Your Last() function does not return the last, it returns the first in the list. You need to find the last close and return that. Your loop keeps going,.
MT4:
-
Do not assume history has only closed orders.
OrderType() == 6, 7 in the history pool? - MQL4 programming forum #4 and #5 (2017) -
Do not assume history is ordered by date, it's not.
Could EA Really Live By Order_History Alone? (ubzen) - MQL4 programming forum (2012)
Taking the last profit and storing it in a variable | MQL4 - MQL4 programming forum #3 (2020) -
Total Profit is OrderProfit() + OrderSwap() + OrderCommission(). Some brokers don't use the Commission/Swap fields. Instead, they add balance entries. (Maybe related to Government required accounting/tax laws.)
"balance" orders in account history - Day Trading Techniques - MQL4 programming forum (2017)Broker History FXCM Commission - «TICKET»
Rollover - «TICKET»>R/O - 1,000 EUR/USD @0.52 #«ticket» N/A OANDA Balance update
Financing (Swap: One entry for all open orders.)
Thank you!
It finally work!
Here the new code:
extern int Serie_Pos=3; //average of consecutive trades closed with profit int x=-1; //number of consecutive positive trades double MM_Lots() //money managment function { double Lots; if (Last()<=0 || x==Serie_Pos) //if last trade closed with a loss or consecutive positive trades equals average positive trades, lots equal minimal lots { x=0; Lots=Lots_min; } else { if (Last()>0 && x<Serie_Pos) //if last trade closed with profit and cosecutive positive trades are less than average positive trades, lots=minimal lots * 2^x { x++; Lots=Lots_min*pow(2,x); } } return (Lots); } datetime last_time = 0; double last_profit = 0; double Last() { for(int i=OrdersHistoryTotal(); i>0; i--) { if(OrderSelect(i,SELECT_BY_POS,MODE_HISTORY)) { if(OrderCloseTime()>last_time) { last_time = OrderCloseTime(); { last_profit = OrderProfit(); } } } } return (last_profit); }
I used the code provided by Marco vd Heijden in this thread https://www.mql5.com/en/forum/343360#comment_16784083 (thank you Marco!) and now everything work as i wanted!
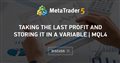
- 2020.06.08
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi everybody! I'm new with coding in mql4 and i'm having some issues while trying to implement money managment rules in my EA.
Here is the code:
It seems like the EA can't get the last trade result because if i remove the function Last() from the function MM_Lots(), the number of lots change like i want it to do.
I want to precise that all the code compile with no errors in Metaeditor and the EA run in Metatrader.
Do you have any clue where is the problem (exept, of course, between the chair and the keyboard)?
Many thanks.