Hi everyone,
I need some help with my attempt at coding a custom indicator to help me trading using the Ichimoku Kinko Hyo.
The idea is to draw 5 different ZigZag on the chart, all based on the Ichimoku Kinko Hyo.
ZigZag 1 = based on the tenkansen line (range of 9 periods/bars) ; everytime the line changes direction, a new top or bottom is marked, thus the ZigZag is created.
ZigZag 2 = based on the kijunsen line (range of 26 periods/bars)
ZigZag 3 = based on the crossings of tenkansen and kijunsen or Senkou Span A (range of 17 periods/bars)
ZigZag 4 = based on the Senkou Span B (range of 52 periods/bars)
ZigZag 5 = based on the crossings of SSA/SSB (range of 34.5 periods/bars)
I started from the ZigZag indicator example; and tweaked/kept only what I needed to create a ZigZag that would follow the change of direction of the Tenkansen. It seems like I didn't need to keep the whole ZigZag system based on Depth, Deviation and backstep. I kept only the Depth, and deleted everything related to deviation and backstep. It resulted in a ZigZag that was following the change of direction of the Tenkansen as I needed.
From there, I expected to have nothing much to do to plot a second ZigZag that would follow the change of direction of the Kijunsen instead of the Tenkansen; I copy/pasted every integer and oncalculate code from the Tenkan ZigZag and modified it so that the Kijun ZigZag has its own integer, buffers and so on.
Once done, unfortunately, the ZigZag created by my code for the Kijunsen is wrong.
On this picture, you can see the Tenkan ZigZag following the change of direction of the Tenkansen properly:
On the next one, you can see the incorrect Kijun ZigZag with the Kijunsen on which the ZigZag is supposed to be based:
And finally, both ZigZag drawn on the chart:
I played around with my code to try and find out where the issue lied but to no avail. So I came to you all for help. Here is the current code I'm using:
Once I manage to get the Kijun ZigZag working, the next step will be to do the same for the 3 other ZigZags, and add buttons on chart to show/hide each zigzag. However, for now, I'm stuck and cannot get that second ZigZag plotting to be populated correctly.
If you have any idea/hypotheses/solutions on this issue, I'll try them all!
Thanks in advance,
basic

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi everyone,
I need some help with my attempt at coding a custom indicator to help me trading using the Ichimoku Kinko Hyo.
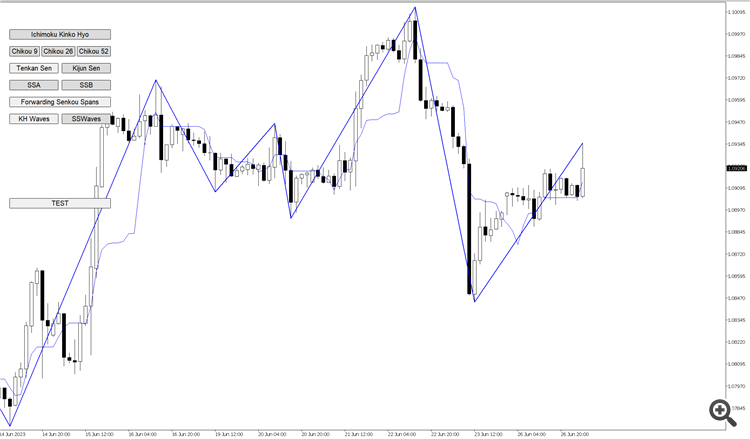
The idea is to draw 5 different ZigZag on the chart, all based on the Ichimoku Kinko Hyo.
ZigZag 1 = based on the tenkansen line (range of 9 periods/bars) ; everytime the line changes direction, a new top or bottom is marked, thus the ZigZag is created.
ZigZag 2 = based on the kijunsen line (range of 26 periods/bars)
ZigZag 3 = based on the crossings of tenkansen and kijunsen or Senkou Span A (range of 17 periods/bars)
ZigZag 4 = based on the Senkou Span B (range of 52 periods/bars)
ZigZag 5 = based on the crossings of SSA/SSB (range of 34.5 periods/bars)
I started from the ZigZag indicator example; and tweaked/kept only what I needed to create a ZigZag that would follow the change of direction of the Tenkansen. It seems like I didn't need to keep the whole ZigZag system based on Depth, Deviation and backstep. I kept only the Depth, and deleted everything related to deviation and backstep. It resulted in a ZigZag that was following the change of direction of the Tenkansen as I needed.
From there, I expected to have nothing much to do to plot a second ZigZag that would follow the change of direction of the Kijunsen instead of the Tenkansen; I copy/pasted every integer and oncalculate code from the Tenkan ZigZag and modified it so that the Kijun ZigZag has its own integer, buffers and so on.
Once done, unfortunately, the ZigZag created by my code for the Kijunsen is wrong.
On this picture, you can see the Tenkan ZigZag following the change of direction of the Tenkansen properly:
On the next one, you can see the incorrect Kijun ZigZag with the Kijunsen on which the ZigZag is supposed to be based:
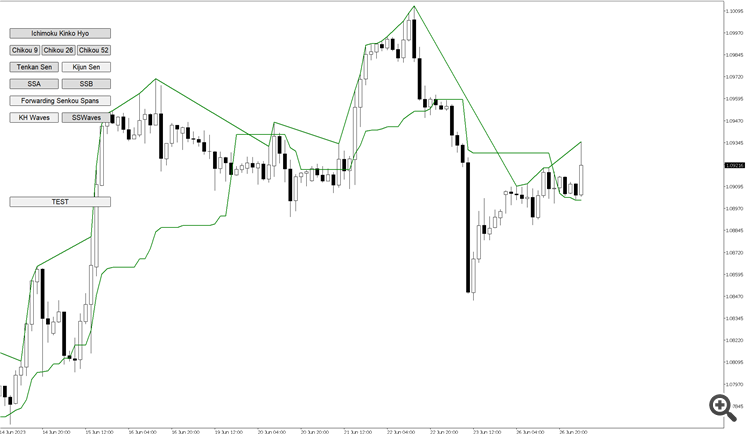
And finally, both ZigZag drawn on the chart:
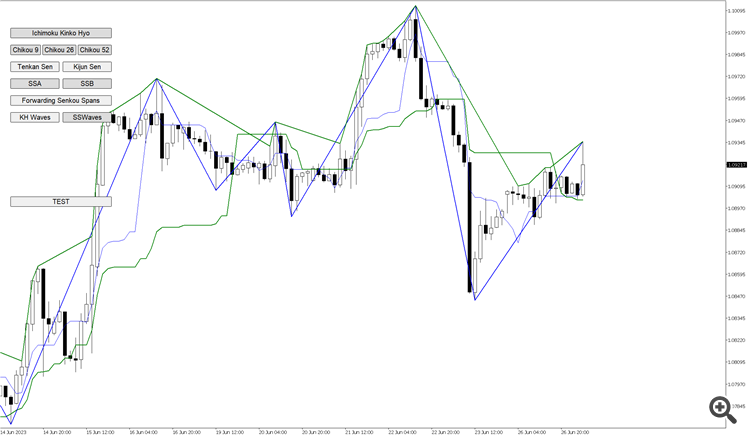
I played around with my code to try and find out where the issue lied but to no avail. So I came to you all for help. Here is the current code I'm using:
Once I manage to get the Kijun ZigZag working, the next step will be to do the same for the 3 other ZigZags, and add buttons on chart to show/hide each zigzag. However, for now, I'm stuck and cannot get that second ZigZag plotting to be populated correctly.
If you have any idea/hypotheses/solutions on this issue, I'll try them all!
Thanks in advance,
basic