
You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Nice article. The Prediction Graph on Testing Data is disappointing. You might as well skip all that DNN modeling/training and simply use a prediction of the next price equal to the last know price. I bet the prediction accuracy of such trivial model will be higher that using your DNN model. I suggest to compare those two accuracies and show them here. In general, using DNN to predict prices is a bad idea. They are better suited for classification of the price patterns (e.g., buy, sell, hold). Also, the number of weights in your DNN is astronomical. It must be overfitting.
Thank you Vladimir.
Just for discussion, when time permitted, I would modify this model to do classification for comparison.
Please let me know if you have ideas.
The tutorial gives a input of one batch SAMPLE_SIZE number of close inputs, you want one batch of input_count inputs.
Also your model doesn't use floats as inputs, but doubles,
Moderator's note: This post is out of sequence due to posts below been moved from another topic. Please see post below.
Can We Troubleshoot this ONNX Model Together?
Hello MQL5 community, I've been trying to follow this tutorial on how to use ONNX in your EA's. In the tutorial a neural network was the model of choice, I've used a gradient boosted tree.
I built the model using the InterpretML Python package, and exported it to ONNX using ebm2onnx.
I'll summaries the process by which the model was trained.
1) The model was trained on 5 inputs, OHLC and Height, Height is calculated as ((H + L) / 2) - C.
2) The model is a binary classifier, aiming to classify the next candle as either UP (1) or DOWN (0).
The data used to train the model.
3) The model was then exported to ONNX format
ONNX model representation.
To get the model to work, I deviated from the code in the tutorial and kept editing the code to try make the ONNX model work, but now I really don't know what I'm doing wrong. I keep getting an error that the handle for the model is invalid.
I have attached the MQL5 code below.
I'll now summaries the steps that I took in my code that deviate from what is in the tutorial, and I'll explain why I deviated from the tutorial as well
1) LINE 57: Setting Model Input Shape.
In the tutorial 3 dimensions were used to set the input and output shape i.e. {1,SAMPLE_SIZE,1}; However when I followed that approach I kept getting an error, specifically error 5808. After the usual trail and error process I realized that if I used only 1 dimension, the number of inputs, the error went away.
2) LINE 68: Setting Model Output Shape.
Same logic as above.
The other deviations I made do not affect the model, for example I kept track of time using logic I found more intuitive than the logic implemented in the tutorial. I didn't need to normalize inputs because it's a tree-based model.
If you can spot other errors I have made , I'd appreciate your insight.
Forum on trading, automated trading systems and testing trading strategies
Discussion of article "How to use ONNX models in MQL5"
Stian Andreassen, 2023.12.08 20:51
The tutorial gives a input of one batch SAMPLE_SIZE number of close inputs, you want one batch of input_count inputs.
Also your model doesn't use floats as inputs, but doubles,
Thank you for sharing Sitan, I've applied what you pointed out but the error is still there
One batch of input_count inputs.
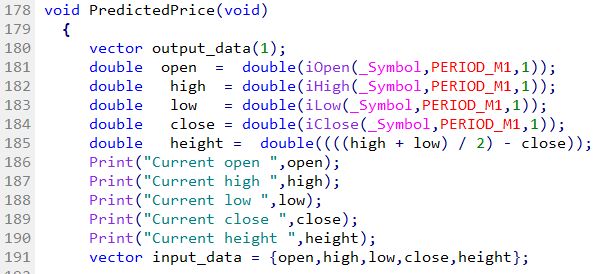
Set input to double
Error message.
Can We Troubleshoot this ONNX Model Together?
Hello MQL5 community, I've been trying to follow this tutorial on how to use ONNX in your EA's. In the tutorial a neural network was the model of choice, I've used a gradient boosted tree.
I built the model using the InterpretML Python package, and exported it to ONNX using ebm2onnx.
I'll summaries the process by which the model was trained.
1) The model was trained on 5 inputs, OHLC and Height, Height is calculated as ((H + L) / 2) - C.
2) The model is a binary classifier, aiming to classify the next candle as either UP (1) or DOWN (0).
The data used to train the model.
3) The model was then exported to ONNX format
ONNX model representation.
To get the model to work, I deviated from the code in the tutorial and kept editing the code to try make the ONNX model work, but now I really don't know what I'm doing wrong. I keep getting an error that the handle for the model is invalid.
I have attached the MQL5 code below.
I'll now summaries the steps that I took in my code that deviate from what is in the tutorial, and I'll explain why I deviated from the tutorial as well
1) LINE 57: Setting Model Input Shape.
In the tutorial 3 dimensions were used to set the input and output shape i.e. {1,SAMPLE_SIZE,1}; However when I followed that approach I kept getting an error, specifically error 5808. After the usual trail and error process I realized that if I used only 1 dimension, the number of inputs, the error went away.
2) LINE 68: Setting Model Output Shape.
Same logic as above.
The other deviations I made do not affect the model, for example I kept track of time using logic I found more intuitive than the logic implemented in the tutorial. I didn't need to normalize inputs because it's a tree-based model.
If you can spot other errors I have made , I'd appreciate your insight.
Thank you for sharing Sitan, I've applied what you pointed out but the error is still there
One batch of input_count inputs.
Set input to double
Error message.
It seems that MQL5 does not (or rather ONNXMLTools does not) yet support EBM's ONNX:
https://www.mql5.com/en/docs/onnx/onnx_conversion
If you refer to the ONNX attachments (especially model.eurusd.D1.10.class.onnx which uses 4 inputs) from https://www.mql5.com/en/articles/12484; and use Netron (web version) to visualise the onnx files you will see the differences.
I think the following two articles would help you understand further too:
Regression models of the Scikit-learn Library and their export to ONNX
Classification models in the Scikit-Learn library and their export to ONNX
Hi everybody,
We are trying to use a keras neural network with 11 predictors at one point in time (batch size 32) to make predictions on the XauUsd (where the output is a singular number between 0 and 1). Firstly, we load in from OnnxCreatefrombuffer (because OnnxCreate itself doesn't work for us), then we always get an error on the OnnxRun stage, where I have attached both errors below. Any help on what dimension to reshape to for the input, what format to put our predictor vector in (if it should be a vector at all?), or just any help or suggestions with syntax to help tackle these errors would be amazing. We have tried reshaping to all sorts of combinations of 32,1,11 vectors and no luck and really no idea with the next steps.. Thanks so much for anyone who can help !! Ben.
' error 5808'
' ONNX: input parameter #0 tensor has wrong dimension [0], try to use OnnxSetInputShape'
'ONNX: invalid input parameter #0 size, expected 1408 bytes instead of 480'
Hi, I try to use the
OnnxModelInfo.mq5
file script, but can't make it work, what am I doing wrong? this cant be so complicated!
I copy pasted the OnnxModelInfo script, and saved into de Files folder
I have a onnx model (attached)
and when I compilate the script, 21 errors appear.
Can some one help me with this? Please