- Tomáš Týbl: it is not automatically closing trades and i do not know why.
Use the debugger or print out your variables, including _LastError and prices and find out why. Do you really expect us to debug your code for you?
Code debugging - Developing programs - MetaEditor Help
Error Handling and Logging in MQL5 - MQL5 Articles (2015)
Tracing, Debugging and Structural Analysis of Source Code - MQL5 Articles (2011)
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator - MQL5 Articles (2010) -
ticket=OrderClose(OrderTicket(), OrderLots(), Bid, Slippage, Red); ⋮ ticket=OrderModify(OrderTicket(), OrderOpenPrice(), Ask-sl, OrderTakeProfit(),0,0);
OrderClose does not return a ticket, OrderModify does not return a ticket. Check your return codes, and report your errors (including market prices and your variables). Don't look at GLE/LE unless you have an error. Don't just silence the compiler (MT5/MT4+strict), it is trying to help you.
What are Function return values ? How do I use them ? - MQL4 programming forum (2012) -
magicNumber++; ticket=OrderSend(Symbol(),OP_SELL,Lots,Bid,Slippage,Bid+(Stop*myPoint),Bid-(Profit*myPoint),NULL,magicNumber,0,Red);
Magic number only allows an EA to identify its trades from all others. Using OrdersTotal/OrdersHistoryTotal (MT4) or PositionsTotal (MT5), directly and/or no Magic number/symbol filtering on your OrderSelect / Position select loop means your code is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 programming forum (2013)
PositionClose is not working - MQL5 programming forum (2020)
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles (2006)
Orders, Positions and Deals in MetaTrader 5 - MQL5 Articles (2011)
Limit one open buy/sell position at a time - General - MQL5 programming forum (2022)You need one Magic Number for each symbol/timeframe/strategy.
Trade current timeframe, one strategy, and filter by symbol requires one MN.
If trading multiple timeframes, and filter by symbol requires use a range of MN (base plus timeframe).
Why are MT5 ENUM_TIMEFRAMES strange? - General - MQL5 programming forum - Page 2 #11 (2020) -
You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit by the Ask.
-
Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid / OrderClosePrice reaches it. Using Ask±n, makes your SL shorter and your TP longer, by the spread. Don't you want the specified amount used in either direction?
-
Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask / OrderClosePrice reaches it. To trigger close at a specific Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 -
The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (control+O) → charts → Show ask line.)
Most brokers with variable spreads widen considerably at end of day (5 PM ET) ± 30 minutes.
My GBPJPY shows average spread = 26 points, average maximum spread = 134.
My EURCHF shows average spread = 18 points, average maximum spread = 106.
(your broker will be similar).
Is it reasonable to have such a huge spreads (20 PIP spreads) in EURCHF? - General - MQL5 programming forum (2022)
-
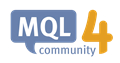

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have made my first EA, working with codes in forum but there is one small issue - it is not automatically closing trades and i do not know why. Can someone please explain to me, why it is opening trades correctly, but not ever closing trades?
I thought i was using magic number the right way, but it seems I am not. I will be glad for any advice or suggestion.
With regards, Thomas