Your topic has been moved to the section: Expert Advisors and Automated Trading
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Fernando Carreiro #:
Your topic has been moved to the section: Expert Advisors and Automated Trading
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Your topic has been moved to the section: Expert Advisors and Automated Trading
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
No problem Fernando thanks for the correction, do you have an answer?
Vincent Kimathi Baariu:
I wrote the code below to get news from calendar of certain symbols but I am getting Error:
Error! Failed to get events of country_code = EUR
Thanks in advance for your help
Haven't tested your code,
But from a quick-look I think this might be your issue-
You are passing the 'currency' as the 'country code' input parameter of the 'CalendarValueHistory' function.
Vincent Kimathi Baariu #: How should it be?
Please read the documentation ... Documentation on MQL5: Economic Calendar / CalendarValueHistory
int CalendarValueHistory( MqlCalendarValue& values[], // array for value descriptions datetime datetime_from, // left border of a time range datetime datetime_to=0 // right border of a time range const string country_code=NULL, // country code name (ISO 3166-1 alpha-2) const string currency=NULL // country currency code name );
The 4th parameter is the country code, and the 5th parameter is the currency.
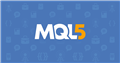
Documentation on MQL5: Economic Calendar / CalendarValueHistory
- www.mql5.com
CalendarValueHistory - Economic Calendar - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I wrote the code below to get news from calendar of certain symbols but I am getting Error:
Error! Failed to get events of country_code = EUR
Thanks in advance for your help