I am new in MQL5. I am struggling with writing a function to get last open trade ticket for a specific type and Open price of that ticket. Appreciate any help.
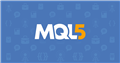
Documentation on MQL5: Constants, Enumerations and Structures / Indicator Constants / Price Constants
- www.mql5.com
Price Constants - Indicator Constants - Constants, Enumerations and Structures - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
Shakhrukh Bobojanov:
I am new in MQL5. I am struggling with writing a function to get last open trade ticket for a specific type and Open price of that ticket. Appreciate any help.
You are comparing position type with order type.
I am new in MQL5. I am struggling with writing a function to get last open trade ticket for a specific type and Open price of that ticket. Appreciate any help.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register