Franco Ferrante:
Esta escribiendo mal la orden de compra/venta. Fíjese en el orden de los valores.
bool Buy( double volume, // volumen de la posición const string symbol=NULL, // símbolo double price=0.0, // precio double sl=0.0, // precio stop loss double tp=0.0, // precio take profit const string comment="" // comentario )
Y comparelos en como los rellena usted.
trade.Buy(InitialLotSize, NULL, 0, tp);
Aquí toda la información en la documentación: https://www.mql5.com/es/docs/standardlibrary/tradeclasses/ctrade/ctradebuy
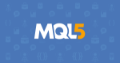
Documentación para MQL5: Biblioteca estándar / Clases de comercio / CTrade / Buy
- www.mql5.com
Abre la posición larga con los parámetros especificados. Parámetros volume [in] Volumen de la posición. symbol=...

Está perdiendo oportunidades comerciales:
- Aplicaciones de trading gratuitas
- 8 000+ señales para copiar
- Noticias económicas para analizar los mercados financieros
Registro
Entrada
Usted acepta la política del sitio web y las condiciones de uso
Si no tiene cuenta de usuario, regístrese
Hola, he intentado crear un robot, pero estoy teniendo un problema, cuando lo inicio en el probador de estrategias hay error. El robot debe iniciar con una compra/venta segun lo que elija y luego copiar una orden pendiente. Ni siquiera le pongo SL y me dice precio invalido y SL invalido.
Dejo los errores y el codigo.
failed market buy 0.01 XAUUSDx sl: 2059.99 [Invalid stops]
CTrade::OrderSend: market buy 0.01 XAUUSDx sl: 2059.99 [invalid stops]
failed buy limit 0.01 XAUUSDx at 2059.91 tp: 2060.91 [Invalid price]