Seufz, ChatGPT :( Aber
- Wir können hier deutsch sprechen ;)
- Auch vor ChtGPT sollte man wissen, wie ein MQL5-Prigramm aussehen soll,
Dazu z.B.
https://www.mql5.com/en/articles/496
https://www.mql5.com/en/articles/100
https://www.mql5.com/en/articles/599
https://www.mql5.com/en/articles/232 - Es gibt Fehler bei der Kompilierung und zur Laufzeit - wo ist er denn bei Dir?
- Es gibt fast nichts, was nicht schon für MT5 programmiert wurde!
Daher wäre suchen besser als ChatGPT:
Supertrend in der Codebase: https://www.mql5.com/en/search#!keyword=Supertrend&module=mql5_module_codebase
Oder über Google nach: site:mql5.com Supertrend
Die funktionieren!
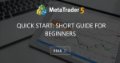
Quick Start: Short Guide for Beginners
- www.mql5.com
Hello dear reader! In this article, I will try to explain and show you how you can easily and quickly get the hang of the principles of creating Expert Advisors, working with indicators, etc. It is beginner-oriented and will not feature any difficult or abstruse examples.

Sie verpassen Handelsmöglichkeiten:
- Freie Handelsapplikationen
- Über 8.000 Signale zum Kopieren
- Wirtschaftsnachrichten für die Lage an den Finanzmärkte
Registrierung
Einloggen
Sie stimmen der Website-Richtlinie und den Nutzungsbedingungen zu.
Wenn Sie kein Benutzerkonto haben, registrieren Sie sich
Hello guys, i am not very good with mql5, but with the help of chatgpt i tried to transform a Supertrend Script from pinescript to mql5.
This is my Code: