if (FileWrite(filehandle, "hellooooo"))
Perhaps you should read the manual. FileWrite - File Functions - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
How To Ask Questions The Smart Way. (2004)
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up.
FileWrite does not return a boolean.
Certainly! Reading and writing CSV files in Python is a common task. Here's a basic example using the built-in `csv` module:
### Reading CSV:
```python
import csv
# Specify the path to your CSV file
csv_file_path = 'path/to/your/file.csv'
# Open the CSV file for reading
with open(csv_file_path, 'r') as file:
# Create a CSV reader object
csv_reader = csv.reader(file)
# Iterate through each row in the CSV file
for row in csv_reader:
# Each 'row' is a list representing a row in the CSV
print(row)
```
### Writing CSV:
```python
import csv
# Specify the path to your CSV file for writing
csv_file_path = 'path/to/your/new_file.csv'
# Data to be written to the CSV file
data_to_write = [
['Name', 'Age', 'Country'],
['John Doe', 25, 'USA'],
['Jane Smith', 30, 'Canada'],
# Add more rows as needed
]
# Open the CSV file for writing
with open(csv_file_path, 'w', newline='') as file:
# Create a CSV writer object
csv_writer = csv.writer(file)
# Write the data to the CSV file
csv_writer.writerows(data_to_write)
print("CSV file has been written successfully.")
```
Make sure to replace `'path/to/your/file.csv'` and `'path/to/your/new_file.csv'` with the actual paths to your existing CSV file and the new file you want to create, respectively.
This is a simple example, and you might need to adapt it based on the structure of your CSV data. Also, remember that error handling is important in real-world applications to handle cases where the file might not exist or have unexpected content.
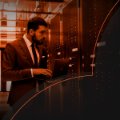
Bammtech
- bammtech.com
Best ingredients for Cybersecurity, High-Performance Computing and Networking Solutions.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
this is one of many attempts.
\AppData\Roaming\MetaQuotes\Terminal\D0E8209F77C8CF37AD8BF550E51FF075\MQL5\Files
This is where "Book.csv" is located and I've also tried using,
AppData\Roaming\MetaQuotes\Terminal\Common \Files
Going the long way and using TERMINAL_DATA_PATH hasn't helped.
I don't think finding the document is the problem anymore since I'm not getting any 5002 or 5004 errors. The filehandle returns 1, but the document remains completely empty.
I am lost but hopefully someone here can steer me in the right direction.
Thanks in advance.