Juergen Aaron Jared Israel Jesse Jean Marie:
2024-10-11 08:42:42,641 INFO worker.py:1786 -- Started a local Ray instance.
Initialized MetaTrader 5
(downloadasset pid=16728) Fetching data for ticker: GBPJPY
(downloadasset pid=16728) No data available for GBPJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDCHF
(downloadasset pid=16728) No data available for USDCHF. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDJPY
(downloadasset pid=16728) No data available for USDJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=31596) Fetching data for ticker: USDCAD
(downloadasset pid=31596) No data available for USDCAD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=12328) Fetching data for ticker: EURUSD
(downloadasset pid=12328) No data available for EURUSD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=33492) Fetching data for ticker: NZDUSD
(downloadasset pid=33492) No data available for NZDUSD. Error: (-10004, 'No IPC connection')Skipping...
Below attached is a simple code. Can you tell me its wrong? Looks straightforward to me.
Cant seem to figure out why I am not getting a good response from the mt5.
2024-10-11 08:42:42,641 INFO worker.py:1786 -- Started a local Ray instance.
Initialized MetaTrader 5
(downloadasset pid=16728) Fetching data for ticker: GBPJPY
(downloadasset pid=16728) No data available for GBPJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDCHF
(downloadasset pid=16728) No data available for USDCHF. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDJPY
(downloadasset pid=16728) No data available for USDJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=31596) Fetching data for ticker: USDCAD
(downloadasset pid=31596) No data available for USDCAD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=12328) Fetching data for ticker: EURUSD
(downloadasset pid=12328) No data available for EURUSD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=33492) Fetching data for ticker: NZDUSD
(downloadasset pid=33492) No data available for NZDUSD. Error: (-10004, 'No IPC connection')Skipping...
Below attached is a simple code. Can you tell me its wrong? Looks straightforward to me.
I know its been a while but I would appreciate the help, thanks alot in advance for the time and consideration.
try and load the rates in mt5 first
this is for testing ? or live ?
Juergen Aaron Jared Israel Jesse Jean Marie:
2024-10-11 08:42:42,641 INFO worker.py:1786 -- Started a local Ray instance.
Initialized MetaTrader 5
(downloadasset pid=16728) Fetching data for ticker: GBPJPY
(downloadasset pid=16728) No data available for GBPJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDCHF
(downloadasset pid=16728) No data available for USDCHF. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDJPY
(downloadasset pid=16728) No data available for USDJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=31596) Fetching data for ticker: USDCAD
(downloadasset pid=31596) No data available for USDCAD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=12328) Fetching data for ticker: EURUSD
(downloadasset pid=12328) No data available for EURUSD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=33492) Fetching data for ticker: NZDUSD
(downloadasset pid=33492) No data available for NZDUSD. Error: (-10004, 'No IPC connection')Skipping...
Below attached is a simple code. Can you tell me its wrong? Looks straightforward to me.
Cant seem to figure out why I am not getting a good response from the mt5.
2024-10-11 08:42:42,641 INFO worker.py:1786 -- Started a local Ray instance.
Initialized MetaTrader 5
(downloadasset pid=16728) Fetching data for ticker: GBPJPY
(downloadasset pid=16728) No data available for GBPJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDCHF
(downloadasset pid=16728) No data available for USDCHF. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDJPY
(downloadasset pid=16728) No data available for USDJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=31596) Fetching data for ticker: USDCAD
(downloadasset pid=31596) No data available for USDCAD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=12328) Fetching data for ticker: EURUSD
(downloadasset pid=12328) No data available for EURUSD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=33492) Fetching data for ticker: NZDUSD
(downloadasset pid=33492) No data available for NZDUSD. Error: (-10004, 'No IPC connection')Skipping...
Below attached is a simple code. Can you tell me its wrong? Looks straightforward to me.
I know its been a while but I would appreciate the help, thanks alot in advance for the time and consideration.
I didn't check your script in depth, but before getting data from a symbol, you need to select it, check the documentation please.
https://www.mql5.com/en/docs/python_metatrader5/mt5symbolselect_py
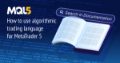
Documentation on MQL5: Python Integration / symbol_select
- www.mql5.com
Select a symbol in the MarketWatch window or remove a symbol from the window. symbol [in] Financial instrument name. Required unnamed...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
2024-10-11 08:42:42,641 INFO worker.py:1786 -- Started a local Ray instance.
Initialized MetaTrader 5
(downloadasset pid=16728) Fetching data for ticker: GBPJPY
(downloadasset pid=16728) No data available for GBPJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDCHF
(downloadasset pid=16728) No data available for USDCHF. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=16728) Fetching data for ticker: USDJPY
(downloadasset pid=16728) No data available for USDJPY. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=31596) Fetching data for ticker: USDCAD
(downloadasset pid=31596) No data available for USDCAD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=12328) Fetching data for ticker: EURUSD
(downloadasset pid=12328) No data available for EURUSD. Error: (-10004, 'No IPC connection')Skipping...
(downloadasset pid=33492) Fetching data for ticker: NZDUSD
(downloadasset pid=33492) No data available for NZDUSD. Error: (-10004, 'No IPC connection')Skipping...
Below attached is a simple code. Can you tell me its wrong? Looks straightforward to me.