-
When you post code please use the CODE button (Alt-S)!
(For large amounts of code, attach it.) Please edit your (original) post.
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor - Russfox: The first trade is identified by the OrderComment () "1" or "1[sl]".Not a good idea to use comments, brokers can change comments, including complete replacement.
- Russfox:vthen count the profit of the trades (tradeprofit) forward from this time.Do not assume history is ordered by date, it's not.
Could EA Really Live By Order_History Alone? (ubzen) - MQL4 and MetaTrader 4 - MQL4 programming forum
Count how many lost orders from the last profit order - MQL4 and MetaTrader 4 - MQL4 programming forum - Russfox:At the start of a martingale string of trades,Martingale, guaranteed to blow your account eventually.
//+------------------------------------------------------------------+ void CheckForSigna11() { if(TotalOpenOrders()==0) if(OrderSelect(lastTradeTicket(),MODE_HISTORY)){ if(OrderType()==OP_BUY) if((OrderProfit()>0)&& (countprofit()>=0)) EnterTrade(OP_BUY);} if(TotalOpenOrders()==0) if(OrderSelect(lastTradeTicket(),MODE_HISTORY)){ if(OrderType()==OP_BUY) if((OrderProfit()<0) && (tradeNumber <MaximumTrades)) EnterOppositeTrade((OP_SELL),OrderLots(),OrderComment());} if(TotalOpenOrders()==0) if(OrderSelect(lastTradeTicket(),MODE_HISTORY)){ if(OrderType()==OP_SELL) if((OrderProfit()>0)&& (countprofit()>=0)) EnterTrade(OP_SELL);} if(TotalOpenOrders()==0) if(OrderSelect(lastTradeTicket(),MODE_HISTORY)){ if(OrderType()==OP_SELL) if((OrderProfit()<0) && (tradeNumber <MaximumTrades)) EnterOppositeTrade((OP_BUY),OrderLots(),OrderComment());} if(TotalOpenOrders()== 0) {if(OrderSelect(lastTradeTicket(),MODE_HISTORY)) {if(OrderType()==OP_BUY) {if(( (tradeNumber >= MaximumTrades) && (countprofit()<0))) {EnterWinTrade1((OP_SELL),OrderLots(),OrderComment()); //if(TotalOpenOrders()<2) //if(OrderSelect(lastTradeTicket(),MODE_HISTORY)){ //if(OrderType()==OP_BUY) //if(( (tradeNumber >= MaximumTrades) && (countprofit()<0))) EnterWinTrade1((OP_BUY),OrderLots(),OrderComment());}} } //if(TotalOpenOrders()== 0) //if(OrderSelect(lastTradeTicket(),MODE_HISTORY)){ {if(OrderType()==OP_SELL) {if(((tradeNumber >= MaximumTrades) && (countprofit()<0))) {EnterWinTrade2((OP_BUY),OrderLots(),OrderComment()); //if(TotalOpenOrders()<2) //if(OrderSelect(lastTradeTicket(),MODE_HISTORY)){ //if(OrderType()==OP_SELL) //if(( (tradeNumber >= MaximumTrades) && (countprofit()<0))) EnterWinTrade2((OP_SELL),OrderLots(),OrderComment());}} } } } //+------------------------------------------------------------------+ //| Trade String Time Function | //+------------------------------------------------------------------+ datetime tradetime() { datetime tradetime=0; //tradeNumber = (int)comment; for(int j=0;j<OrdersHistoryTotal()-1;j++){ if(OrderSelect(j,SELECT_BY_POS,MODE_HISTORY)) {if(((OrderMagicNumber() == magic)) && ((OrderSymbol()==Symbol())) && ((OrderComment() == "1[sl]"))) //break; tradetime = OrderOpenTime(); //else Print("Failed to select order",GetLastError()); } Comment(ScreenComment+"\nTradetime; ",(string)tradetime,"\nOrderComment; ",(string)OrderComment()); } //Comment(ScreenComment+"\nThis is trade time: "+(string) OrderComment()+")"); return(tradetime); } //+------------------------------------------------------------------+ //| Trade String Profit/Loss Count Function | //+------------------------------------------------------------------+ double countprofit() { double profit=0; for(int j=0;j<OrdersHistoryTotal()-1;j++){ if(OrderSelect(j,SELECT_BY_POS,MODE_HISTORY)) {if(((OrderMagicNumber() == magic)) && ((OrderSymbol()==Symbol())) && (OrderOpenTime()>=tradetime())) break; {profit += OrderProfit(); Comment(ScreenComment+"\nThis is trade profit: "+(string)profit+""); } } //else Print("Failed to select order",GetLastError()); } //Comment(ScreenComment+"\nThis is trade number: "+(string)tradeNumber+"\nThe Lotsize is: "+DoubleToStr(lotsize,2)); //Print("OrderComment ",OrderComment()); Print("\nprofit ",profit); Print("\ntradetime ",tradetime()); return(profit); }
Ok, I've added the code in the format requested.
From what I've read on forums I understand that ordercomment may not be reliable. It has worked so far in the initial checkforsignal functions I've created.
If I couldn't get the ordecomment code to work I was considering using an array, which appears to be suggested in the link you provided. I don't have any experience with arrays, however I'll make an attempt and revert in future comments when I need further help...
I've been running some martingale code in the tester and demo account and have drawn the same conclusion. So I'm attempting to solve this with the above code.
Are there any good links for building arrays? I would want to add to the array, while counting the profit, then when the condition is met, empty the array of information and start again.
Hypothetically, if the ordercomment function did work, is the basic code ok?
Ok, I've added the code in the format requested.
From what I've read on forums I understand that ordercomment may not be reliable. It has worked so far in the initial checkforsignal functions I've created.
If I couldn't get the ordecomment code to work I was considering using an array, which appears to be suggested in the link you provided. I don't have any experience with arrays, however I'll make an attempt and revert in future comments when I need further help...
I've been running some martingale code in the tester and demo account and have drawn the same conclusion. So I'm attempting to solve this with the above code.
Are there any good links for building arrays? I would want to add to the array, while counting the profit, then when the condition is met, empty the array of information and start again.
Hypothetically, if the ordercomment function did work, is the basic code ok?
hi. i also have the same problem. Still looking for answer.
actually if we using order select by post mode history there are no guarantee the order is sequence as you may think. i already tried and also mention in here : https://docs.mql4.com/trading/orderselect
what i do is using select by ticket, but i still have difficulty to get the total by specific pair. so it can be attached in several pairs.
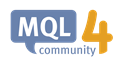
- docs.mql4.com
-
Do not assume history has only closed orders.
OrderType() == 6, 7 in the history pool? - MQL4 programming forum 2017.11.30 -
Do not assume history is ordered by date, it's not.
Could EA Really Live By Order_History Alone? (ubzen) - MQL4 programming forum 2012.04.21
Taking the last profit and storing it in a variable | MQL4 - MQL4 programming forum #3 2020.06.08 -
Total Profit is OrderProfit() + OrderSwap() + OrderCommission(). Some brokers don't use the Commission/Swap fields. Instead, they add balance entries. (Maybe related to Government required accounting/tax laws.)
"balance" orders in account history - Day Trading Techniques - MQL4 programming forum 2017.11.01Broker History FXCM Commission - <TICKET>
Rollover - <TICKET>>R/O - 1,000 EUR/USD @0.52 #<ticket> N/A OANDA Balance update
Financing (Swap: One entry for all open orders.)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I would like to count the profit of a varying number of closed trades.
At the start of a martingale string of trades, each trade is provided with a tradenumber in the OrderComment (). The trade number is increased by 1 after each loss until a win where the number is reset.
After a certain tradenumber I start to hedge the trades in an attempt to make a series of smaller profits to make up for the larger total loss. To stop the hedging once a total trade string profit is made I need to monitor the profit position of the trade string.
I want to achieve this by continuously calculating the profit of the closed trades back to tradenumber 1 (first trade in string). The first trade is identified by the OrderComment () "1" or "1[sl]".
My proposed method is to find the first trade and using the OpenOrderTime () (tradetime )and then count the profit of the trades (tradeprofit) forward from this time. I am using a for loop for both the tradetime and tradeprofit.
'I think' that the code works for the pre-hedging trades (i.e. single trades) but once it goes into the hedging trades the tradeprofit spirals out of control... Is there any one who can see where I'm going wrong?
I've included the relevant code below. First time user of forum so I may have not done this correctly. I have not included entering trade code etc... The trade numbers are added here.