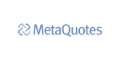
- www.metaquotes.net
- Fractals - Bill Williams' Indicators - Indicators - Charts - MetaTrader 5 for Android
- Fractals - Bill Williams' Indicators - Indicators - Chart - MetaTrader 5 for iPhone
- Fractals - Bill Williams' Indicators - Technical Indicators - Price Charts, Technical and Fundamental Analysis
Hi
This is result of a logic – how fractals are calculated here. As you can see n the code the fractal is detected when we have a local maximum/minimum but to find local extremes, you need to have candles from both sides of the current candle (to check for example if current low price s lower than previous candle and is lower from next candle. And this can be checked only if we have those candles available and closed. In this code there is some extra “confirmation” that this local extreme is the highest/lowest price from two nearby candles – that’s why it requires two candles after to confirm this local extreme price. And hence the signal is after 2nd closed candle (not earlier). I’m not sure how the fractals are calculated on JForex – but they might use some “predictions” or assumptions which can be later “repainted” or recalculated. Here it won’t repaint at all.
Best Regards
Hi guys, I have an issue with an indicator, specifically on JForex. I have an indicator called Fractal. Setting the Fractal to 1, the indicator creates an arrow (when the conditions are met) immediately as the new candle opens. However, with this script I'm trying to modify for MT4, the arrow doesn't appear until at least after 3 candles, that is, on candle 2. Why is that? Can anyone help me out?
If candlesBefore is set to 1, it compares the current high/low with the high/low of the candle preceding it ( i+1 and i-1 ).
If candlesBefore is set to 2, it additionally compares the current high/low with the high/low of the second candle before and after it ( i+2 and i-2 ).
The issue arises from the way the loop is structured and how fractals are identified. Let's consider the case here when candlesBefore is set to 1:
On the first loop iteration, it compares the current candle's high/low with the previous candle's high/low. If the conditions for a fractal are met, it marks the current candle as a fractal.On the second loop iteration ( i-- ), it compares the second candle's high/low with the previous candle's high/low. However, at this point, the previous candle is the one before the one marked as a fractal in the first iteration. Therefore, the indicator waits until the third candle to identify and mark the fractal.
To fix this issue, you can adjust the loop to start from the most recent candle ( i = Bars - 1 ) and iterate backwards. Then, you can compare the current candle with the specified number of candles before it, irrespective of whether a fractal is already identified in previous iterations.
Adjust the loop in the start() function like this, delete everything from the int start and paste this, then compile and test
int start() { int i, nCountedBars; double dCurrent; bool bFound; nCountedBars = IndicatorCounted(); // Start from the most recent candle i = Bars - 1; //Then Iterate backwards while (i >= 2) { // Fractals identification logic //---- Fractals Bullish bFound = false; dCurrent = High[i]; if (candlesBefore == 1) { if(dCurrent > High[i+1] && dCurrent > High[i-1]) { bFound = true; ExtUpFractalsBuffer[i] = dCurrent; } } else if (candlesBefore == 2) { if(dCurrent > High[i+1] && dCurrent > High[i+2] && dCurrent > High[i-1] && dCurrent > High[i-2]) { bFound = true; ExtUpFractalsBuffer[i] = dCurrent; } } //---- Fractals Bearish dCurrent = Low[i]; if(dCurrent < Low[i+1] && dCurrent < Low[i-1]) { bFound = true; ExtDownFractalsBuffer[i] = dCurrent; } // Decrement counter i--; } return (0); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use