surubabs:
What is Main, what is Signal ? CopyBuffer need 5 parameters.
Dear friend i am little confused how to coppy buffer of stochastic ?
Please correct this for me,,
Thanks in a advance
Suresh
angevoyageur:
What is Main, what is Signal ? CopyBuffer need 5 parameters.
What is Main, what is Signal ? CopyBuffer need 5 parameters.
//+------------------------------------------------------------------+ //| Stoc_Candle.mq5 | //| Copyright 2013, Suresh Kakkattil. | //| http://www.Rocktrader.in | //+------------------------------------------------------------------+ #property copyright "Copyright 2013, Suresh Kakkattil." #property link "http://www.Rocktrader.in" #property version "1.00" #include <Expert\MySignals\ACandlePatterns.mqh> //--- input parameters input double Lot=0.5; // Lots to trade input double Stoch_Max=80; input double Stoch_min=20; //--- global variables int Stoch; // double Main[],Signal[]; // dynamic arrays for numerical values of Bollinger Bands //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Do we have sufficient bars to work if(Bars(_Symbol,_Period)<60) // total number of bars is less than 60? { Alert("We have less than 60 bars on the chart, an Expert Advisor terminated!!"); return(-1); } //--- get handle of the Stochastic indicators Stoch=iStochastic(_Symbol,PERIOD_CURRENT,14,3,3,MODE_SMA,STO_LOWHIGH); //--- Check for Invalid Handle if(Stoch<0) { Alert("Error in creation of indicators - error: ",GetLastError(),"!!"); return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- release indicator handles IndicatorRelease(Stoch); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- we will use the static Old_Time variable to serve the bar time. //--- at each OnTick execution we will check the current bar time with the saved one. //--- if the bar time isn't equal to the saved time, it indicates that we have a new tick. static datetime Old_Time; datetime New_Time[1]; bool IsNewBar=false; //--- copying the last bar time to the element New_Time[0] int copied=CopyTime(_Symbol,_Period,0,1,New_Time); if(copied>0) // ok, the data has been copied successfully { if(Old_Time!=New_Time[0]) // if old time isn't equal to new bar time { IsNewBar=true; // if it isn't a first call, the new bar has appeared if(MQL5InfoInteger(MQL5_DEBUGGING)) Print("We have new bar here ",New_Time[0]," old time was ",Old_Time); Old_Time=New_Time[0]; // saving bar time } } else { Alert("Error in copying historical times data, error =",GetLastError()); ResetLastError(); return; } //--- EA should only check for new trade if we have a new bar if(IsNewBar==false) { return; } //--- do we have enough bars to work with int Mybars=Bars(_Symbol,_Period); if(Mybars<60) // if total bars is less than 60 bars { Alert("We have less than 60 bars, EA will now exit!!"); return; } MqlRates mrate[]; // To be used to store the prices, volumes and spread of each bar /* Let's make sure our arrays values for the Rates and Indicators is stored serially similar to the timeseries array */ // the rates arrays ArraySetAsSeries(mrate,true); ArraySetAsSeries(Signal,true); ArraySetAsSeries(Main,true); //--- Get the details of the latest 3 bars if(CopyRates(_Symbol,_Period,0,3,mrate)<0) { Alert("Error copying rates/history data - error:",GetLastError(),"!!"); return; } //--- Copy the new values of our indicators to buffers (arrays) using the handle if(CopyBuffer(Stoch,0,2,Main)<0 || CopyBuffer(Stoch,1,2,Signal)<0) { Alert("Error copying Stochastic indicator Buffers - error:",GetLastError(),"!!"); return; } double Ask = SymbolInfoDouble(_Symbol,SYMBOL_ASK); // Ask price double Bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); // Bid price //--- Declare bool type variables to hold our Buy and Sell Conditions bool Buy_Condition =(Main[1] > Signal[1] && Main[2] > Stoch_min && CANDLE_PATTERN_MORNING_STAR || CANDLE_PATTERN_THREE_WHITE_SOLDIERS || CANDLE_PATTERN_MORNING_DOJI ||CANDLE_PATTERN_BULLISH_ENGULFING || CANDLE_PATTERN_HAMMER || CANDLE_PATTERN_HANGING_MAN || CANDLE_PATTERN_PIERCING_LINE || CANDLE_PATTERN_BULLISH_HARAMI || CANDLE_PATTERN_BULLISH_MEETING_LINES ); // and DEMA is growing up bool Sell_Condition = (Main[1] < Signal[1] && Main[2] < Stoch_Max && CANDLE_PATTERN_THREE_BLACK_CROWS || CANDLE_PATTERN_DARK_CLOUD_COVER || CANDLE_PATTERN_EVENING_DOJI || CANDLE_PATTERN_BEARISH_ENGULFING || CANDLE_PATTERN_BEARISH_HARAMI || CANDLE_PATTERN_EVENING_STAR || CANDLE_PATTERN_BEARISH_MEETING_LINES || CANDLE_PATTERN_HAMMER || CANDLE_PATTERN_HANGING_MAN ); // bool Buy_Close=(Main[1] < Signal[1] && Main[2] < Stoch_Max && CANDLE_PATTERN_THREE_BLACK_CROWS || CANDLE_PATTERN_DARK_CLOUD_COVER || CANDLE_PATTERN_EVENING_DOJI || CANDLE_PATTERN_BEARISH_ENGULFING || CANDLE_PATTERN_BEARISH_HARAMI || CANDLE_PATTERN_EVENING_STAR || CANDLE_PATTERN_BEARISH_MEETING_LINES || CANDLE_PATTERN_HAMMER || CANDLE_PATTERN_HANGING_MAN ); // Black candle crossed the Upper Band from above to below bool Sell_Close=(Main[1] > Signal[1] && Main[2] > Stoch_min && CANDLE_PATTERN_MORNING_STAR || CANDLE_PATTERN_THREE_WHITE_SOLDIERS || CANDLE_PATTERN_MORNING_DOJI ||CANDLE_PATTERN_BULLISH_ENGULFING || CANDLE_PATTERN_HAMMER || CANDLE_PATTERN_HANGING_MAN || CANDLE_PATTERN_PIERCING_LINE || CANDLE_PATTERN_BULLISH_HARAMI || CANDLE_PATTERN_BULLISH_MEETING_LINES ); // White candle crossed the Lower Band from below to above if(Buy_Condition && !PositionSelect(_Symbol)) // Open long position { // DEÌÀ is growing up LongPositionOpen(); // and white candle crossed the Lower Band from below to above } if(Sell_Condition && !PositionSelect(_Symbol)) // Open short position { // DEÌÀ is falling down ShortPositionOpen(); // and Black candle crossed the Upper Band from above to below } if(Buy_Close && PositionSelect(_Symbol)) // Close long position { // Black candle crossed the Upper Band from above to below LongPositionClose(); } if(Sell_Close && PositionSelect(_Symbol)) // Close short position { // White candle crossed the Lower Band from below to above ShortPositionClose(); } return; } //+------------------------------------------------------------------+ //| Open Long position | //+------------------------------------------------------------------+ void LongPositionOpen() { MqlTradeRequest mrequest; // Will be used for trade requests MqlTradeResult mresult; // Will be used for results of trade requests ZeroMemory(mrequest); ZeroMemory(mresult); double Ask = SymbolInfoDouble(_Symbol,SYMBOL_ASK); // Ask price double Bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); // Bid price if(!PositionSelect(_Symbol)) { mrequest.action = TRADE_ACTION_DEAL; // Immediate order execution mrequest.price = NormalizeDouble(Ask,_Digits); // Lastest Ask price mrequest.sl = 0; // Stop Loss mrequest.tp = 0; // Take Profit mrequest.symbol = _Symbol; // Symbol mrequest.volume = Lot; // Number of lots to trade mrequest.magic = 0; // Magic Number mrequest.type = ORDER_TYPE_BUY; // Buy Order mrequest.type_filling = ORDER_FILLING_FOK; // Order execution type mrequest.deviation=5; // Deviation from current price OrderSend(mrequest,mresult); // Send order } } //+------------------------------------------------------------------+ //| Open Short position | //+------------------------------------------------------------------+ void ShortPositionOpen() { MqlTradeRequest mrequest; // Will be used for trade requests MqlTradeResult mresult; // Will be used for results of trade requests ZeroMemory(mrequest); ZeroMemory(mresult); double Ask = SymbolInfoDouble(_Symbol,SYMBOL_ASK); // Ask price double Bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); // Bid price if(!PositionSelect(_Symbol)) { mrequest.action = TRADE_ACTION_DEAL; // Immediate order execution mrequest.price = NormalizeDouble(Bid,_Digits); // Lastest Bid price mrequest.sl = 0; // Stop Loss mrequest.tp = 0; // Take Profit mrequest.symbol = _Symbol; // Symbol mrequest.volume = Lot; // Number of lots to trade mrequest.magic = 0; // Magic Number mrequest.type= ORDER_TYPE_SELL; // Sell order mrequest.type_filling = ORDER_FILLING_FOK; // Order execution type mrequest.deviation=5; // Deviation from current price OrderSend(mrequest,mresult); // Send order } } //+------------------------------------------------------------------+ //| Close Long position | //+------------------------------------------------------------------+ void LongPositionClose() { MqlTradeRequest mrequest; // Will be used for trade requests MqlTradeResult mresult; // Will be used for results of trade requests ZeroMemory(mrequest); ZeroMemory(mresult); double Ask = SymbolInfoDouble(_Symbol,SYMBOL_ASK); // Ask price double Bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); // Bid price if(PositionGetInteger(POSITION_TYPE)==POSITION_TYPE_BUY) { mrequest.action = TRADE_ACTION_DEAL; // Immediate order execution mrequest.price = NormalizeDouble(Bid,_Digits); // Lastest Bid price mrequest.sl = 0; // Stop Loss mrequest.tp = 0; // Take Profit mrequest.symbol = _Symbol; // Symbol mrequest.volume = Lot; // Number of lots to trade mrequest.magic = 0; // Magic Number mrequest.type= ORDER_TYPE_SELL; // Sell order mrequest.type_filling = ORDER_FILLING_FOK; // Order execution type mrequest.deviation=5; // Deviation from current price OrderSend(mrequest,mresult); // Send order } } //+------------------------------------------------------------------+ //| Close Short position | //+------------------------------------------------------------------+ void ShortPositionClose() { MqlTradeRequest mrequest; // Will be used for trade requests MqlTradeResult mresult; // Will be used for results of trade requests ZeroMemory(mrequest); ZeroMemory(mresult); double Ask = SymbolInfoDouble(_Symbol,SYMBOL_ASK); // Ask price double Bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); // Bid price if(PositionGetInteger(POSITION_TYPE)==POSITION_TYPE_SELL) { mrequest.action = TRADE_ACTION_DEAL; // Immediate order execution mrequest.price = NormalizeDouble(Ask,_Digits); // Latest ask price mrequest.sl = 0; // Stop Loss mrequest.tp = 0; // Take Profit mrequest.symbol = _Symbol; // Symbol mrequest.volume = Lot; // Number of lots to trade mrequest.magic = 0; // Magic Number mrequest.type = ORDER_TYPE_BUY; // Buy order mrequest.type_filling = ORDER_FILLING_FOK; // Order execution type mrequest.deviation=5; // Deviation from current price OrderSend(mrequest,mresult); // Send order } } //+------------------------------------------------------------------+
surubabs:
You post your code but don't correct it, why ?angevoyageur:
You post your code but don't correct it, why ?
Stochastic main signal stants for main, Signal stants for signal line,
You post your code but don't correct it, why ?
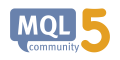
Documentation on MQL5: Standard Constants, Enumerations and Structures / Indicator Constants / Indicators Lines
- www.mql5.com
Standard Constants, Enumerations and Structures / Indicator Constants / Indicators Lines - Documentation on MQL5
I saw your code. Do you understand what I wrote ? CopyBuffer need 5 parameters. You have only 4.
angevoyageur:
I saw your code. Do you understand what I wrote ? CopyBuffer need 5 parameters. You have only 4.
I saw your code. Do you understand what I wrote ? CopyBuffer need 5 parameters. You have only 4.
No i dont understand, but i could find a code like this in some other code base, but i dont know how to use it.
//+------------------------------------------------------------------+ //| Filling indicator buffers from the iStochastic indicator | //+------------------------------------------------------------------+ bool FillArraysFromBuffers(double &main_buffer[], // indicator buffer of Stochastic Oscillator values double &signal_buffer[], // indicator buffer of the signal line int ind_handle, // handle of the iStochastic indicator int amount // number of copied values ) { //--- reset error code ResetLastError(); //--- fill a part of the StochasticBuffer array with values from the indicator buffer that has 0 index if(CopyBuffer(ind_handle,MAIN_LINE,0,amount,main_buffer)<0) { //--- if the copying fails, tell the error code PrintFormat("Failed to copy data from the iStochastic indicator, error code %d",GetLastError()); //--- quit with zero result - it means that the indicator is considered as not calculated return(false); } //--- fill a part of the SignalBuffer array with values from the indicator buffer that has index 1 if(CopyBuffer(ind_handle,SIGNAL_LINE,0,amount,signal_buffer)<0) { //--- if the copying fails, tell the error code PrintFormat("Failed to copy data from the iStochastic indicator, error code %d",GetLastError()); //--- quit with zero result - it means that the indicator is considered as not calculated return(false); } //--- everything is fine return(true); }i dont understand what is amount stants for !
surubabs:
How many data do you want to copy ? It's "amount" or instead "count".No i dont understand, but i could find a code like this in some other code base, but i dont know how to use it.
i dont understand what is amount stants for !
angevoyageur:
How many data do you want to copy ? It's "amount" or instead "count".
Only Main signal value and Signal line value i need,
How many data do you want to copy ? It's "amount" or instead "count".
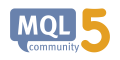
Documentation on MQL5: Standard Constants, Enumerations and Structures / Indicator Constants / Indicators Lines
- www.mql5.com
Standard Constants, Enumerations and Structures / Indicator Constants / Indicators Lines - Documentation on MQL5
For which bar(s)/candle(s) ?
angevoyageur:
For which bar(s)/candle(s) ?
For which bar(s)/candle(s) ? what does it meen ?
For which bar(s)/candle(s) ?

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Dear friend i am little confused how to coppy buffer of stochastic ?
Please correct this for me,,
Thanks in a advance
Suresh