Dominik Egert:
Check these topics:
Thanks Dominik Egert for the test provided. I guess this thread is mainly test data.
I'm here to provide my test results on i7-11th CPU on X64, AVX, AVX2, AVX512.
Not much difference on AVX versions even retested several times, but any version of AVX definitely better than X64 in some aspect.
X64 Regular: (209, 317, 195)
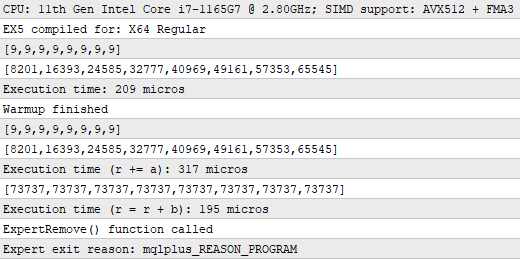
AVX: (163, 92, 172)
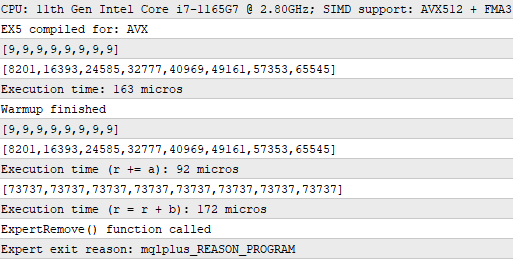
AVX2: (198, 95, 181)
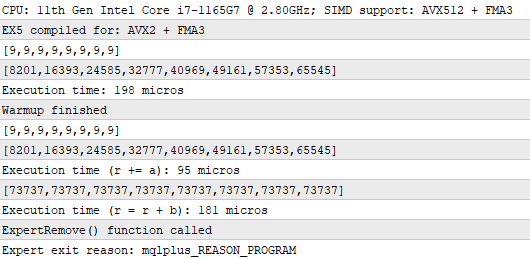
AVX512: (201, 94, 177)
I'm here to provide my test results on i7-11th CPU on X64, AVX, AVX2, AVX512.
Not much difference on AVX versions even retested several times, but any version of AVX definitely better than X64 in some aspect.
CPU | Warmup | r += a | r = r + b |
---|---|---|---|
X64 | 209 | 317 | 195 |
AVX | 163 | 92 | 172 |
AVX2 | 198 | 95 | 181 |
AVX512 | 207 | 94 | 177 |
X64 Regular: (209, 317, 195)
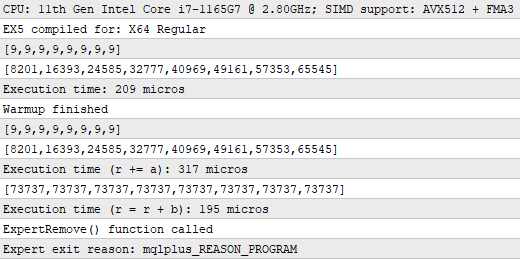
AVX: (163, 92, 172)
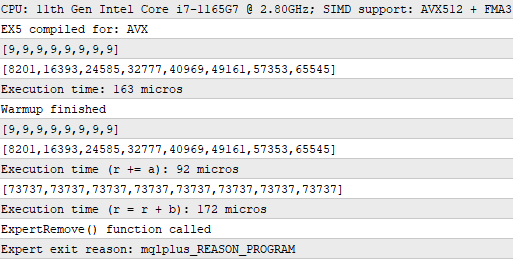
AVX2: (198, 95, 181)
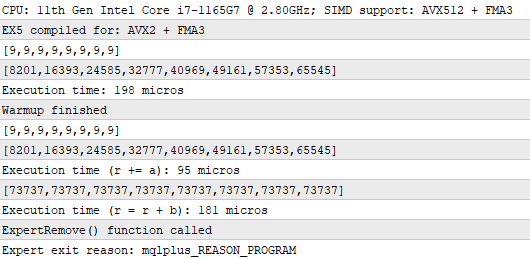
AVX512: (201, 94, 177)
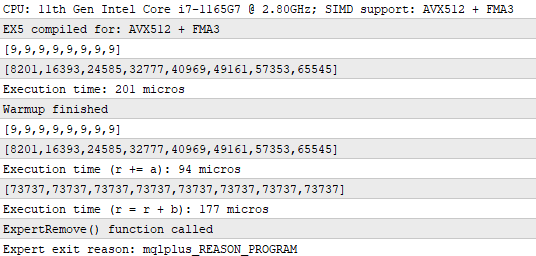

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello there,
I've been experimenting with AVX (and its derivatives AVX2 + AVX512) support in MQL5. - Assuming, and to some extend verified, AVX is being utilized by the compiler, if enabled, we should be able to see significant speed increase on some types of operations. - Due to the lack of documentation, I have taken simple assumptions, required to make any approach to "get access" to SIMD (Single Instruction Multiple Data == AVX) operations.
In this case I focused on a simple vectorf data type, since it seemse to be sensible to assume, this data type has the closest possibility to be utilized as an input to a SIMD operation. - To proof actually the compiler uses SIMD, I have written following code:
By the print out of the results, I think I actually made the compiler use (at least) once SIMD instructions to optimize the operations. - Actually it is impossible to identify and validate this, but given the speedup, it should not be related to efficient-core/performance-core differences.
The results measured had an increase in speed, though I am not able to make out how it actually works, or better said, how the compiler applies SIMD optimizations.
See screenshot:
These are the results of 4 different runs, each freshly compiled with the desired optimization. It can be seen in the third run, the optimization was actually applied by the compiler. If you take a look at the second run, the optimization is only partially applied, obviously.
I would like to get some more insight into this subject, as it is currently a more or less an undocumented feature, and there is no way to figure out how to utilize this feature set provided by the CPUs.
Some feedback by an MQL dev with more insight would be very nice. - Currently I have the impression, this feature is more or less broken, as it is.
Also as a sidenote, AVX2 inherently had been released to add support for integer data types, which is not available through mql5 vector data type. - Also it would be nice to know how to gain access to the available SIMD set of instructions in a determined way.
PS: An enumeration for the CPU architecture would be a nice addition as well.
- Here is a manual and incomplete implementation of such: