ストラテジーテスターで作ったEAが機能しないのですがどうしたらいいですか?(参考のためEAのコードを貼っておきます)
//+------------------------------------------------------------------+
//| MyExpertAdvisor.mq4 |
//| Generated by Aqua |
//+------------------------------------------------------------------+
#property strict
// Inputs
input double TakeProfitPoints = 50;
input double StopLossPoints = 20;
input double LotSize = 0.1;
input double HorizontalLinePrice = 1.2000; // 水平線の価格を設定
// Global variables
double EntryPrice = 0;
bool InPosition = false;
double TotalProfit = 0.0;
int TextHandle;
string HorizontalLineName = "TradeHorizontalLine";
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
Print("EA Initialized");
// テキストオブジェクトの作成
TextHandle = ObjectCreate(0, "TotalProfitText", OBJ_LABEL, 0, 0, 0);
if (TextHandle < 0)
{
Print("Error creating text object: ", GetLastError());
return(INIT_FAILED);
}
ObjectSetInteger(0, "TotalProfitText", OBJPROP_CORNER, CORNER_LEFT_UPPER);
ObjectSetInteger(0, "TotalProfitText", OBJPROP_XDISTANCE, 10);
ObjectSetInteger(0, "TotalProfitText", OBJPROP_YDISTANCE, 10);
ObjectSetInteger(0, "TotalProfitText", OBJPROP_FONTSIZE, 12);
ObjectSetString(0, "TotalProfitText", OBJPROP_TEXT, "Total Profit: 0.0");
// 水平線の作成
if (!ObjectCreate(0, HorizontalLineName, OBJ_HLINE, 0, 0, HorizontalLinePrice))
{
Print("Error creating initial horizontal line: ", GetLastError());
}
else
{
ObjectSetInteger(0, HorizontalLineName, OBJPROP_COLOR, clrRed);
ObjectSetInteger(0, HorizontalLineName, OBJPROP_WIDTH, 2);
ObjectSetDouble(0, HorizontalLineName, OBJPROP_PRICE, HorizontalLinePrice);
Print("Initial horizontal line created at price: ", HorizontalLinePrice);
}
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
Print("EA Deinitialized. Reason: ", reason);
// テキストオブジェクトの削除
ObjectDelete(0, "TotalProfitText");
ObjectDelete(0, HorizontalLineName); // 水平線の削除
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
double currentPrice = NormalizeDouble(Bid, Digits);
Print("Current Price: ", currentPrice);
// Check for break and rebound
if (!InPosition && CheckBreakAndRebound())
{
Print("Break and Rebound condition met.");
int ticket = OrderSend(Symbol(), OP_BUY, LotSize, Ask, 2, 0, 0, "Break and Rebound Entry", 0, 0, clrBlue);
if (ticket < 0)
{
Print("Error opening order: ", GetLastError());
}
else
{
Print("Order opened successfully. Ticket: ", ticket);
EntryPrice = Ask;
InPosition = true;
// トレード中の水平線の作成
if (!ObjectCreate(0, HorizontalLineName, OBJ_HLINE, 0, 0, HorizontalLinePrice))
{
Print("Error creating trade horizontal line: ", GetLastError());
}
else
{
ObjectSetInteger(0, HorizontalLineName, OBJPROP_COLOR, clrRed);
ObjectSetInteger(0, HorizontalLineName, OBJPROP_WIDTH, 2);
ObjectSetDouble(0, HorizontalLineName, OBJPROP_PRICE, HorizontalLinePrice);
Print("Trade horizontal line created at price: ", HorizontalLinePrice);
}
}
}
// Manage position
if (InPosition)
{
double tp = EntryPrice + (TakeProfitPoints * Point);
double sl = EntryPrice - (StopLossPoints * Point);
Print("Managing Position. TP: ", tp, " SL: ", sl);
bool modified = OrderModify(OrderTicket(), OrderOpenPrice(), sl, tp, 0, clrRed);
if (!modified)
{
Print("Error modifying order: ", GetLastError());
}
else
{
Print("Order modified successfully.");
}
// Check for take profit or stop loss
if (Bid >= tp || Bid <= sl)
{
bool closed = OrderClose(OrderTicket(), OrderLots(), Bid, 2, clrGreen);
if (!closed)
{
Print("Error closing order: ", GetLastError());
}
else
{
Print("Order closed successfully.");
InPosition = false;
// 総利益の更新
double profit = OrderProfit();
TotalProfit += profit;
// チャートに総利益を表示
string profitText = "Total Profit: " + DoubleToString(TotalProfit, 2);
ObjectSetString(0, "TotalProfitText", OBJPROP_TEXT, profitText);
Print(profitText);
// トレード中の水平線の削除
ObjectDelete(0, HorizontalLineName);
}
}
}
}
// Function to check for break and rebound
bool CheckBreakAndRebound()
{
double previousLow = iLow(Symbol(), 0, 1);
double currentPrice = Bid;
// 水平線をブレイクしたかどうかを確認
Print("Previous Low: ", previousLow, " Current Price: ", currentPrice, " Horizontal Line Price: ", HorizontalLinePrice);
if (currentPrice > HorizontalLinePrice && previousLow <= HorizontalLinePrice)
{
Print("Price broke above the horizontal line.");
return true;
}
Print("Checking for break and rebound. Condition not met.");
return false;
}
- expert advisor - miscellaneous questions
- Questions from Beginners MQL4 MT4 MetaTrader 4
- help for write my first EA in mql4
ストラテジーテスターで作ったEAが機能しないのですがどうしたらいいですか?(参考のためEAのコードを貼っておきます)
「機能しない」とは、通常チャートに挿入した場合は動作するが、ストラテジーテスターで動作しないということですか?
何かエラーがでるのですか?
現象や問題点を具体的に書かれた方が、問題解決が早いと思います。
また、コードは以下で編集されると良いと思います。
まだストラテジーテスターでしか動作確認はしてません。
MetaEditorでブレイクポイントを置いて、デバッグされてみてはいかがでしょうか?
チャートに水平線を引くようにしているのですがポジションを取らないどころか水平線も引かれません。
ぱっと見なのですが、
11行目
input double HorizontalLinePrice = 1.2000; // 水平線の価格を設定
160行目
if (currentPrice > HorizontalLinePrice && previousLow <= HorizontalLinePrice)
入力値(HorizontalLinePrice)で、HLineを引いていますが、銘柄によっては表示範囲(目視できる)を超えた値を設定されていませんか?
また、
入力値(HorizontalLinePrice) で、ブレイク判定していますが、この点は問題ないですか?
実行してみましたが、赤い水平ラインは描かれていますし、オーダーも入っているっぽいです。
USDJPY、M5で、期間:2025/01/31 ~ 2025/02/28
設定: HorizontalLinePrice のみ変更 154.0
ちなみに、ご存知かとは思いますが、オブジェクトがチャートに描画されているかどうかの確認は、
チャートで右クリック>表示中のライン等リスト を選択すると確認できます。
ではでは。
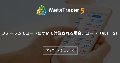
【モデレーターの秘訣】フォーラムでコードについて討議される場合、コード(Alt+S)の機能をご活用ください。
- 2025.03.18
- Sky All
- www.mql5.com
皆様 お世話になっております。 フォーラムでコードについて討議されたい場合、そのまま貼り付けると、ロボに勝手に削除される場合がございます。 できれる限り、コード(Alt+S)の機能をご活用いただければ幸甚です。 引き続き、どうぞよろしくお願いいたします...

取引の機会を逃しています。
- 無料取引アプリ
- 8千を超えるシグナルをコピー
- 金融ニュースで金融マーケットを探索