Good morning
Look at
Bars - Timeseries and Indicators Access - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
The function expects parameters between ()... hence the compilation message
Look at
Bars - Timeseries and Indicators Access - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
The function expects parameters between ()... hence the compilation message
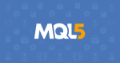
Documentation on MQL5: Timeseries and Indicators Access / Bars
- www.mql5.com
Returns the number of bars count in the history for a specified symbol and period. There are 2 variants of functions calls. Request all of the...
Hi
Change the line 53 to this:
while (!FileIsEnding(file_handle) && i < iBars(Symbol(), PERIOD_CURRENT))
In mql5 there is no function called “Bars” – you need to use full function: iBars(Symbol(), PERIOD_CURRENT)
Best regards

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello everyone,
I am creating a custom indicator for MetaTrader 5, but I am encountering the following compilation error:
Purpose of the Code:
This indicator aims to display backtest balance data on the chart. Specifically, it reads daily balance data from a CSV file resulting from a backtest and displays that balance corresponding to the chart's bars. This allows traders to visually confirm the performance of the backtest.
Code in Question:
mql5
About the Error:
I have checked the location indicated by the error message but could not find any mismatched parentheses. Could anyone provide guidance on what might be causing this issue?
Additional Steps Taken:
Code Structure Review:
Alternative Debugging Methods:
IDE Settings and Environment Check:
Environmental Dependence Issues:
If anyone has encountered a similar issue or can offer insight into possible causes and solutions, it would be greatly appreciated. Additionally, if there are similar indicators or resources that could serve as a reference, please let me know.
Thank you for your assistance.