Hi, I would like to extend the lines beyond the "Chart shift limit".
Where be the error in these command strings?
Thanks for the support!
ObjectSet("m.Fib", OBJPROP_RAY, false);
OBJPROP_RAY_RIGHT | Ray goes to the right |
bool |
OBJPROP_RAY |
A vertical line goes through all the windows of a chart |
bool |
UPDATE: It does not work, if the tracking is outside the (shift chart)
Try
OBJ_TRENDBYANGLE
https://www.mql5.com/en/docs/constants/objectconstants/enum_object/obj_trendbyangle
you have to set the angle too
OBJPROP_ANGLE
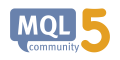
- www.mql5.com
Try
OBJ_TRENDBYANGLE
https://www.mql5.com/en/docs/constants/objectconstants/enum_object/obj_trendbyangle
you have to set the angle too
Thanks for the answer, but how and where the string must be inserted into code?
It's functions that you can use as is.
Please read the documentation.
//--- description #property description "Script draws \"Trend Line By Angle\" graphical object." #property description "Anchor point coordinates are set in percentage of the size of" #property description "the chart window." //--- display window of the input parameters during the script's launch #property script_show_inputs //--- input parameters of the script input string InpName="Trend"; // Line name input int InpDate1=50; // 1 st point's date, % input int InpPrice1=75; // 1 st point's price, % input int InpAngle=0; // Line's slope angle input color InpColor=clrRed; // Line color input ENUM_LINE_STYLE InpStyle=STYLE_DASH; // Line style input int InpWidth=2; // Line width input bool InpBack=false; // Background line input bool InpSelection=true; // Highlight to move input bool InpRayLeft=false; // Line's continuation to the left input bool InpRayRight=true; // Line's continuation to the right input bool InpHidden=true; // Hidden in the object list input long InpZOrder=0; // Priority for mouse click //+------------------------------------------------------------------+ //| Create a trend line by angle | //+------------------------------------------------------------------+ bool TrendByAngleCreate(const long chart_ID=0, // chart's ID const string name="TrendLine", // line name const int sub_window=0, // subwindow index datetime time=0, // point time double price=0, // point price const double angle=45.0, // slope angle const color clr=clrRed, // line color const ENUM_LINE_STYLE style=STYLE_SOLID, // line style const int width=1, // line width const bool back=false, // in the background const bool selection=true, // highlight to move const bool ray_left=false, // line's continuation to the left const bool ray_right=true, // line's continuation to the right const bool hidden=true, // hidden in the object list const long z_order=0) // priority for mouse click { //--- create the second point to facilitate dragging the trend line by mouse datetime time2=0; double price2=0; //--- set anchor points' coordinates if they are not set ChangeTrendEmptyPoints(time,price,time2,price2); //--- reset the error value ResetLastError(); //--- create a trend line using 2 points if(!ObjectCreate(chart_ID,name,OBJ_TRENDBYANGLE,sub_window,time,price,time2,price2)) { Print(__FUNCTION__, ": failed to create a trend line! Error code = ",GetLastError()); return(false); } //--- change trend line's slope angle; when changing the angle, coordinates of the second //--- point of the line are redefined automatically according to the angle's new value ObjectSetDouble(chart_ID,name,OBJPROP_ANGLE,angle); //--- set line color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- set line style ObjectSetInteger(chart_ID,name,OBJPROP_STYLE,style); //--- set line width ObjectSetInteger(chart_ID,name,OBJPROP_WIDTH,width); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- enable (true) or disable (false) the mode of moving the line by mouse //--- when creating a graphical object using ObjectCreate function, the object cannot be //--- highlighted and moved by default. Inside this method, selection parameter //--- is true by default making it possible to highlight and move the object ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- enable (true) or disable (false) the mode of continuation of the line's display to the left ObjectSetInteger(chart_ID,name,OBJPROP_RAY_LEFT,ray_left); //--- enable (true) or disable (false) the mode of continuation of the line's display to the right ObjectSetInteger(chart_ID,name,OBJPROP_RAY_RIGHT,ray_right); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Change trend line anchor point's coordinates | //+------------------------------------------------------------------+ bool TrendPointChange(const long chart_ID=0, // chart's ID const string name="TrendLine", // line name datetime time=0, // anchor point time coordinate double price=0) // anchor point price coordinate { //--- if point position is not set, move it to the current bar having Bid price if(!time) time=TimeCurrent(); if(!price) price=SymbolInfoDouble(Symbol(),SYMBOL_BID); //--- reset the error value ResetLastError(); //--- move trend line's anchor point if(!ObjectMove(chart_ID,name,0,time,price)) { Print(__FUNCTION__, ": failed to move the anchor point! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Change trend line's slope angle | //+------------------------------------------------------------------+ bool TrendAngleChange(const long chart_ID=0, // chart's ID const string name="TrendLine", // trend line name const double angle=45) // trend line's slope angle { //--- reset the error value ResetLastError(); //--- change trend line's slope angle if(!ObjectSetDouble(chart_ID,name,OBJPROP_ANGLE,angle)) { Print(__FUNCTION__, ": failed to change the line's slope angle! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Delete the trend line | //+------------------------------------------------------------------+ bool TrendDelete(const long chart_ID=0, // chart's ID const string name="TrendLine") // line name { //--- reset the error value ResetLastError(); //--- delete a trend line if(!ObjectDelete(chart_ID,name)) { Print(__FUNCTION__, ": failed to delete a trend line! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Check the values of trend line's anchor points and set default | //| values for empty ones | //+------------------------------------------------------------------+ void ChangeTrendEmptyPoints(datetime &time1,double &price1, datetime &time2,double &price2) { //--- if the first point's time is not set, it will be on the current bar if(!time1) time1=TimeCurrent(); //--- if the first point's price is not set, it will have Bid value if(!price1) price1=SymbolInfoDouble(Symbol(),SYMBOL_BID); //--- set coordinates of the second, auxiliary point //--- the second point will be 9 bars left and have the same price datetime second_point_time[10]; CopyTime(Symbol(),Period(),time1,10,second_point_time); time2=second_point_time[0]; price2=price1; } //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- check correctness of the input parameters if(InpDate1<0 || InpDate1>100 || InpPrice1<0 || InpPrice1>100) { Print("Error! Incorrect values of input parameters!"); return; } //--- number of visible bars in the chart window int bars=(int)ChartGetInteger(0,CHART_VISIBLE_BARS); //--- price array size int accuracy=1000; //--- arrays for storing the date and price values to be used //--- for setting and changing line anchor points' coordinates datetime date[]; double price[]; //--- memory allocation ArrayResize(date,bars); ArrayResize(price,accuracy); //--- fill the array of dates ResetLastError(); if(CopyTime(Symbol(),Period(),0,bars,date)==-1) { Print("Failed to copy time values! Error code = ",GetLastError()); return; } //--- fill the array of prices //--- find the highest and lowest values of the chart double max_price=ChartGetDouble(0,CHART_PRICE_MAX); double min_price=ChartGetDouble(0,CHART_PRICE_MIN); //--- define a change step of a price and fill the array double step=(max_price-min_price)/accuracy; for(int i=0;i<accuracy;i++) price[i]=min_price+i*step; //--- define points for drawing the line int d1=InpDate1*(bars-1)/100; int p1=InpPrice1*(accuracy-1)/100; //--- create a trend line if(!TrendByAngleCreate(0,InpName,0,date[d1],price[p1],InpAngle,InpColor,InpStyle, InpWidth,InpBack,InpSelection,InpRayLeft,InpRayRight,InpHidden,InpZOrder)) { return; } //--- redraw the chart and wait for 1 second ChartRedraw(); Sleep(1000); //--- now, move and rotate the line //--- loop counter int v_steps=accuracy/2; //--- move the anchor point and change the line's slope angle for(int i=0;i<v_steps;i++) { //--- use the following value if(p1>1) p1-=1; //--- move the point if(!TrendPointChange(0,InpName,date[d1],price[p1])) return; if(!TrendAngleChange(0,InpName,18*(i+1))) return; //--- check if the script's operation has been forcefully disabled if(IsStopped()) return; //--- redraw the chart ChartRedraw(); } //--- 1 second of delay Sleep(1000); //--- delete from the chart TrendDelete(0,InpName); ChartRedraw(); //--- 1 second of delay Sleep(1000); //--- }
It's functions that you can use as is.
Please read the documentation.
I would only to extend the fibo lines beyond the "Chart shift limit". Can you correct my string?
//+------------------------------------------------------------------+ //| AG Multi Color Fib.mq4 | //| Alan.81@live.it | //+------------------------------------------------------------------+ #property copyright "Alan Gasperi" #property indicator_chart_window extern int mLineExtend = 400; extern bool mSendAlerts = true; extern color mCol0 = SteelBlue; extern color mCol1 = SteelBlue; extern color mCol2 = DimGray; extern color mCol3 = Red; extern color mCol4 = Yellow; extern color mCol5 = Yellow; extern color mCol6 = Red; extern color mCol7 = SteelBlue; extern color mCol8 = SteelBlue; extern color mCol = DimGray; extern color mTxtCol = White; extern double mFib0 = 0.0; extern double mFib1 = 100.0; extern double mFib2 = 23.6; extern double mFib3 = 38.2; extern double mFib4 = 50.0; extern double mFib5 = 61.8; extern double mFib6 = 76.6; extern double mFib7 = 123.6; extern double mFib8 = 138.2; double mPipFact = 1, mP1, mP2, mFibPcnts[9], mFibs[9]; int mT1, mT2, mLabT, mArrSize; color mFibCols[9]; //------------------------------------------------------------------| int init() { if(Digits == 3 || Digits == 5) mPipFact = 10; mFibPcnts[0] = mFib0; mFibPcnts[1] = mFib1; mFibPcnts[2] = mFib2; mFibPcnts[3] = mFib3; mFibPcnts[4] = mFib4; mFibPcnts[5] = mFib5; mFibPcnts[6] = mFib6; mFibPcnts[7] = mFib7; mFibPcnts[8] = mFib8; mFibCols[0] = mCol0; mFibCols[1] = mCol1; mFibCols[2] = mCol2; mFibCols[3] = mCol3; mFibCols[4] = mCol4; mFibCols[5] = mCol5; mFibCols[6] = mCol6; mFibCols[7] = mCol7; mFibCols[8] = mCol8; mArrSize = ArraySize(mFibPcnts) +1 ; return(0); } //+------------------------------------------------------------------+ int deinit() { for(int i = ObjectsTotal(); i >= 0; i--) if(StringSubstr(ObjectName(i), 0, 2) == "m.") ObjectDelete(ObjectName(i)); return(0); } //+------------------------------------------------------------------+ int start() { CreateObj(); mT1 = iBarShift(NULL, 0, ObjectGet("m.Fib", 0)); mT2 = iBarShift(NULL, 0, ObjectGet("m.Fib", 2)); if(mT1 < mT2) { mT1 = mT2; mT2 = iBarShift(NULL, 0, ObjectGet("m.Fib", 0)); } mT2 = MathMax(0, mT2 - mLineExtend); SetFibs(); return(0); } //+------------------------------------------------------------------+ void CreateObj() { if(ObjectFind("m.Fib") == -1) { double mDepth = (WindowPriceMax(0) - WindowPriceMin(0)) / 4; ObjectCreate("m.Fib", OBJ_TREND, 0, Time[30], High[30], Time[10], High[30] + mDepth); ObjectSet("m.Fib", OBJPROP_RAY, false); ObjectSet("m.Fib", OBJPROP_WIDTH, 1); ObjectSet("m.Fib", OBJPROP_STYLE,STYLE_DOT); ObjectSet("m.Fib", OBJPROP_COLOR, mCol); } for(int c = 0; c < mArrSize; c++) { if(ObjectFind("m.Fib"+c) == -1) { ObjectCreate("m.Fib"+c, OBJ_TREND, 0, 0, 0, 0, 0); ObjectSet("m.Fib"+c, OBJPROP_RAY, false); ObjectSet("m.Fib"+c, OBJPROP_WIDTH, 2); ObjectCreate("m.Lab"+c, OBJ_TEXT, 0, 0, 0, 0, 0); } } return(0); } //+------------------------------------------------------------------+ void SetFibs() { double mRange; mP1 = ObjectGet("m.Fib", 1); mP2 = ObjectGet("m.Fib", 3); mRange = MathAbs(mP1 - mP2); if(mP2 < mP1) { ArraySort(mFibPcnts, WHOLE_ARRAY, 0, MODE_ASCEND); for(int y = 0; y < mArrSize; y++) mFibs[y] = mP1 - mRange * mFibPcnts[y] / 100; } else { ArraySort(mFibPcnts, WHOLE_ARRAY, 0, MODE_DESCEND); for(y = 0; y < mArrSize; y++) mFibs[y] = mP1 + mRange * mFibPcnts[y] / 100; } for(y = 0; y < mArrSize; y++) { ObjectSet("m.Fib"+y, OBJPROP_TIME1, Time[mT1]); ObjectSet("m.Fib"+y, OBJPROP_TIME2, Time[mT2]); ObjectSet("m.Fib"+y, OBJPROP_PRICE1, mFibs[y]); ObjectSet("m.Fib"+y, OBJPROP_PRICE2, mFibs[y]); ObjectSet("m.Fib"+y, OBJPROP_COLOR, mFibCols[y]); ObjectSetText("m.Lab"+y, DoubleToStr(mFibPcnts[y], 0) + "% " + DoubleToStr(mFibs[y], Digits), 9, "Arial", mTxtCol); mLabT = MathMin(mT1, mT2) + 4; ObjectSet("m.Lab"+y, OBJPROP_TIME1, Time[mLabT]); ObjectSet("m.Lab"+y, OBJPROP_PRICE1, mFibs[y]); } return(0); } //+------------------------------------------------------------------+
Change the purple values.
void SetFibs() { double mRange; mP1 = ObjectGet("m.Fib", 1); mP2 = ObjectGet("m.Fib", 3); mRange = MathAbs(mP1 - mP2); if(mP2 < mP1) { //ArraySort(mFibPcnts, WHOLE_ARRAY, 0, MODE_DESCEND); for(int y = 0; y < mArrSize; y++) mFibs[y] = mP1 - mRange * mFibPcnts[y] / 100; } else { //ArraySort(mFibPcnts, WHOLE_ARRAY, 0, MODE_ASCEND); for(y = 0; y < mArrSize; y++) mFibs[y] = mP1 + mRange * mFibPcnts[y] / 100; } if(mT1==0 && mT2==0) { mT1=1; mT2=0; } for(y = 0; y < mArrSize; y++) { ObjectSet("m.Fib"+y, OBJPROP_TIME1, Time[mT1]); ObjectSet("m.Fib"+y, OBJPROP_TIME2, Time[mT2]); ObjectSet("m.Fib"+y, OBJPROP_PRICE1, mFibs[y]); ObjectSet("m.Fib"+y, OBJPROP_PRICE2, mFibs[y]); ObjectSet("m.Fib"+y, OBJPROP_COLOR, mFibCols[y]); ObjectSet("m.Fib"+y, OBJPROP_RAY_RIGHT, true); ObjectSet("m.Fib"+y, OBJPROP_SELECTABLE, false); ObjectSetText("m.Lab"+y, DoubleToStr(mFibPcnts[y], 0) + "% " + DoubleToStr(mFibs[y], Digits), 9, "Arial", mTxtCol); mLabT = MathMin(mT1, mT2); ObjectSet("m.Lab"+y, OBJPROP_TIME1, Time[mLabT]); ObjectSet("m.Lab"+y, OBJPROP_PRICE1, mFibs[y]); ObjectSet("m.Lab"+y, OBJPROP_COLOR, mFibCols[y]); ObjectSet("m.Lab"+y, OBJPROP_ANCHOR, ANCHOR_TOP); ObjectSet("m.Lab"+y, OBJPROP_SELECTABLE, false); } return(0); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi, I would like to extend the lines beyond the "Chart shift limit".
Where is the error in these command strings?
Thanks for the support!