Hello everybody. I'm starting the study of creating "panels" in mql5. I made a simple label (code attached). How can I set the Background and Border color on the label? Thanks
Files:
Label_in_panel.mq5
14 kb
Tiofelo Da Olga Gilbert Teles:
Hello everybody. I'm starting the study of creating "panels" in mql5. I made a simple label (code attached). How can I set the Background and Border color on the label? Thanks
Hello everybody. I'm starting the study of creating "panels" in mql5. I made a simple label (code attached). How can I set the Background and Border color on the label? Thanks
//+------------------------------------------------------------------+ //| Label in Panel.mq5 | //| Copyright © 2023, Tiofelo Teles | //| "https://www.mql5.com/en/users/mozmakaveli"| //+------------------------------------------------------------------+ #property copyright "Copyright © 2023, Tiofelo Teles" #property link "https://www.mql5.com/en/users/mozmakaveli" #property version "1.00" #include <Controls\Dialog.mqh> #include <Controls\Label.mqh> //+------------------------------------------------------------------+ //| defines | //+------------------------------------------------------------------+ //--- indents and gaps #define INDENT_LEFT (11) // indent from left (with allowance for border width) #define INDENT_TOP (11) // indent from top (with allowance for border width) #define INDENT_RIGHT (11) // indent from right (with allowance for border width) #define INDENT_BOTTOM (11) // indent from bottom (with allowance for border width) #define CONTROLS_GAP_X (5) // gap by X coordinate #define CONTROLS_GAP_Y (5) // gap by Y coordinate //+------------------------------------------------------------------+ //| Class CControlsDialog | //| Usage: main dialog of the Controls application | //+------------------------------------------------------------------+ class CControlsDialog : public CAppDialog { private: CLabel m_label; // CLabel object public: CControlsDialog(void); ~CControlsDialog(void); //--- create virtual bool Create(const long chart,const string name,const int subwin,const int x1,const int y1,const int x2,const int y2); //--- chart event handler virtual bool OnEvent(const int id,const long &lparam,const double &dparam,const string &sparam); //--- Change text virtual void ChangeText(const string text) {m_label.Text(text);} protected: //--- create dependent controls bool CreateLabel(void); //--- handlers of the dependent controls events void OnClickLabel(void); }; //+------------------------------------------------------------------+ //| Event Handling | //+------------------------------------------------------------------+ EVENT_MAP_BEGIN(CControlsDialog) //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ EVENT_MAP_END(CAppDialog) //+------------------------------------------------------------------+ //| Constructor | //+------------------------------------------------------------------+ CControlsDialog::CControlsDialog(void) { } //+------------------------------------------------------------------+ //| Destructor | //+------------------------------------------------------------------+ CControlsDialog::~CControlsDialog(void) { } //+------------------------------------------------------------------+ //| Create | //+------------------------------------------------------------------+ bool CControlsDialog::Create(const long chart,const string name,const int subwin,const int x1,const int y1,const int x2,const int y2) { if(!CAppDialog::Create(chart,name,subwin,x1,y1,x2,y2)) return(false); //--- create dependent controls if(!CreateLabel()) return(false); //--- succeed return(true); } //+------------------------------------------------------------------+ //| Create the "CLabel" | //+------------------------------------------------------------------+ bool CControlsDialog::CreateLabel(void) { //--- coordinates int x1=INDENT_RIGHT; int y1=INDENT_TOP+CONTROLS_GAP_Y; int x2=x1+120; int y2=y1+30; //--- create if(!m_label.Create(m_chart_id,m_name+"Label",m_subwin,x1,y1,x2,y2)) return(false); if(!m_label.Text("Label1")) return(false); if(!Add(m_label)) return(false); if(!m_label.ColorBorder(clrWhite)) return(false); if(!m_label.ColorBackground(clrFireBrick)) return(false); //--- succeed return(true); } //+------------------------------------------------------------------+ //| Global Variables | //+------------------------------------------------------------------+ CControlsDialog ExtDialog; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- create application dialog if(!ExtDialog.Create(0," Label in Panel",0,40,40,380,344)) return(INIT_FAILED); //--- run application ExtDialog.Run(); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- destroy dialog ExtDialog.Destroy(reason); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- MqlTick STick; if(SymbolInfoTick(Symbol(),STick)) { string ask="The ask price:"; ExtDialog.ChangeText(ask+DoubleToString(STick.ask,Digits())); } } //+------------------------------------------------------------------+ //| Expert chart event function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, // event ID const long& lparam, // event parameter of the long type const double& dparam, // event parameter of the double type const string& sparam) // event parameter of the string type { ExtDialog.ChartEvent(id,lparam,dparam,sparam); } //+------------------------------------------------------------------+
pls use code ( Alt+S ) button to add .. like this
Tiofelo Da Olga Gilbert Teles:
Hello everybody. I'm starting the study of creating "panels" in mql5. I made a simple label (code attached). How can I set the Background and Border color on the label? Thanks
Hello everybody. I'm starting the study of creating "panels" in mql5. I made a simple label (code attached). How can I set the Background and Border color on the label? Thanks
There is almost nothing that hos not yet been programmed for MT4/5 - do you know that?
So here is a series of articles with code and examples about panel - just for you (and others) to past and copy what you need:
- DoEasy. Controls (Part 27): Working on ProgressBar WinForms object
- DoEasy. Controls (Part 26): Finalizing the ToolTip WinForms object and moving on to ProgressBar development
- DoEasy. Controls (Part 25): Tooltip WinForms object
- DoEasy. Controls (Part 24): Hint auxiliary WinForms object
- DoEasy. Controls (Part 23): Improving TabControl and SplitContainer WinForms objects
- DoEasy. Controls (Part 22): SplitContainer. Changing the properties of the created object
DoEasy. Controls (Part 14): New algorithm for naming graphical elements. Continuing work on the TabControl WinForms object
DoEasy. Controls (Part 15): TabControl WinForms object — several rows of tab headers, tab handling methods
DoEasy. Controls (Part 16): TabControl WinForms object — several rows of tab headers, stretching headers to fit the container
DoEasy. Controls (Part 17): Cropping invisible object parts, auxiliary arrow buttons WinForms objects
DoEasy. Controls (Part 18): Functionality for scrolling tabs in TabControl
DoEasy. Controls (Part 19): Scrolling tabs in TabControl, WinForms object events
DoEasy. Controls (Part 20): SplitContainer WinForms object
DoEasy. Controls (Part 21): SplitContainer control. Panel separator
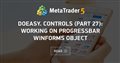
DoEasy. Controls (Part 27): Working on ProgressBar WinForms object
- www.mql5.com
In this article, I will continue the development of the ProgressBar control. In particular, I will create the functionality for managing the progress bar and visual effects.
Carl Schreiber #:
DoEasy. Controls (Part 14): New algorithm for naming graphical elements. Continuing work on the TabControl WinForms object
DoEasy. Controls (Part 15): TabControl WinForms object — several rows of tab headers, tab handling methods
DoEasy. Controls (Part 16): TabControl WinForms object — several rows of tab headers, stretching headers to fit the container
DoEasy. Controls (Part 17): Cropping invisible object parts, auxiliary arrow buttons WinForms objects
DoEasy. Controls (Part 18): Functionality for scrolling tabs in TabControl
DoEasy. Controls (Part 19): Scrolling tabs in TabControl, WinForms object events
DoEasy. Controls (Part 20): SplitContainer WinForms object
DoEasy. Controls (Part 21): SplitContainer control. Panel separator
There is almost nothing that hos not yet been programmed for MT4/5 - do you know that?
So here is a series of articles with code and examples about panel - just for you (and others) to past and copy what you need:
- DoEasy. Controls (Part 27): Working on ProgressBar WinForms object
- DoEasy. Controls (Part 26): Finalizing the ToolTip WinForms object and moving on to ProgressBar development
- DoEasy. Controls (Part 25): Tooltip WinForms object
- DoEasy. Controls (Part 24): Hint auxiliary WinForms object
- DoEasy. Controls (Part 23): Improving TabControl and SplitContainer WinForms objects
- DoEasy. Controls (Part 22): SplitContainer. Changing the properties of the created object
DoEasy. Controls (Part 14): New algorithm for naming graphical elements. Continuing work on the TabControl WinForms object
DoEasy. Controls (Part 15): TabControl WinForms object — several rows of tab headers, tab handling methods
DoEasy. Controls (Part 16): TabControl WinForms object — several rows of tab headers, stretching headers to fit the container
DoEasy. Controls (Part 17): Cropping invisible object parts, auxiliary arrow buttons WinForms objects
DoEasy. Controls (Part 18): Functionality for scrolling tabs in TabControl
DoEasy. Controls (Part 19): Scrolling tabs in TabControl, WinForms object events
DoEasy. Controls (Part 20): SplitContainer WinForms object
DoEasy. Controls (Part 21): SplitContainer control. Panel separator
Many thanks for the links provided.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register