The function
void OnStart()
features either a script or a service. EA must have OnTick.
Maybe you should start with this:
If you place the cursor on an MQL function and press F1, you will see the reference directly, many with examples to copy and paste - the fastest form to code.
https://www.mql5.com/en/articles/496
https://www.mql5.com/en/articles/100
and for debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
https://www.mql5.com/en/search#!keyword=cookbook
"Bear in mind there's virtually nothing that hasn't already been programmed for MT4/MT5 and is ready for you - so searching gives better results than AI or ChatGPT!" (Carl Schreiber):
=> Search in the articles: https://www.mql5.com/en/articles
=> Search in the codebase: https://www.mql5.com/en/code
=> Search in general: https://www.mql5.com/en/search or via Google with: "site:mql5.com .." (forgives misspelling)
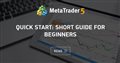
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
When I try to add this EA to the chart I get a message same "is not an expert and cannot be executed".
Can someone shed some light on what is wrong here?