First set the empty value (PLOT_EMPTY_VALUE) with PlotIndexSetDouble().
Then set in the arrow buffer all values older than ... on PLOT_EMPTY_VALUE.
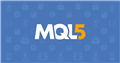
Documentation on MQL5: Custom Indicators / PlotIndexSetDouble
- www.mql5.com
PlotIndexSetDouble - Custom Indicators - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Im trying fracts buffor arrows to show only limited to 5 backwards and 5 frontwards of OBJ_'TEXT highest and lowest point
currently it shows fractal on every bar
Any help is highly appreciated
Here is code
Thanks