We are not going to count lines for you.
Please identify the lines by highlighting them in your post.
Assuming it is the lines ...
if(PositionSelect(ticket)) {if(PositionSelect(ticket)) // Select the position by its ticket for further work
... then please note that you are using the wrong function.
It should be PositionSelectByTicket and not PositionSelect.
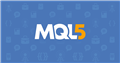
Documentation on MQL5: Trade Functions / PositionSelectByTicket
- www.mql5.com
PositionSelectByTicket - Trade Functions - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I'm getting the below 2 warnings when I compile my expert advisor. Can someone please point me in the right direction to get the 2 functions working
implicit conversion from 'number' to 'string' TrendBotV2.mq5 68 27
implicit conversion from 'number' to 'string' TrendBotV2.mq5 116 25