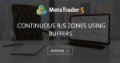
- 2023.02.04
- www.mql5.com
Hi Yashar,
I think what I am struggling with - is how to even paint those R/S zones using buffers:
< Looks like I cannot embed the picture itself, but I have attached it in the above post >
For example - if I want to plot two lines, I can specify two buffers ahead of time. But what if I want to plot N amount of lines which i cannot specify ahead of time?
Can I set a dynamic amount of buffers or am I able to capture the say two price lines using one buffer?
Hey all,
I have developed a function to plot prices of interest - however it uses ObjectCreate() which does not visualise nicely when backtesting, so I am in the process of creating it as an indicator. The problem is that the number of prices/levels it returns is variable depending on its parameters, making it problematic to transform into an indicator.
I would want these to plot across the whole screen as a horizontial trendline - such that there are no "breaks" between the prices.
Suppose my prices of interest are
My questions are:
1. Is it possible to create a variable amount of buffers ( and therefore, arrays ) which may hold the price levels I am interested in.
* E.g. Am I able to declare the number of indicator buffers ( in this case , 3 ) after the someFunction runs?
I assume not, given it needs to be instantiated with #property from what I've seen online.
2. Otherwise, am I able to plot more than one horizontal line ( say 0.65380 and 0.66380 - though it may be extended up to 50-100) using only one or two indicator buffers?
I've attached some dummy code as to what I have attempted - it looks like we need to set up a new indicator buffer for each line I am interested in?
Which results in :
However, how could I extend this to a variable number of prices I calculate?
Let me know of any tips, much appreciated!
Thanks Dominik - given this then, I would have to intialize 511 ( empty ) arrays, check how many prices/zones I'd like to plot and then fill them in?
Is that the best way around this?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hey all,
I have developed a function to plot prices of interest - however it uses ObjectCreate() which does not visualise nicely when backtesting, so I am in the process of creating it as an indicator. The problem is that the number of prices/levels it returns is variable depending on its parameters, making it problematic to transform into an indicator.
I would want these to plot across the whole screen as a horizontial trendline - such that there are no "breaks" between the prices.
Suppose my prices of interest are
[0.65380, 0.66380, etc]
My questions are:
1. Is it possible to create a variable amount of buffers ( and therefore, arrays ) which may hold the price levels I am interested in.
* E.g. Am I able to declare the number of indicator buffers ( in this case , 3 ) after the someFunction runs?
I assume not, given it needs to be instantiated with #property from what I've seen online.
2. Otherwise, am I able to plot more than one horizontal line ( say 0.65380 and 0.66380 - though it may be extended up to 50-100) using only one or two indicator buffers?
I've attached some dummy code as to what I have attempted - it looks like we need to set up a new indicator buffer for each line I am interested in?
Which results in :
However, how could I extend this to a variable number of prices I calculate?
Let me know of any tips, much appreciated!