- Creating an Array of object, getting error
- Adding value to array
- Any questions from newcomers on MQL4 and MQL5, help and discussion on algorithms and codes
Sorry for the simple question, I am very new to MQL5. I know how to create a generic array, but am now trying to create a list of undefined size, which values can be added to. Most of the literature I read seemed to go over my head
Read the documentation for Standard Library -> Data Collections
There you will find CList, CArray, and CTree.
Also, read the documentation for the whole standard library.
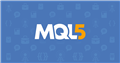
- www.mql5.com
Sorry for the simple question, I am very new to MQL5. I know how to create a generic array, but am now trying to create a list of undefined size, which values can be added to. Most of the literature I read seemed to go over my head
Besides @Drazen Penic 's points you can do it yourself too .
Let's say you want a one type solution so as to not mix templates into the example.
long tickets[];
That is your list of tickets with an unknown size
You need 3 things
Adding to your list
//you receive the array where you want to add the item and its value in the function int add(long &array[],long add_this){ //calculate the new size of the array int new_size=ArraySize(array)+1; //apply the new size ArrayResize(array,new_size,0); //fill in the new element which sits at size-1 array[new_size-1]=add_this; //and return the address of the new element or the total elements return(new_size-1); }
Removing from your list
//you send the array and the index of the item you want to remove int remove_unordered(long &array[],int remove_index){ //you calculate the new size int new_size=ArraySize(array)-1; //now the new size points to the last element as well , interesting //so take the last element and place it in the index you no longer need array[remove_index]=array[new_size];//because new size points to the last element //aand shrink it ArrayResize(array,new_size,0); //return the size return(new_size); }
And the most fun of all , finding one item in your list
//you provide the array and what value you are looking for int find(long &array[],long find_this){ for(int i=0;i<ArraySize(array);i++){ //if the value matches what you are looking for return the index if(array[i]==find_this){ return(i); } } //you return -1 if you find nothing return(-1); }
So in a use scenario you would add a ticket like this :
//you send the name of the array , tickets , and the ticket add(tickets,4235315631);
and so on
Besides @Drazen Penic 's points you can do it yourself too .
Let's say you want a one type solution so as to not mix templates into the example.
That is your list of tickets with an unknown size
You need 3 things
Adding to your list
Removing from your list
And the most fun of all , finding one item in your list
So in a use scenario you would add a ticket like this :
and so on
Exactly the kind of help I was looking for. Thank you very much!!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use