Did you lookup the meaning of the error code?
ERR_HISTORY_NOT_FOUND
4401
Requested history not found
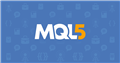
Documentation on MQL5: Constants, Enumerations and Structures / Codes of Errors and Warnings / Runtime Errors
- www.mql5.com
Runtime Errors - Codes of Errors and Warnings - Constants, Enumerations and Structures - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
Arpit T:
Both failed when i try to load in M1 chart and want to copy D1 date. Error 4401
I know someone is going to refer me to read this
but this is also not working as i want to copy open every time on OnCalculate()
//+------------------------------------------------------------------+ //| test_newcandle.mq5 | //| Copyright 2022 | //| https://www.mql5.com/ | //+------------------------------------------------------------------+ #property copyright "Copyright 2022" #property link "https://www.mql5.com/" #property version "1.00" #property indicator_chart_window #property indicator_plots 0 input string InpLoadedSymbol="a_SOLUSDT"; // Symbol to be load input ENUM_TIMEFRAMES InpLoadedPeriod=PERIOD_D1; // Period to be loaded input datetime InpStartDate=D'2006.01.01'; // Start date //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- //Method 1 // MqlRates rates[]; // ZeroMemory(rates); // ArraySetAsSeries(rates,true); // // // int copied=CopyRates(Symbol(),PERIOD_D1,0,1,rates); // if(copied<0) // Print("Failed to get history data for the symbol ",Symbol(), " err: " + IntegerToString(GetLastError())); // // // //Method 2 // double iH[]; // if(CopyOpen(Symbol(),PERIOD_D1,0,1,iH)==-1) // Print("Failed to get open data for the symbol ",Symbol(), " err: " + IntegerToString(GetLastError())); Print("Start load",InpLoadedSymbol+","+GetPeriodName(InpLoadedPeriod),"from",InpStartDate); //--- int res=CheckLoadHistory(InpLoadedSymbol,InpLoadedPeriod,InpStartDate); switch(res) { case -1 : Print("Unknown symbol ",InpLoadedSymbol); break; case -2 : Print("Requested bars more than max bars in chart"); break; case -3 : Print("Program was stopped"); break; case -4 : Print("Indicator shouldn't load its own data"); break; case -5 : Print("Load failed"); break; case 0 : Print("Loaded OK"); break; case 1 : Print("Loaded previously"); break; case 2 : Print("Loaded previously and built"); break; default : Print("Unknown result"); } //--- datetime first_date; SeriesInfoInteger(InpLoadedSymbol,InpLoadedPeriod,SERIES_FIRSTDATE,first_date); int bars=Bars(InpLoadedSymbol,InpLoadedPeriod); Print("First date ",first_date," - ",bars," bars"); double iH[]; if(CopyOpen(Symbol(),PERIOD_D1,0,1,iH)==-1) Print("Failed to get open data for the symbol ",Symbol(), " err: " + IntegerToString(GetLastError())); else Print(iH[0]); //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int CheckLoadHistory(string symbol,ENUM_TIMEFRAMES period,datetime start_date) { datetime first_date=0; datetime times[100]; //--- check symbol & period if(symbol==NULL || symbol=="") symbol=Symbol(); if(period==PERIOD_CURRENT) period=Period(); //--- check if symbol is selected in the Market Watch if(!SymbolInfoInteger(symbol,SYMBOL_SELECT)) { if(GetLastError()==ERR_MARKET_UNKNOWN_SYMBOL) return(-1); SymbolSelect(symbol,true); } //--- check if data is present SeriesInfoInteger(symbol,period,SERIES_FIRSTDATE,first_date); if(first_date>0 && first_date<=start_date) return(1); //--- don't ask for load of its own data if it is an indicator if(MQL5InfoInteger(MQL5_PROGRAM_TYPE)==PROGRAM_INDICATOR && Period()==period && Symbol()==symbol) return(-4); //--- second attempt if(SeriesInfoInteger(symbol,PERIOD_M1,SERIES_TERMINAL_FIRSTDATE,first_date)) { //--- there is loaded data to build timeseries if(first_date>0) { //--- force timeseries build CopyTime(symbol,period,first_date+PeriodSeconds(period),1,times); //--- check date if(SeriesInfoInteger(symbol,period,SERIES_FIRSTDATE,first_date)) if(first_date>0 && first_date<=start_date) return(2); } } //--- max bars in chart from terminal options int max_bars=TerminalInfoInteger(TERMINAL_MAXBARS); //--- load symbol history info datetime first_server_date=0; while(!SeriesInfoInteger(symbol,PERIOD_M1,SERIES_SERVER_FIRSTDATE,first_server_date) && !IsStopped()) Sleep(5); //--- fix start date for loading if(first_server_date>start_date) start_date=first_server_date; if(first_date>0 && first_date<first_server_date) Print("Warning: first server date ",first_server_date," for ",symbol, " does not match to first series date ",first_date); //--- load data step by step int fail_cnt=0; while(!IsStopped()) { //--- wait for timeseries build while(!SeriesInfoInteger(symbol,period,SERIES_SYNCHRONIZED) && !IsStopped()) Sleep(5); //--- ask for built bars int bars=Bars(symbol,period); if(bars>0) { if(bars>=max_bars) return(-2); //--- ask for first date if(SeriesInfoInteger(symbol,period,SERIES_FIRSTDATE,first_date)) if(first_date>0 && first_date<=start_date) return(0); } //--- copying of next part forces data loading int copied=CopyTime(symbol,period,bars,100,times); if(copied>0) { //--- check for data if(times[0]<=start_date) return(0); if(bars+copied>=max_bars) return(-2); fail_cnt=0; } else { //--- no more than 100 failed attempts fail_cnt++; if(fail_cnt>=100) return(-5); Sleep(10); } } //--- stopped return(-3); } //+------------------------------------------------------------------+ //| Returns string value of the period | //+------------------------------------------------------------------+ string GetPeriodName(ENUM_TIMEFRAMES period) { if(period==PERIOD_CURRENT) period=Period(); //--- switch(period) { case PERIOD_M1: return("M1"); case PERIOD_M2: return("M2"); case PERIOD_M3: return("M3"); case PERIOD_M4: return("M4"); case PERIOD_M5: return("M5"); case PERIOD_M6: return("M6"); case PERIOD_M10: return("M10"); case PERIOD_M12: return("M12"); case PERIOD_M15: return("M15"); case PERIOD_M20: return("M20"); case PERIOD_M30: return("M30"); case PERIOD_H1: return("H1"); case PERIOD_H2: return("H2"); case PERIOD_H3: return("H3"); case PERIOD_H4: return("H4"); case PERIOD_H6: return("H6"); case PERIOD_H8: return("H8"); case PERIOD_H12: return("H12"); case PERIOD_D1: return("Daily"); case PERIOD_W1: return("Weekly"); case PERIOD_MN1: return("Monthly"); } //--- return("unknown period"); } //+------------------------------------------------------------------+
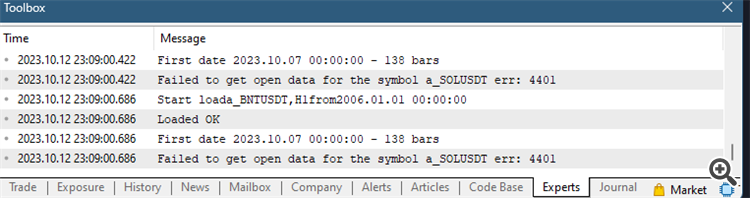
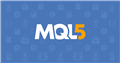
Documentation on MQL5: Timeseries and Indicators Access / Organizing Data Access
- www.mql5.com
Organizing Data Access - Timeseries and Indicators Access - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
Fernando Carreiro #:
Did you lookup the meaning of the error code?
Did you lookup the meaning of the error code?
ERR_HISTORY_NOT_FOUND
4401
Requested history not found
History do exist, and it also works for 1st run on script if i use CheckLoadHistory()
but my question is about copying to everytime on Oncalculate function
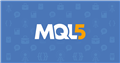
Documentation on MQL5: Timeseries and Indicators Access / Organizing Data Access
- www.mql5.com
Organizing Data Access - Timeseries and Indicators Access - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Both failed when i try to load in M1 chart and want to copy D1 date. Error 4401