.00 aroonstoplossvalue=11.10 2022.06.09 15:54:15.775 [040] aroonperiodvalue=79.00 aroonstoplossvalue=11.00 2022.06.09 15:54:15.775 [041] aroonperiodvalue=186.00 aroonstoplossvalue=10.90 2022.06.09 15:54:15.775 [042] aroonperiodvalue=41.00 aroonstoplossvalue=10.80 2022.06.09 15:54:15.775 [043] aroonperiodvalue=161.00 aroonstoplossvalue=10.70 2022.06.09 15:54:15.775 [044] aroonperiodvalue=42.00 aroonstoplossvalue=10.60 2022.06.09 15:54:15.775 [045] aroonperiodvalue=162.00 aroonstoplossvalue=10.50 2022.06.09 15:54:15.775 [046] aroonperiodvalue=146.00 aroonstoplossvalue=10.40 2022.06.09 15:54:15.775 [047] aroonperiodvalue=181.00 aroonstoplossvalue=10.30 2022.06.09 15:54:15.775 [048] aroonperiodvalue=180.00 aroonstoplossvalue=10.20 2022.06.09 15:54:15.775 [049] aroonperiodvalue=273.00 aroonstoplossvalue=10.10 2022.06.09 15:54:15.775 [050] aroonperiodvalue=183.00 aroonstoplossvalue=10.00 2022.06.09 15:54:15.775 [051] aroonperiodvalue=250.00 aroonstoplossvalue=9.90 2022.06.09 15:54:15.775 [052] aroonperiodvalue=278.00 aroonstoplossvalue=9.80 2022.06.09 15:54:15.775 [053] aroonperiodvalue=105.00 aroonstoplossvalue=9.70 2022.06.09 15:54:15.775 [054] aroonperiodvalue=225.00 aroonstoplossvalue=9.60 2022.06.09 15:54:15.775 [055] aroonperiodvalue=282.00 aroonstoplossvalue=9.50 2022.06.09 15:54:15.775 Runtime=1006 microSecs 2022.06.09 15:54:15.775 === End ===
I tried your code - it was crashing as the numberofparameters was larger than the arraysize.
Thanks for the reply R4tna C #:the numberofparameters was the first total size of the array before I split it into to 2 :aroonperiod and aroonstoploss, since both of them have different sizes that's why I use stringsplit() and arraycopy() to seperate them in to there separate arrays so that I can calculate them individually. theoretically if I try to add more data on the text file I don't need to change the for loop although that is my thought about it. maybe it makes the script slow. what I'm trying to achieve is that once I convert the string into numbers. I need to place them in to array so that I can calculate them to get the results that I want. the aroonperiod[] and arronstoploss[] are string type variable, I can't use it to calculate. that's why I tried to use stringtointeger() and stringtodouble() to convert it into a number but the problem is that I cannot hold them into array for some reason. the code below is my attempt to place the number into array but for some reason, the result does not show up once I run my script maybe it is because of the alert() function or something else. any thoughts?
for(int x = 0; x<=numberofparameters; x++) { aroonperiodarray[x] = StringToInteger(aroonperiod[x]); Alert(aroonperiodarray[x]); aroonstoplossarray[x] =StringToDouble(aroonstoploss[x]); Alert(aroonstoplossarray[x]); }
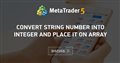
- 2022.06.09
- www.mql5.com
Thanks for the reply R4tna C #:the numberofparameters was the first total size of the array before I split it into to 2 :aroonperiod and aroonstoploss, since both of them have different sizes that's why I use stringsplit() and arraycopy() to seperate them in to there separate arrays so that I can calculate them individually. theoretically if I try to add more data on the text file I don't need to change the for loop although that is my thought about it. maybe it makes the script slow. what I'm trying to achieve is that once I convert the string into numbers. I need to place them in to array so that I can calculate them to get the results that I want. the aroonperiod[] and arronstoploss[] are string type variable, I can't use it to calculate. that's why I tried to use stringtointeger() and stringtodouble() to convert it into a number but the problem is that I cannot hold them into array for some reason. the code below is my attempt to place the number into array but for some reason, the result does not show up once I run my script maybe it is because of the alert() function or something else. any thoughts?
I don't use the alert function much so can't say, but print is a very easy way to get the info out.
Your aroon arrays were working fine according to my tests but they hold strings - you could easily create 2 new arrays (one for int and one for double) and use the loop to convert the data from string to numeric form. You just have to do the conversion once - so that is an optimization.
I note you have defined some non-string arrays:
static long aroonperiodarray[]; static double aroonstoplossarray[];
Are these the ones you want to populate?
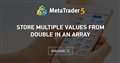
- 2022.06.02
- www.mql5.com
I don't use the alert function much so can't say, but print is a very easy way to get the info out.
Your aroon arrays were working fine according to my tests but they hold strings - you could easily create 2 new arrays (one for int and one for double) and use the loop to convert the data from string to numeric form. You just have to do the conversion once - so that is an optimization.
I note you have defined some non-string arrays:
Are these the ones you want to populate?
Assuming these are the ones, here is the code to do it:
void OnStart() { ulong timer = GetMicrosecondCount(); string TestFileName="Excellent.txt"; string filename=TestFileName; ReadFileToPrintCSV2(filename); PrintFormat("ArraySize(aroonperiod)=%d ArraySize(aroonstoploss)=%d numberofparameters=%d", ArraySize(aroonperiod), ArraySize(aroonstoploss), numberofparameters); ArrayResize(aroonperiodarray, ArraySize(aroonperiod)); ArrayResize(aroonstoplossarray, ArraySize(aroonstoploss)); ZeroMemory(aroonperiodarray); ZeroMemory(aroonstoplossarray); for(int x = 0; x<ArraySize(aroonperiod); x++) { aroonperiodarray[x] = StringToInteger(aroonperiod[x]); } Print("aroonperiodarray"); ArrayPrint(aroonperiodarray); for(int x = 0; x<ArraySize(aroonstoploss); x++) { aroonstoplossarray[x] = StringToDouble(aroonstoploss[x]); } Print("aroonstoplossarray"); ArrayPrint(aroonstoplossarray); // for(int x = 0; x<ArraySize(aroonperiod); x++) // { // // aroonperiodvalue = StringToInteger(aroonperiod[x]); // // aroonstoplossvalue =StringToDouble(aroonstoploss[x]); // // PrintFormat("[%03d] aroonperiodvalue=%.2f aroonstoplossvalue=%.2f ", x, aroonperiodvalue, aroonstoplossvalue); // } timer = GetMicrosecondCount()-timer; PrintFormat("Runtime=%d microSecs\n=== End ===", timer); }
Thanks for the reply again R4tna C #: I don't really use scripts when I'm implementing ideas for my system rather I use EA to see errors but now I see the error that I didn't saw when I was making this. the array size of aroonperiodarray[] and aroonstoplossarray[] was the main issue on why it keeps giving me error from the start because it does not properly size or no size at all. splitting for loop, for different array was a great idea since my goal is to separate them on array I don't need the numberofparameters variable to run both of them. I tried if the array is now a number so i try to sum random numbers from the array and it works. Thank you so much R4tna C #:. now i can try this idea to my system and made adjustment to make it faster. Thanks again!!
ArrayResize(aroonperiodarray, ArraySize(aroonperiod)); ArrayResize(aroonstoplossarray, ArraySize(aroonstoploss)); ZeroMemory(aroonperiodarray); ZeroMemory(aroonstoplossarray); for(int x = 0; x<ArraySize(aroonperiod); x++) { aroonperiodarray[x] = StringToInteger(aroonperiod[x]); } long sumaroonperiod = aroonperiodarray[1]+aroonperiodarray[3]; Print(sumaroonperiod); //ArrayPrint(aroonperiodarray); for(int x = 0; x<ArraySize(aroonstoploss); x++) { aroonstoplossarray[x] = StringToDouble(aroonstoploss[x]); } double sumaroonstoploss = aroonstoplossarray[5]+aroonstoplossarray[5]; Print(sumaroonstoploss); //ArrayPrint(aroonstoplossarray); for(int x = 0; x<ArraySize(aroonperiod); x++) { aroonperiodvalue = StringToInteger(aroonperiod[x]); aroonstoplossvalue =StringToDouble(aroonstoploss[x]); PrintFormat("[%03d] aroonperiodvalue=%.2f aroonstoplossvalue=%.2f ", x, aroonperiodvalue, aroonstoplossvalue); } timer = GetMicrosecondCount()-timer; PrintFormat("Runtime=%d microSecs\n=== End ===", timer);
Thanks for the reply again R4tna C #: I don't really use scripts when I'm implementing ideas for my system rather I use EA to see errors but now I see the error that I didn't saw when I was making this. the array size of aroonperiodarray[] and aroonstoplossarray[] was the main issue on why it keeps giving me error from the start because it does not properly size or no size at all. splitting for loop, for different array was a great idea since my goal is to separate them on array I don't need the numberofparameters variable to run both of them. I tried if the array is now a number so i try to sum random numbers from the array and it works. Thank you so much R4tna C #:. now i can try this idea to my system and made adjustment to make it faster. Thanks again!!
Good - glad this helped.
I often use scripts to test ideas in isolation - its a great way of ironing out issues before you alter the main EA code base

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
hello, i've been facing issues on how to read file and convert it into usable data for calculation. i've read and convert the file into integer but when i'm trying to place it in a array and try to do alert function it does not show anything. the code below is working properly as intended but i don't know if this code is the most efficient way to do this logic and when i try to change the highlighted code into array it does not work? any thoughts?