adicahyanto:
hi guys, i want to create a matrix from a csv file,
i've read the documentation here :
https://www.mql5.com/en/docs/basis/types/matrix_vector
i try to use the FromFile method but when i try to compile, it came out error "FromFile" undeclared identifier.
could somebody explain me more please?
---------------------------
then i'm trying to import manually from csv to matrix as code below:
but the printed out of data and the printed out of matrix mat_ show different result. could you please pointing me out where did i miss.
thanks
found it.
what its need to be done is just by adding another finalcols, finalrows variables and then do matrix.Resize one again after the while loop.
int cols = 0, rows = 0, fcols = 0, frows = 0; string data; while(!FileIsEnding(fhandle)) { data = FileReadString(fhandle); if(rows == 0) { mtx.Resize(rows+1,cols+1); } mtx[rows,cols] = (double(data)); cols++; if(FileIsLineEnding(fhandle)) { fcols = cols; rows++; cols = 0; mtx.Resize(rows+1,fcols); } } frows = rows; mtx.Resize(frows,fcols);
Here is the simple function to do it https://www.mql5.com/en/articles/11858#matrix-from-csv
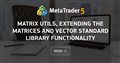
Matrix Utils, Extending the Matrices and Vector Standard Library Functionality
- www.mql5.com
Matrix serves as the foundation of machine learning algorithms and computers in general because of their ability to effectively handle large mathematical operations, The Standard library has everything one needs but let's see how we can extend it by introducing several functions in the utils file, that are not yet available in the library

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
hi guys, i want to create a matrix from a csv file,
i've read the documentation here :
https://www.mql5.com/en/docs/basis/types/matrix_vector
i try to use the FromFile method but when i try to compile, it came out error "FromFile" undeclared identifier.
could somebody explain me more please?
---------------------------
then i'm trying to import manually from csv to matrix as code below:
but the printed out of data and the printed out of matrix mat_ show different result. could you please pointing me out where did i miss.
thanks