estou tentando fazer um cruzamento entre média móvel e o indicador AFIRMA, mas nao roda no backtest pois aparece erro nessa linha: int Afirmahandle =iCustom(0,0,2,21,Blackman,0); dizendo algo sobre o "indicador 2" (referente ao número 2 da sequencia anterior).
se alguém souber, poderia me ajudar?
Bom dia!
O autor já publicou também um EA baseado no indicador AFIRMA.
Obs.: Ao postar código, utilize esse botão (ou Alt+S) para facilitar a leitura / análise.
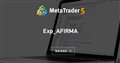
- www.mql5.com
Bom dia!
O autor já publicou também um EA baseado no indicador AFIRMA.
Obs.: Ao postar código, utilize esse botão (ou Alt+S) para facilitar a leitura / análise.
SIM, mas ele não compila, pois aparece muitos erros.
SIM, mas ele não compila, pois aparece muitos erros.
Vou dar uma olhada ...
Aqui não deu erro de compilação. Acho que você não está salvando os arquivos nas pastas corretas:
Aqui não deu erro de compilação. Acho que você não está salvando os arquivos nas pastas corretas:
Eu baixei esse outro arquivo e eu tinha errado e baixei um diferente, mas fiz o download correto e compilei, e apareceu
o aviso na compilação:
return value of 'OrderCalcProfit' should be checked tradealgorithms.mqh 1170 10.
e no backtest nao funciona, pois aparece varios alertas e ele nao realizada nenhuma ordem de compra ou venda; o gráfico corre normalmente, mas não realiza operações.
Eu baixei esse outro arquivo e eu tinha errado e baixei um diferente, mas fiz o download correto e compilei, e apareceu
o aviso na compilação:
return value of 'OrderCalcProfit' should be checked tradealgorithms.mqh 1170 10.
e no backtest nao funciona, pois aparece varios alertas e ele nao realizada nenhuma ordem de compra ou venda; o gráfico corre normalmente, mas não realiza operações.
Esses avisos não impedem a execução do EA.
Faça o seguinte:
- Crie uma pasta \MQL5\Indicators\Testes e salve o indicador nessa pasta;
- No código do EA, onde tem:
InpInd_Handle=iCustom(Symbol(),InpInd_Timeframe,"AFIRMA",Periods,Taps,Window,0);
... Atere para:
InpInd_Handle=iCustom(Symbol(),InpInd_Timeframe,"Testes\\AFIRMA",Periods,Taps,Window,0);
Salvar o indicador AFIRMA na pasta Terminal\[...]\MQL5\Indicators\Testes.
[CÓDIGO ORIGINAL AQUI ...]
//+------------------------------------------------------------------+ //| MA Cross.mq5 | //| Copyright 2021, MetaQuotes Ltd. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, MetaQuotes Ltd." #property link "https://www.mql5.com" #property version "1.00" //--- #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> #include <Trade\SymbolInfo.mqh> //--- CPositionInfo m_position; // trade position object CTrade m_trade; // trading object CSymbolInfo m_symbol; // symbol info object //+----------------------------------------------+ //| declaration of enumerations | //+----------------------------------------------+ enum ENUM_WINDOWS //Type of constant { Rectangular = 1, //Rectangular window Hanning1, //Hanning window 1 Hanning2, //Hanning window 2 Blackman, //Blackman window Blackman_Harris //Blackman-Harris window }; //+----------------------------------------------+ //| AFIRMA indicator input parameters | //+----------------------------------------------+ input int Periods = 4; // 1/(2*Periods) filter bandwidth input int Taps = 21; // Taps (odd number) input ENUM_WINDOWS Window = Blackman; // Window index input uint SignalBar = 1; // Bar number for getting an entry signal //--- slow ma input int MA_2_Period = 13; // Averaging period of the 2nd MA input ENUM_MA_METHOD MA_2_Method = MODE_LWMA; // Method for calculating MA2 input ENUM_APPLIED_PRICE MA_2_Price = PRICE_MEDIAN; // Method for calculating the price MA2 input int MA_2_Shift = 0; // Indicator shift MA2 //--- volume input double Lot = 0.1; // Fixed lot //--- int New_Bar; // 0/1 Факт образования нового бара datetime Time_0; // Время начала нового бара int PosOpen; // Направление пересечения int PosClose; // Направление пересечения int all_positions; // Количество открытых позиций double MA1_0; // Текущее значение 1-й МА double MA1_1; // Предыдущее значение 1-й МА double MA2_0; // Текущее значение 2-й МА double MA2_1; // Предыдущее значение 2-й МА int PosBuy; // 1 = факт наличия позиции Buy int PosSell; // 1 = факт наличия позиции Sell //--- int handle_iMA_1; // variable for storing the handle of the AFIRMA indicator int handle_iMA_2; // variable for storing the handle of the iMA indicator //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { m_symbol.Name(Symbol()); // sets symbol name //--- create handle of the indicator AFIRMA handle_iMA_1=iCustom(m_symbol.Name(),Period(),"Testes\\AFIRMA",Periods,Taps,Window,0); //--- if the handle is not created if(handle_iMA_1==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the AFIRMA indicator for the symbol %s/%s, error code %d", m_symbol.Name(), EnumToString(Period()), GetLastError()); //--- the indicator is stopped early return(INIT_FAILED); } //--- create handle of the indicator iMA handle_iMA_2=iMA(m_symbol.Name(),Period(),MA_2_Period,MA_2_Shift,MA_2_Method,MA_2_Price); //--- if the handle is not created if(handle_iMA_2==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the iMA indicator for the symbol %s/%s, error code %d", m_symbol.Name(), EnumToString(Period()), GetLastError()); //--- the indicator is stopped early return(INIT_FAILED); } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { if(!RefreshRates()) return; PosBuy=0; PosSell=0; int openOrders=0; all_positions=PositionsTotal(); // Общее количество позиций for(int i=all_positions-1;i>=0;i--) // returns the number of open positions { if(m_position.SelectByIndex(i)) // Выбираем позицию { if(m_position.PositionType()==POSITION_TYPE_BUY)// Если позиция BUY { PosBuy=1; if(CrossPositionClose()==1) // Закрывем ордер, если удовлетворяет условию CrossPositionClose()=1 { m_trade.PositionClose(m_position.Ticket()); } } if(m_position.PositionType()==POSITION_TYPE_SELL) // Если позиция SELL { PosSell=1; if(CrossPositionClose()==2) // Закрывем ордер, если удовлетворяет условию CrossPositionClose()=2 { m_trade.PositionClose(m_position.Ticket()); } } } } New_Bar=0; // Для начала обнулимся if(Time_0!=iTime(m_symbol.Name(),Period(),0)) // Если уже другое время начала бара { New_Bar=1; // А вот и новый бар Time_0=iTime(m_symbol.Name(),Period(),0); // Запомним время начала нового бара } MA1_0=iMAGet(handle_iMA_1, 1, SignalBar); // Current value of 1st MA MA1_1=iMAGet(handle_iMA_1, 1, SignalBar + 1); // Previous value of 1st MA MA2_0=iMAGet(handle_iMA_2, 0, 0); // Current value of 2nd MA MA2_1=iMAGet(handle_iMA_2, 0, 1); // Previous value of 2nd MA if(CrossPositionOpen()==1 && New_Bar==1) // Движение снизу вверх = откр. Buy { OpenBuy(); } if(CrossPositionOpen()==2 && New_Bar==1) // Движение сверху вниз = откр. Sell { OpenSell(); } return; } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int CrossPositionOpen() { PosOpen=0; // Вот где собака зарыта!!:) if((MA1_1<=MA2_0 && MA1_0>MA2_0) || (MA1_1<MA2_0 && MA1_0>=MA2_0)) // Пересечение снизу вверх { PosOpen=1; } if((MA1_1>=MA2_0 && MA1_0<MA2_0) || (MA1_1>MA2_0 && MA1_0<=MA2_0)) // Пересечение сверху вниз { PosOpen=2; } return(PosOpen); // Возвращаем направление пересечен. } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int CrossPositionClose() { PosClose=0; // Вот где собака зарыта!!:) if((MA1_1>=MA2_0 && MA1_0<MA2_0) || (MA1_1>MA2_0 && MA1_0<=MA2_0)) // Пересечение сверху вниз { PosClose=1; } if((MA1_1<=MA2_0 && MA1_0>MA2_0) || (MA1_1<MA2_0 && MA1_0>=MA2_0)) // Пересечение снизу вверх { PosClose=2; } return(PosClose); // Возвращаем направление пересечен. } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OpenBuy() { if(all_positions==1) { for(int i=PositionsTotal()-1;i>=0;i--) // returns the number of open positions if(m_position.SelectByIndex(i)) if(m_position.PositionType()==POSITION_TYPE_BUY) return; // Если buy, то не открываемся } if(!RefreshRates()) return; m_trade.Buy(Lot,m_symbol.Name(),m_symbol.Ask(),0.0,0.0,"Buy: MA_cross_Method_PriceMode");// Открываемся return; } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OpenSell() { if(all_positions==1) { for(int i=PositionsTotal()-1;i>=0;i--) // returns the number of open positions if(m_position.SelectByIndex(i)) if(m_position.PositionType()==POSITION_TYPE_SELL) return; // Если sell, то не открываемся } m_trade.Sell(Lot,m_symbol.Name(),m_symbol.Bid(),0.0,0.0,"Sell: MA_cross_Method_PriceMode"); return; } //+------------------------------------------------------------------+ //| Refreshes the symbol quotes data | //+------------------------------------------------------------------+ bool RefreshRates() { //--- refresh rates if(!m_symbol.RefreshRates()) return(false); //--- protection against the return value of "zero" if(m_symbol.Ask()==0 || m_symbol.Bid()==0) return(false); //--- return(true); } //+------------------------------------------------------------------+ //| Get value of buffers for the iMA | //+------------------------------------------------------------------+ double iMAGet(const int handle,const int buffer_num, const int index) { double MA[]; ArraySetAsSeries(MA,true); //--- reset error code ResetLastError(); //--- fill a part of the iMABuffer array with values from the indicator buffer that has 0 index if(CopyBuffer(handle,buffer_num,0,index+1,MA)<0) { //--- if the copying fails, tell the error code PrintFormat("Failed to copy data from the indicator, error code %d",GetLastError()); //--- quit with zero result - it means that the indicator is considered as not calculated return(0.0); } return(MA[index]); } //+------------------------------------------------------------------+
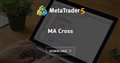
Salvar o indicador AFIRMA na pasta Terminal\[...]\MQL5\Indicators\Testes.
[CÓDIGO ORIGINAL AQUI ...]
eu consegui com um esboço de um cruzamento de 2 media, adicionando o icustom fazer ser compilado; porém o backtest não executou nenhuma ordem, ao fim aparecia na tela :
ERRO 4801:Ativo desconhecido.
Com esse seu código, também compilou e o backtest também não executou nenhuma ordem e apareceu ao final:
ERRO 4802: Indicador não pode ser criado.
Ou seja, o problema está no indicador Afirma que não é executado junto com todo o código, como se ele não estive sido introduzido ao que queremos...
PS: Obrigado pela dedicação e atenção...
eu consegui com um esboço de um cruzamento de 2 media, adicionando o icustom fazer ser compilado; porém o backtest não executou nenhuma ordem, ao fim aparecia na tela :
ERRO 4801:Ativo desconhecido.
Com esse seu código, também compilou e o backtest também não executou nenhuma ordem e apareceu ao final:
ERRO 4802: Indicador não pode ser criado.
Ou seja, o problema está no indicador Afirma que não é executado junto com todo o código, como se ele não estive sido introduzido ao que queremos...
PS: Obrigado pela dedicação e atenção...
De nada.
Quando eu salvo o indicador AFIRMA diretamente na pasta MQL5\Indicators, realmente ocorre esse erro. Mas eu criei essa pasta MQL5\Indicators\Testes, salvei o indicador nela e alterei o path na criação do handle e funcionou.
Não sei qual a lógica de funcionar numa pasta e noutra não, mas o fato é que aqui tá funcionando. 😃
Você tentou essa alteração como eu sugeri?
PS.: Testei no EURUSD H1 com os parâmetros default. Se você seguir essas sugestões e ainda assim não abrir nenhuma posição, dê uma olhada na guia Diário pra ver os logs de erros e retorne.
De nada.
Quando eu salvo o indicador AFIRMA diretamente na pasta MQL5\Indicators, realmente ocorre esse erro. Mas eu criei essa pasta MQL5\Indicators\Testes, salvei o indicador nela e alterei o path na criação do handle e funcionou.
Não sei qual a lógica de funcionar numa pasta e noutra não, mas o fato é que aqui tá funcionando. 😃
Você tentou essa alteração como eu sugeri?
PS.: Testei no EURUSD H1 com os parâmetros default. Se você seguir essas sugestões e ainda assim não abrir nenhuma posição, dê uma olhada na guia Diário pra ver os logs de erros e retorne.
Olhe o caminho declarado no codigo....
handle_iMA_1=iCustom(m_symbol.Name(),Period(),"Testes\\AFIRMA",Periods,Taps,Window,0);
A documentação diz o seguinte:
name
[in] O nome do indicador personalizado, com o caminho relativo ao diretório raiz de indicadores (MQL5\Indicators\). Se um indicador está localizado em um subdiretório, por exemplo, em MQL5/Indicadores/Exemplos, o seu nome deve ser especificado como: "Examples\\nome_do_indicador" (é necessário utilizar uma barra dupla, em vez de uma única barra como um separador).
Na atualização 2845 temos uma abordagem melhorada.
If the backslash '\' is indicated before the custom indicator name, the EX5 indicator file is searched relative to the MQL5 root folder. So, for a call of iCustom(Symbol(), Period(), "\FirstIndicator"...), the indicator will be loaded as MQL5\FirstIndicator.ex5. If the file is not found at this path, error 4802 (ERR_INDICATOR_CANNOT_CREATE) is returned.
If the path does not start with a backslash '\', the indicator is searched and loaded based on the following sequence of actions:
- The EX5 file is searched in the same folder, where the caller program's EX5 is located. For example, the CrossMA.EX5 Expert Advisor is located at MQL5\Experts\MyExperts. It contains the following call: iCustom(Symbol(), Period(), "SecondIndicator"...). In this case, the indicator is searched at MQL5\Experts\MyExperts\SecondIndicator.ex5.
- If the indicator is not found, a search relative to the Indicators root directory is performed: MQL5\Indicators. Thus, file MQL5\Indicators\SecondIndicator.ex5 is searched. If the indicator is not found, the function returns INVALID_HANDLE and error 4802 (ERR_INDICATOR_CANNOT_CREATE) is raised.
Also, if a custom indicator call via iCustom is found in the program code, the compiler will implicitly add the "#property tester_indicator XXX" directive if it is not specified.
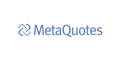
- 2020.06.05
- MetaQuotes
- www.metaquotes.net

- Aplicativos de negociação gratuitos
- 8 000+ sinais para cópia
- Notícias econômicas para análise dos mercados financeiros
Você concorda com a política do site e com os termos de uso
estou tentando fazer um cruzamento entre média móvel e o indicador AFIRMA, mas nao roda no backtest pois aparece erro nessa linha: int Afirmahandle =iCustom(0,0,2,21,Blackman,0); dizendo algo sobre o "indicador 2" (referente ao número 2 da sequencia anterior).
se alguém souber, poderia me ajudar?
//+------------------------------------------------------------------+