Hi!
First things first you need visual studio for this .
Install it , start it up.
- Select the programming language
- Select the operating system
- Select Library
- Select Dynamic Link library , it will appear
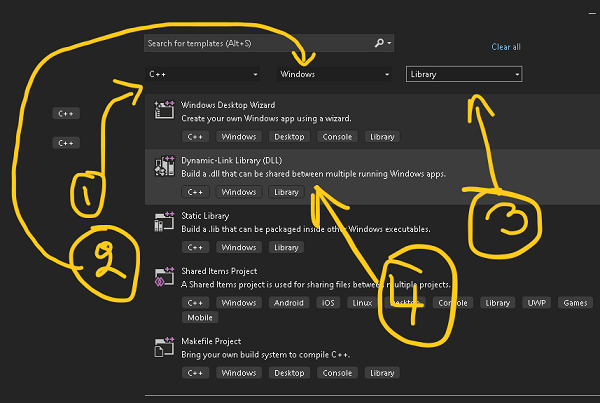
Click Next
give it a name
Click Create
wait for the slow pc to create the project
This is the first thing you may see , the explorer could be on the right in your setup .
It opens up with the dllMain.cpp in view :
Now , correct me if you are more experienced but ,whatever .cpp file (because we chose c++) you create inside the project could be accessible
in the DLL .
For this one let's pack everything in one file since we s*ck at programming . 😊
So let's create a new cpp file called "mySimpleDLL.cpp" , you can name it whatever you want don't worry.
- In the solutions explorerTab right click on the name of the project
- Go to menu item "add"
- Go to menu item "New item"
- From the popup select "C++ file" , if you cant see it click "detailed view" bottom left
- Name your file
- Hit "add"
Add the following on top of your new cpp file :
- Name it name + _EXPORTS
- Name it name +_API
Is that important (the naming convention) ? I don't know , someone more experienced can explain.
here is the code form :
#pragma once #include "pch.h" // use stdafx.h in Visual Studio 2017 and earlier #include <utility> #include <limits.h> #ifdef MYSIMPLEDLL_EXPORTS #define MYSIMPLEDLL_API __declspec(dllexport) #else #define MYSIMPLEDLL_API __declspec(dllimport) #endif
Excellent , now let's expose a function !
- extern "C"
- name of api as defined
- return type
- name of function
- function parameters
in code , simple a+b in the return :
extern "C" MYSIMPLEDLL_API int hard_math(int a, int b) { return(a+b); }
Awesome , but does it work ?
On the compile tab on the header :
- Click on "Debug" (if it says release skip this step)
- Select "Release"
wait for your fast pc to prepare
next to it
- select x86 if the DLL is for MT4
- select x64 if the DLL is for MT5
- click "Build" on the menu on top
- click "Build Solution"
If it compiles succesfully you will see this in the console
Cool now , let's find the file (the dll)
- under solution explorer right click the name of the project
- select "open folder in file explorer"
the file explorer will send you to a folder looking like this :
Go one level up , you need to be in the folder marked with the pink marker
- Navigate in the "Release" folder
- Your dll file is there for x86 (32bit)
Copy it and move it to your expert advisor folder to test it .
For the first test create a folder in experts called mySimpleDLL and place the mySimpleDLL.dll dll in that folder .
create a test1.mq4 file in the editor , it should look like this
In the test1.mq4 we add this to the header to import the function from the dll :
#import "mySimpleDLL.dll" int hard_math(int a,int b); #import
let's see if it does not work.
this is the source code :
#property version "1.00" #property strict #import "mySimpleDLL.dll" int hard_math(int a,int b); #import int OnInit() { int result=hard_math(3,5); Print("DLL says "+IntegerToString(result)); return(INIT_SUCCEEDED); } void OnDeinit(const int reason) { } void OnTick() { }
Compile it and when attaching to the chart make sure this is checked in the EA :
Also :
- Go to Tools
- Go to options
- Go to expert advisors
- Make sure the allow dll imports is checked
That's it , time to test it .
it does . Awesome