Your topic has been moved to the section: MQL4 e MetaTrader 4 — In the future, please consider which section is most appropriate for your query.
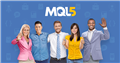
MQL5 forum: Expert Advisors and Automated Trading
- www.mql5.com
How to create an Expert Advisor (a trading robot) for Forex trading
bool NoTradesToday() { datetime today = iTime(NULL,Period(),0); for(int i=OrdersHistoryTotal()-1; i>=0; i--) { if(!OrderSelect(i,SELECT_BY_POS,MODE_HISTORY)) continue; if(OrderSymbol() != _Symbol) continue; if(OrderMagicNumber() != InpMagicNumber) continue; if(OrderOpenTime() >= today) return(false); } for(int i=OrdersTotal()-1; i>=0; i--) { if(!OrderSelect(i,SELECT_BY_POS)) continue; if(OrderSymbol() != _Symbol) continue; if(OrderMagicNumber() != InpMagicNumber) continue; if(OrderOpenTime() >= today) return(false); } return(true); }
use
datetime today = iTime(NULL,PERIOD_D1,0);

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Good afternoon everybody,
I recently created an EA and is working exactly as expected. The signals are being delivered correctly and it is opening them accordingly. Everything is perfect.
Now, there is one fault which I already have it contemplated on my manual trading and it is that it only has to open one trade a day. ONLY ONE TRADE.
Due to this, the performance of my EA is not as good as the manual trading. I would like to add this condition.
I will attach the code in here: