//if (StringToDouble(val)) //How can one detect a data type here ???
The problem I found with StringToDouble() is that it will return 0.0 for incorrect strings - no easy way of knowing whether it is pass/fail.
For example:
string test = "AB.12"; double result = StringToDouble(test); PrintFormat("%s = %f", test, result);
Outputs:
AB.12 = 0.000000
So I think you need to write your own utils to check the strings in detail - I have done that, not just in MQL5 but other languages too whenever I find the standard tools omit something I need.
This thread has some good examples which may help:
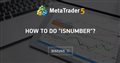
- 2014.06.09
- www.mql5.com
Does anyone know a way to detect a datatype from a string value?
This can be important when reading from a CSV file, as we all know mql5 reads a csv file as a data of one type it, for example reading the information from a CSV as strings which works better and gives you the option to convert the data loaded from a file into whatever datatype you intend to, Just like using pandas.read_csv
I would like to be able to detect the datatype from a string which could have data in different formats like:
Something like this,
You can use MqlParam to detect the datatype from a string.
I just found an example for reading file: https://www.mql5.com/en/code/24777

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Does anyone know a way to detect a datatype from a string value?
This can be important when reading from a CSV file, as we all know mql5 reads a csv file as a data of one type it, for example reading the information from a CSV as strings which works better and gives you the option to convert the data loaded from a file into whatever datatype you intend to, Just like using pandas.read_csv
I would like to be able to detect the datatype from a string which could have data in different formats like:
Something like this,