Here is the basic maths — use positive volume for "buy" and negative volume for "sell":
For your example, and using the maths shown above, the Net Mean Open Price (or break even) is at 300, which is the net equivalent of a single "buy" position of 1 lot at that price.
For your example, and using the maths shown above, the Net Mean Open Price (or break even) is at 300, which is the net equivalent of a single "buy" position of 1 lot at that price.
Awesome thank you,
I don't suppose you know of any code I could paste into mt5 that would run this equation for my open positions. I've been scouring the web for it for days with no luck. I unfortunately lack the skills as of yet to write it myself.
- Usually people who can't code don't receive free help on this forum.
- If you show your attempts and describe your problem clearly, you will most probably receive an answer from the community. Use the CODE button (Alt-S) when inserting code.
- To learn MQL programming, you can research the many available Articles on the subject, or examples in the Codebase, as well as reference the online Documentation.
- If you do not want to learn to code, that is not a problem. You can either look at the Codebase if something free already exists, or in the Market for paid products (also sometimes free). However, recommendations or suggestions for Market products are not allowed on the forum, so you will have to do your own research.
- Finally, you also have the option to hire a programmer in the Freelance section.
- Usually people who can't code don't receive free help on this forum.
- If you show your attempts and describe your problem clearly, you will most probably receive an answer from the community. Use the CODE button (Alt-S) when inserting code.
- To learn MQL programming, you can research the many available Articles on the subject, or examples in the Codebase, as well as reference the online Documentation.
- If you do not want to learn to code, that is not a problem. You can either look at the Codebase if something free already exists, or in the Market for paid products (also sometimes free). However, recommendations or suggestions for Market products are not allowed on the forum, so you will have to do your own research.
- Finally, you also have the option to hire a programmer in the Freelance section.
int OnInit() { double totalPositionSize = 0.0; double weightedSum = 0.0; ulong tickets[]; for (int i = 0; i < PositionsTotal(); i++) { ulong ticket = PositionGetTicket(i); ArrayResize(tickets, i+1); tickets[i] = ticket; } for (int i = 0; i < ArraySize(tickets); i++) { double entryPrice = PositionGetDouble(POSITION_PRICE_OPEN, tickets[i]); double positionSize = PositionGetDouble(POSITION_VOLUME, tickets[i]); weightedSum += entryPrice * positionSize; totalPositionSize += positionSize; } double averagePrice = totalPositionSize > 0 ? weightedSum / totalPositionSize : 0.0; Print("Average price of all positions: ", averagePrice); return(INIT_SUCCEEDED); } void OnDeinit(const int reason) { }
Here's what I was trying to use but it wouldn't compile with the following errors.
'PositionGetDouble' - no one of the overloads can be applied to the function call
could be one of 2 function(s)
built-in: double PositionGetDouble(ENUM_POSITION_PROPERTY_DOUBLE)
built-in: bool PositionGetDouble(ENUM_POSITION_PROPERTY_DOUBLE,double&)
'PositionGetDouble' - no one of the overloads can be applied to the function call
could be one of 2 function(s)
built-in: double PositionGetDouble(ENUM_POSITION_PROPERTY_DOUBLE)
built-in: bool PositionGetDouble(ENUM_POSITION_PROPERTY_DOUBLE,double&)
How about reading the documentation? — Documentation on MQL5: Trade Functions / PositionGetDouble
There is no "ticket number" for any of its parameters. The position needs to be selected before the function can be used, as explained in the documentation.
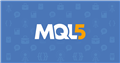
- www.mql5.com
Also remember that you will need to apply a negative volume for "sell" positions, and you will have to group the positions by symbol.
PS! Also, don't put everything in the OnInit. That event handler is only for initialisations, not for carrying out procedures.
Forum on trading, automated trading systems and testing trading strategies
how to calculate break even price for buy and sell positions
Fernando Carreiro, 2023.09.15 07:36
Also remember that you will need to apply a negative volume for "sell" positions, and you will have to group the positions by symbol.
PS! Also, don't put everything in the OnInit. That event handler is only for initialisations, not for carrying out procedures.
//+------------------------------------------------------------------+ //| Position average price | //+------------------------------------------------------------------+ double PositionAveragePrice(const string symbol) { //--- For calculating the average price double sum_mult =0.0; double sum_volumes =0.0; //--- Check if there is a position with specified properties for(int i=PositionsTotal()-1; i>=0; i--) { if(symbol!=PositionGetSymbol(i)) continue; //--- Get the price and position volume double pos_price =PositionGetDouble(POSITION_PRICE_OPEN); double pos_volume =PositionGetDouble(POSITION_VOLUME); //--- Sum up the intermediate values pos_volume = PositionGetInteger(POSITION_TYPE) ? -pos_volume : pos_volume; sum_mult+=(pos_price*pos_volume); sum_volumes+=pos_volume; } //--- Prevent division by zero if(sum_volumes==0) return(0.0); //--- Return the average price return(sum_mult/sum_volumes); } //+------------------------------------------------------------------+ //| Script start function | //+------------------------------------------------------------------+ void OnStart() { double average_price =PositionAveragePrice(Symbol()); Print("Average price of all positions: ", average_price); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
In a hedge account what would be the formula to calculate the break even price of my buy and sell positions (of one instrument)
for example if I bought 3 lots at 200 and sold 2 lots at 150 how would I calculate where my break even level is for all 5 positions.
obviously if I had an equal number of buy and sell positions there would be no break even level.