Hello everyone,
this is my first post on the forum; since I am quite new to mql4 programming I would be grateful if somebody could help with the following peace of code:
for(int i=0; i<=limit; i++)
{
Avg1[i]=iMA(NULL,0,4,0,MODE_LWMA,PRICE_CLOSE,i);
Avg2[i]=iMA(NULL,0,12,0,MODE_LWMA,PRICE_CLOSE,i);
Mom[i]=(Avg2[i]-Avg1[i])/Avg1[i]*100;
DeltaMom[i]=Mom[i]-Mom[i+1];
}
This will return a Zero Divide error.
therefore I changed the code to:
for(int i=0; i<=limit; i++)
{
Avg1[i]=iMA(NULL,0,4,0,MODE_LWMA,PRICE_CLOSE,i);
Avg2[i]=iMA(NULL,0,12,0,MODE_LWMA,PRICE_CLOSE,i);
if(Avg1[i]==0)
Mom[i]=100;
if(Avg1[i]!=0)
Mom[i]=(Avg2[i]-Avg1[i])/Avg1[i]*100;
DeltaMom[i]=Mom[i]-Mom[i+1];
}
Now this assigns the value 100 to the variable Mom on the first iteration of the loop and does not get updated anymore, hence Mom is plotted as a straight line .
Can anybody tell me what am I doing wrong? Thank you.
2 things :
- Primo, a moving average with a 0 (a MathIsValidNumber(value) returning false) value isn't valid - you could skip it directly or be more strict on the bars counter - I don't remind exactly but it seems to me that there's an IndicatorCounted() and a "Bars" in MQL4
- Secondo, you ask for Mom[i+1] without calculating Avg1[i+1] & Avg2[i+1]
Use the code (toolbar or Alt+S) for more clarity
for(int i=0; i<=limit; i++) { Avg1[i]=iMA(NULL,0,4,0,MODE_LWMA,PRICE_CLOSE,i); Avg2[i]=iMA(NULL,0,12,0,MODE_LWMA,PRICE_CLOSE,i); Mom[i]=(Avg2[i]-Avg1[i])/Avg1[i]*100; DeltaMom[i]=Mom[i]-Mom[i+1]; }
- Please edit your (original) post and use the CODE
button (Alt-S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor -
for(int i=0; i<=limit; i++){ … DeltaMom[i]=Mom[i]-Mom[i+1];
You are accessing i+1 element before you calculate it. Count down. -
Avg2[i]=iMA(NULL,0,12,0,MODE_LWMA,PRICE_CLOSE,i);
Post all the relevant context. We have no idea what limit is. Your maximum lookback is 12 for the MA and 13 for mom[i+1]. See How to do your lookbacks correctly.
Try This : (if you attempt it in MT5 make sure to control your ArraySerialisation)
//+------------------------------------------------------------------+ //| xaf_avg.mq4 | //| Copyright 2119, Republic Of Mars. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property strict #property indicator_separate_window #property indicator_buffers 4 #property indicator_plots 1 //--- plot Delta #property indicator_label1 "Delta" #property indicator_type1 DRAW_LINE #property indicator_color1 clrRed #property indicator_style1 STYLE_SOLID #property indicator_width1 1 input string notea="";//Average 1 --- input int AVGA_Period=4;//Period input ENUM_MA_METHOD AVGA_Method=MODE_LWMA;//Method input ENUM_APPLIED_PRICE AVGA_Price=PRICE_TYPICAL;//Price input string noteb="";//Average 2 --- input int AVGB_Period=12;//Period input ENUM_MA_METHOD AVGB_Method=MODE_LWMA;//Method input ENUM_APPLIED_PRICE AVGB_Price=PRICE_TYPICAL;//Price //--- indicator buffers double Delta[]; double Mom[],Avg1[],Avg2[]; int bar_reduction=0,oldest_bar=0; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping SetIndexBuffer(0,Delta); SetIndexBuffer(1,Mom); SetIndexStyle(1,DRAW_NONE,EMPTY,EMPTY,clrNONE); SetIndexBuffer(2,Avg1); SetIndexStyle(2,DRAW_NONE,EMPTY,EMPTY,clrNONE); SetIndexBuffer(3,Avg2); SetIndexStyle(3,DRAW_NONE,EMPTY,EMPTY,clrNONE); bar_reduction=MathMax(AVGA_Period,AVGB_Period)+1; oldest_bar=0; //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- int old_bar=1; int new_bar=1; if(prev_calculated==0) old_bar=rates_total-bar_reduction-1; if(prev_calculated!=0) old_bar=1; //Loop for(int i=old_bar;i>=new_bar;i--) { if(i>oldest_bar) oldest_bar=i; Avg1[i]=iMA(Symbol(),Period(),AVGA_Period,0,AVGA_Method,AVGA_Price,i); Avg2[i]=iMA(Symbol(),Period(),AVGB_Period,0,AVGB_Method,AVGB_Price,i); Mom[i]=(Avg1[i]-Avg2[i])/(Avg1[i]*100); if(i==oldest_bar) Delta[i]=0; if(i<oldest_bar) Delta[i]=Mom[i]-Mom[i+1]; } //Loop Ends Here //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- } //+------------------------------------------------------------------+
2 things :
- Primo, a moving average with a 0 (a MathIsValidNumber(value) returning false) value isn't valid - you could skip it directly or be more strict on the bars counter - I don't remind exactly but it seems to me that there's an IndicatorCounted() and a "Bars" in MQL4
- Secondo, you ask for Mom[i+1] without calculating Avg1[i+1] & Avg2[i+1]
Use the code (toolbar or Alt+S) for more clarity
Thank you for your help Icham, will try and devise a solution based on your comments.
Thank you.
Welcome to mql5 Xaf ,
Try This : (if you attempt it in MT5 make sure to control your ArraySerialisation)
Thank you Lorenzos,
I'll study it carefully, very grateful.
- Please edit your (original) post and use the CODE
button (Alt-S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor - You are accessing i+1 element before you calculate it. Count down.
- Post all the relevant context. We have no idea what limit is. Your maximum lookback is 12 for the MA and 13 for mom[i+1]. See How to do your lookbacks correctly.
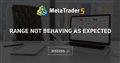

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello everyone,
this is my first post on the forum; since I am quite new to mql4 programming I would be grateful if somebody could help with the following peace of code:
This will return a Zero Divide error.
therefore I changed the code to:
Now this assigns the value 100 to the variable Mom on the first iteration of the loop and does not get updated anymore, hence Mom is plotted as a straight line .
Can anybody tell me what am I doing wrong? Thank you.