It should be:
double stopsLevel = SymbolInfoInteger(_Symbol, SYMBOL_TRADE_STOPS_LEVEL) * _Point;
Please look in the provided documentation
https://www.mql5.com/en/docs/constants/environment_state/marketinfoconstants
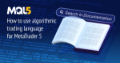
Documentation on MQL5: Constants, Enumerations and Structures / Environment State / Symbol Properties
- www.mql5.com
To obtain the current market information there are several functions: SymbolInfoInteger() , SymbolInfoDouble() and SymbolInfoString() . The first...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi. I can't get rid of these errors at compilation stage. It seems to me that I'm using a correct syntax but if there is an error then I'm definitely doing something wrong. Can you show me where the problem is?
Errors:
'SymbolInfoDouble' - no one of the overloads can be applied to the function call
could be one of 2 function(s)
built-in: double SymbolInfoDouble(const string,ENUM_SYMBOL_INFO_DOUBLE)
built-in: bool SymbolInfoDouble(const string,ENUM_SYMBOL_INFO_DOUBLE,double&)
'SymbolInfoDouble' - no one of the overloads can be applied to the function call
could be one of 2 function(s)
built-in: double SymbolInfoDouble(const string,ENUM_SYMBOL_INFO_DOUBLE)
built-in: bool SymbolInfoDouble(const string,ENUM_SYMBOL_INFO_DOUBLE,double&)