Leonardo A:
I’m working on an MQL5 indicator where I need to check if any of the last 8 daily candles falls on a Sunday. I’m using the TimeDayOfWeek function to determine the day of the week, but I’m encountering the following errors:
- 'TimeDayOfWeek' - undeclared identifier
- time - some operator expected
- (' - unbalanced left parenthesis
There is no such built-in function TimeDayOfWeek. You need to include a library (mqh-file) which defines this function. If you used some example as a reference, look into its source code.
For example, there is one implementation in the algotrading book.
Also there is a more effective implementation specifically for day of week, if you don't need other components of the date:
int DayOfWeek(const datetime time) { return (int)((time / 86400) + 4) % 7; }
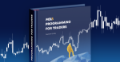
MQL5 Book: Common APIs / Built-in type conversions / Date and time
- www.mql5.com
Values of type datetime intended for storing date and/or time usually undergo several types of conversion: into lines and back to display data to...
Your topic has been moved to the section: Technical Indicators
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Use the API (programmers manual, or documentation if you will)
bool sundayCandlesDetected = false; MqlDateTime dateTime; for (int i = 0; i < 8; i++) { datetime time = iTime(NULL, PERIOD_D1, i); // Convert datetime to MqlDateTime structure TimeToStruct(time, dateTime); if (dateTime.day_of_week == 0) { // 0 represents Sunday sundayCandlesDetected = true; break; // Exit loop if a Sunday candle is detected } }

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi everyone,
I’m working on an MQL5 indicator where I need to check if any of the last 8 daily candles falls on a Sunday. I’m using the TimeDayOfWeek function to determine the day of the week, but I’m encountering the following errors:
Here’s the relevant portion of my code where the error occurs:
I’m using TimeDayOfWeek(time) to check if the returned value is 0 (representing Sunday). However, these errors persist despite reviewing the syntax. I’ve ensured that time is properly initialized with iTime(NULL, PERIOD_D1, i) .
I’ve checked the documentation but haven’t found a clear explanation for these specific errors. Could someone help me understand how to resolve this issue? Is there an alternative to TimeDayOfWeek or any adjustments I could make to get this function to work correctly?
Thank you for your help!