It appears that these two lines are producing the exact same data
curtaHandle = iMA(_Symbol,_Period,periodoCurta,0,MODE_SMA,PRICE_CLOSE); longaHandle = iMA(_Symbol,_Period,periodoCurta,0,MODE_SMA,PRICE_CLOSE);
So, curtaHandle will always equal longaHandle.
To troubleshoot further you can comment out the most complicated lines in your code. Then add a Print Statement to what is left and see if the code is running. So comment out everything in your first IF statement , Add a print comment. then look in the journal to see if the code enters the print statement ( ie print the value of curtaHandle and longaHandle).
A further question is : Does curtaHandle and longaHandle even get to 3?
Hope this helps,
Chris
It appears that these two lines are producing the exact same data
So, curtaHandle will always equal longaHandle.
To troubleshoot further you can comment out the most complicated lines in your code. Then add a Print Statement to what is left and see if the code is running. So comment out everything in your first IF statement , Add a print comment. then look in the journal to see if the code enters the print statement ( ie print the value of curtaHandle and longaHandle).
A further question is : Does curtaHandle and longaHandle even get to 3?
Hope this helps,
Chris
Thank you for the reply, how would i print? New to MQL5.
Edit: printed the code by using: printf(curtaHandle,longaHandle); Commented out the entire IF statement, Where do i acess the print? Not seeing anything.
If you are new you maybe should read:
Quickstart for newbies: https://www.mql5.com/en/articles/496
and: https://www.mql5.com/en/articles/100
Beside that if your EAQ compiles without any error bus does not do what it should debug it:
https://www.metatrader5.com/en/metaeditor/help/development/debug
https://www.mql5.com/en/articles/654
Die Fehlerverarbeitung und Protokollierung in MQL5: https://www.mql5.com/en/articles/2041
https://www.mql5.com/en/articles/272
Fehler finden und Protokollierung https://www.mql5.com/en/articles/150
Can i get any Free Tutorial for Forex Trading? https://www.mql5.com/en/forum/381853#comment_25845157
My personal recommendation would be, look (search) for something that could be close to your idea and start from there by changing this according to your idea.
Bear in mind there's virtually nothing that hasn't already been programmed for MT4/MT5 and is ready even for you.
You can be much faster when you copy, paste, and modify than when you check out all the rookie mistakes. :)
In your case an EA of crossing MA: https://www.mql5.com/en/search#!keyword=crossing%20MA&module=mql5_module_articles
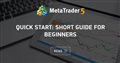
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
My trading bot doesn't buy or sell anything when i try to use the strategy calculator, other bot's do. Heres the code!