Please help with MQL5 code for EA which opens a trade automatically once an existing trade is closed.
Please help with MQL5 code for EA which opens a trade automatically once an existing trade is closed.
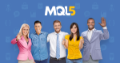
MQL5 forum
- www.mql5.com
MQL5: Forum on automated trading systems and strategy testing
QAISER GILL:
Please help with MQL5 code for EA which opens a trade automatically once an existing trade is closed.
Please help with MQL5 code for EA which opens a trade automatically once an existing trade is closed.
Sample
#property copyright "Your Name" #property link "https://www.example.com" #property version "1.00" #property strict // Input parameters input double Lots = 0.1; // Lot size input int SL = 50; // Stop Loss in pips input int TP = 100; // Take Profit in pips // Global variables bool tradeOpen = false; ulong tradeTicket = 0; // OnInit function int OnInit() { return(INIT_SUCCEEDED); } // OnDeinit function void OnDeinit(const int reason) { } // OnTick function void OnTick() { // Check if a trade is already open if (!tradeOpen) { // Check for trade close event if (PositionsTotal() == 0) { // Open a new trade OpenTrade(); } } } // OpenTrade function void OpenTrade() { MqlTradeRequest request = { 0 }; MqlTradeResult result = { 0 }; // Set the trade request parameters request.action = TRADE_ACTION_DEAL; request.type = ORDER_TYPE_BUY; request.volume = Lots; request.symbol = Symbol(); request.deviation = 10; request.magic = 123456; request.comment = "Auto Trade"; // Calculate the stop loss and take profit levels double ask = SymbolInfoDouble(Symbol(), SYMBOL_ASK); request.price = ask; request.sl = ask - SL * SymbolInfoDouble(Symbol(), SYMBOL_POINT); request.tp = ask + TP * SymbolInfoDouble(Symbol(), SYMBOL_POINT); // Send the trade request if(OrderSend(request, result)) { if(result.retcode == TRADE_RETCODE_DONE) { tradeOpen = true; tradeTicket = result.order; Print("Trade opened successfully. Ticket: ", tradeTicket); } else { Print("Trade failed. Error code: ", result.retcode); } } else { Print("Trade request failed. Error code: ", GetLastError()); } }

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register